Most asked Singleton Design Pattern Interview Questions
Last Updated :
21 Dec, 2023
This article comprises some of the most asked Singleton design pattern interview questions, which will help you tackle any Singleton design pattern question properly.
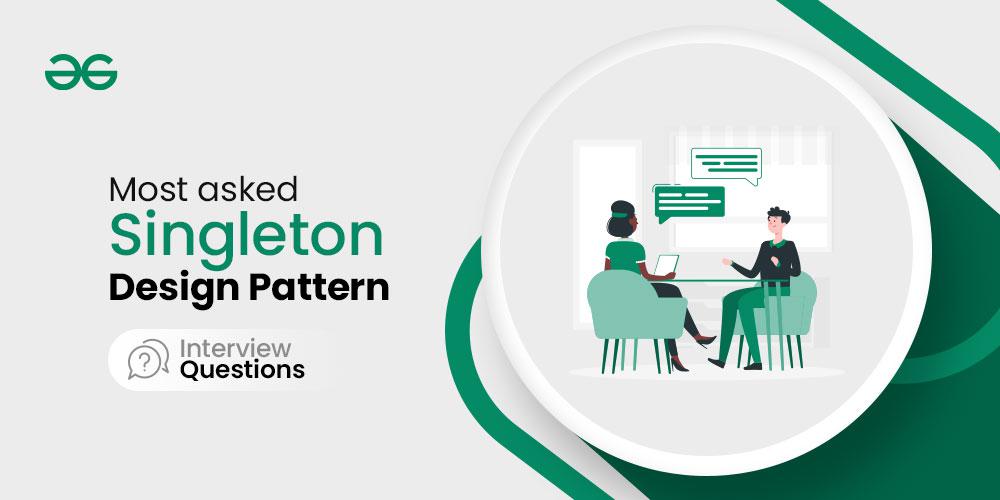
1. Give an example use case for the singleton design pattern.
This design pattern can be useful in various scenarios, such as when you need to control access to a shared resource, manage a single point of control, or maintain a single configuration object.
for example:
Use Case: Logger
Let’s say you are developing a large application, and you want to implement a logging system to keep track of important events, errors, and debugging information. You want to ensure that there is only one instance of the logger throughout your application to avoid redundant logging or conflicting logs.
2. How does the Singleton pattern ensure that only one instance of a class is available in the application?
The Singleton pattern ensures that only one instance of a class is available in an application by controlling the instantiation process. The key aspects of the Singleton pattern that contribute to this are:
- Private Constructor: The class with the Singleton pattern typically has a private constructor, preventing external code from directly creating instances of the class.
- Static Instance: The Singleton class maintains a static or class-level variable that holds the reference to the single instance. This variable is often named something like
_instance
and is initially set to None
.
- Creation Control in “
new"
or getInstance
method: The Singleton class overrides the “new"
method (or provides a separate method like getInstance
) to control the instantiation process. If the _instance
variable is None
, it creates a new instance; otherwise, it returns the existing instance.
With these elements in place, when a client code requests an instance of the Singleton class, it always receives the same instance. The first time an instance is requested, the _instance
is None
, so a new instance is created. For subsequent requests, the existing instance is returned, preventing the creation of multiple instances.
3. Mention potential security or privacy concerns when using the singleton design pattern and how you can mitigate them?
Here are some potential security and privacy concer, along with ways to mitigate them:
Global State
Since a Singleton provides a global point of access to its instance, it introduces global state into the application. This can lead to unintended interactions between different parts of the code, potentially causing security vulnerabilities or unexpected behavior.
Mitigation:
Be mindful of the global state and encapsulate it properly. Avoid excessive reliance on global variables within the Singleton instance. If possible, use dependency injection to provide necessary dependencies rather than relying on global access.
Thread Safety
In a multi-threaded environment, the Singleton pattern may lead to race conditions if not implemented carefully. If multiple threads attempt to create the Singleton instance simultaneously, it might result in the creation of multiple instances.
Mitigation:
Implement thread-safe creation of the Singleton instance. Techniques such as double-checked locking, using synchronization mechanisms like locks or mutexes, or using lazy initialization can help ensure thread safety.
Reflection and Serialization
Singleton instances might be susceptible to issues related to reflection and serialization, allowing an attacker to create additional instances or manipulate the state.
Mitigation:
Override serialization-related methods (e.g., __reduce__
in Python) to control how the object is serialized. Additionally, consider using techniques to prevent reflection-based attacks, such as marking the constructor as private or implementing additional checks during deserialization.
Testing Challenges
Unit testing can become challenging when a class relies heavily on global state, as it may introduce dependencies that are hard to control.
Mitigation:
Design classes to be more testable by favoring dependency injection over global state. Ensure that the Singleton class can be easily mocked or replaced with test doubles during testing.
Memory Leaks
If the Singleton instance holds onto resources that are not properly released, it can lead to memory leaks.
Mitigation:
Implement proper resource management and ensure that the Singleton instance releases resources when they are no longer needed. Use destructors or finalization methods if necessary.
- Guaranteed Single Instance:
- The primary purpose of the Singleton pattern is to ensure that a class has only one instance and provides a global point of access to that instance. This is particularly useful when exactly one object is needed to coordinate actions across the system.
- Global Point of Access:
- The Singleton provides a global point of access to the instance, making it easy for different parts of the codebase to interact with the same object. This can simplify communication and coordination within an application.
- Lazy Initialization:
- The Singleton instance can be created lazily, i.e., only when it is first requested. This can be beneficial in terms of resource efficiency, especially if the instantiation of the object is resource-intensive.
- Centralized Resource Management:
- The Singleton pattern is useful for managing and centralizing resources such as database connections, file handles, or network connections. It ensures that these resources are shared effectively and avoids unnecessary duplication.
- Reduction of Global Variables:
- Singleton can help reduce the reliance on global variables, promoting better encapsulation and organization of code.
- Consistency:
- Since there is only one instance of the Singleton class, it helps maintain a consistent state across the application. This can be important in scenarios where consistency is crucial.
- Ease of Implementation:
- Implementing the Singleton pattern is relatively simple, and it’s a well-known design pattern. Many programming languages provide mechanisms (like static variables or class-level variables) that facilitate its implementation.
- Global State:
- Issue: The Singleton pattern introduces a global point of access, leading to a global state. This global state can make the system less modular and harder to understand and maintain.
- Impact: Increased interdependence between different parts of the code can result in unintended side effects and make it challenging to reason about the program’s behavior.
- Testing Challenges:
- Issue: Testing a class that relies on a Singleton can be difficult. The global state of the Singleton might affect the behavior of the unit tests, making it harder to isolate and test individual components.
- Impact: Unit tests should ideally be independent and predictable, but the use of a Singleton can introduce dependencies that are hard to control during testing.
- Limited Extensibility:
- Issue: The Singleton pattern does not readily support subclassing or extension. If you want to change the behavior of the Singleton class, you might face challenges due to its rigid structure.
- Impact: This limitation can hinder the flexibility and extensibility of the codebase, making it harder to adapt to changing requirements.
- Violates Single Responsibility Principle:
- Issue: The Singleton class is responsible for both managing its instance and providing the global point of access. This violates the Single Responsibility Principle, which states that a class should have only one reason to change.
- Impact: Violating this principle can lead to code that is less maintainable and more prone to bugs as changes in one aspect of the class may inadvertently affect the other.
- Concurrency Issues:
- Issue: In a multithreaded environment, creating a Singleton instance might lead to race conditions if not implemented carefully. Multiple threads might attempt to create an instance simultaneously, leading to the creation of multiple instances.
- Impact: Concurrency issues can result in unpredictable behavior and compromise the integrity of the Singleton pattern. Implementing thread safety adds complexity to the code.
6. How to handle the multithreaded environments when you are implementing the singleton design pattern?
Several techniques can be employed to ensure thread safety in a multithreaded environment such as:
- Double-Checked Locking (DCL):
- Use a double-checked locking mechanism to minimize the overhead of synchronization while still ensuring thread safety.
- Module-Level Initialization:
- Leverage the fact that module-level initialization in Python is atomic to ensure that the Singleton instance is created only once, even in a multithreaded environment.
- Use of
@synchronized
Decorator:
- Utilize a custom synchronization decorator to ensure that only one thread can execute the method at a time.
The typical approach is to employ synchronization mechanisms, guaranteeing that only a single thread can access the method concurrently and ensuring the creation of just one instance of the singleton class. Another option is to implement the double-checked locking pattern, aiming to mitigate the performance overhead associated with synchronization. However, this alternative introduces added complexity and might still be susceptible to race conditions.
7. lternative design patterns that can be used to achieve the same goals as the Singleton Pattern?
- Dependency Injection:
- Goal: Provide a single instance of a class without enforcing a global point of access.
- Description: Instead of relying on a Singleton pattern, inject the instance of the class where it is needed, making dependencies explicit and allowing for better testability and flexibility.
- Monostate Pattern:
- Goal: Share state across instances without enforcing a single instance.
- Description: In the Monostate pattern, all instances share the same internal state. Each instance has its own methods, but they operate on a shared set of data. This is different from Singleton, as you can create multiple instances with shared state.
- Registry Pattern:
- Goal: Centralized management of instances without enforcing a single instance.
- Description: A registry or container holds references to instances, and other parts of the application can retrieve instances from the registry. This allows for centralized management of shared resources without enforcing a single instance.
- Factory Method Pattern:
- Goal: Provide a method for creating instances of a class without specifying the exact class.
- Description: The Factory Method Pattern involves defining an interface for creating an object, but letting subclasses alter the type of objects that will be created. It allows for flexibility in creating instances without enforcing a single instance.
- Lazy Initialization Pattern:
- Goal: Delay the creation of an instance until it is needed.
- Description: Instances are created only when they are first requested. This pattern doesn’t enforce a single instance globally but allows for efficient resource utilization.
8. How to test a class designed with the Singleton Pattern?
To assess a class implemented with the Singleton Pattern, it is essential to first confirm its correct implementation. One method to validate the class involves creating multiple instances of it and subsequently comparing the memory addresses of each instance. This comparison helps ascertain whether they reference the same object, thereby confirming the proper utilization of the design pattern.
After confirming the class’s correctness, another approach to assess its functionality is to develop unit tests for each of its methods. These tests should encompass various scenarios, including positive, negative, and boundary cases that the method must handle. For instance, a unit test could be crafted to verify that a Singleton class appropriately returns an existing instance when a new instance is requested.
9. Differentiate between an eager and a lazy Singleton?
The difference between an eager and a lazy Singleton lies in the timing of the instantiation of the Singleton instance:
Eager Singleton:
- In an eager Singleton, the instance of the class is created at the time the class is loaded or the application starts, regardless of whether it will be used immediately or not.
- The instance is created eagerly, and it is readily available for use whenever needed.
- Eager instantiation can help avoid potential concurrency issues in multithreaded environments since the instance is created before any threads start accessing it.
Lazy Singleton:
- In a lazy Singleton, the instance of the class is created only when it is first requested or when it is needed during the program’s execution.
- Lazy instantiation is a form of optimization, as it defers the creation of the instance until it is actually needed, potentially saving resources.
- Lazy instantiation might introduce concurrency concerns, especially in a multithreaded environment, as multiple threads could potentially request the instance simultaneously. To address this, developers often use synchronization mechanisms like locks.
10. Describe how the Singleton Pattern can help reduce resource consumption.
The Singleton Pattern can help reduce resource consumption through the implementation of a single, shared instance of a class, rather than creating multiple instances. Here are several ways in which the Singleton Pattern contributes to resource efficiency:
- Memory Efficiency:
- By ensuring that only one instance of the class exists throughout the program’s lifecycle, the Singleton Pattern prevents unnecessary duplication of objects in memory.
- Lazy Initialization:
- When the Singleton Pattern is implemented with lazy initialization, the instance is created only when it is first requested. This defers the allocation of resources until the instance is actually needed.
- It is especially useful when the Singleton instance is not required for the entire duration of the program, reducing the program’s initial memory footprint.
- Global Access to Resources:
- The Singleton Pattern provides a global point of access to its instance. This means that resources, such as database connections or configuration settings, can be managed centrally within the Singleton instance.
- Prevention of Unnecessary Object Creation:
- In scenarios where creating multiple instances of a class is not only unnecessary but also wasteful, the Singleton Pattern ensures that only one instance is ever created.
- Consistent State:
- The Singleton Pattern helps maintain a consistent state across the entire application by providing a single point of control for the instance. This can lead to more predictable resource usage and prevent conflicts arising from multiple instances with potentially conflicting states.
It’s important to note that while the Singleton Pattern can contribute to resource efficiency, its implementation should be approached carefully.
Share your thoughts in the comments
Please Login to comment...