JQuery Multiple ID selectors
Last Updated :
11 Jul, 2023
Given an HTML document and the task is to select the elements with different ID’s at the same time using JQuery.
Approach:
- Select the ID’s of different element and then use each() method to apply the CSS property on all selected ID’s element.
- Then use css() method to set the background color to pink to all selected elements.
- Display the text which indicates the multiple ID selectors.
Example 1: In this example, the elements of different ID’s are selected and the isbackground color of these elements are changed.
html
<!DOCTYPE HTML>
< html >
< head >
< title >
JQuery Multiple ID selectors
</ title >
< style >
#GFG_DIV {
background: green;
height: 100px;
width: 200px;
margin: 0 auto;
color: white;
}
</ style >
< script src =
</ script >
</ head >
< body style = "text-align:center;" >
< h1 style = "color:green;" >
GeeksForGeeks
</ h1 >
< p id = "GFG_UP" style="font-size: 19px;
font-weight: bold;">
</ p >
< div id = "GFG_DIV" >
This is Div box.
</ div >
< br >
< button onClick = "GFG_Fun()" >
click here
</ button >
< p id = "GFG_DOWN" style="color: green;
font-size: 24px;
font-weight: bold;">
</ p >
< script >
$('#GFG_UP').text(
"Click on button to select multiple"
+ " ID's and change their background-color");
function GFG_Fun() {
$("#GFG_UP, #GFG_DIV, #GFG_DOWN").each(
function () {
$(this).css("background-color", "pink");
});
$('#GFG_DOWN').text("Background-color of all "
+ "elements is changed.");
}
</ script >
</ body >
</ html >
|
Output:
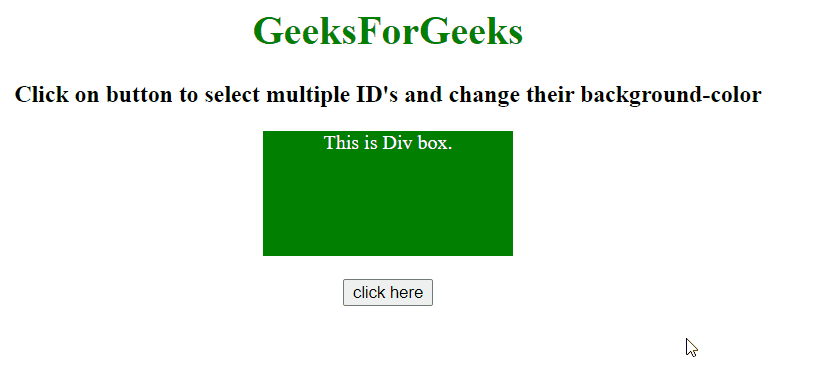
Example 2: In this example, the elements of different ID’s are selected and the text color of these elements is changed.
html
<!DOCTYPE HTML>
< html >
< head >
< title >
JQuery | Multiple ID selectors
</ title >
< style >
#GFG_DIV {
background: green;
height: 100px;
width: 200px;
margin: 0 auto;
color: white;
}
</ style >
< script src =
</ script >
</ head >
< body style = "text-align:center;" >
< h1 style = "color:green;" >
GeeksForGeeks
</ h1 >
< p id = "GFG_UP" style="font-size: 19px;
font-weight: bold;">
</ p >
< div id = "GFG_DIV" >
This is Div box.
</ div >
< br >
< button onClick = "GFG_Fun()" >
click here
</ button >
< p id = "GFG_DOWN" style="color: green;
font-size: 24px; font-weight: bold;">
</ p >
< script >
$('#GFG_UP').text(
"Click on button to select multiple"
+ "ID's and change their Text color");
function GFG_Fun() {
$("#GFG_UP, #GFG_DIV, #GFG_DOWN").each(function () {
$(this).css("color", "blue");
});
$('#GFG_DOWN').text("Text color of all elements is "
+ "changed.");
}
</ script >
</ body >
</ html >
|
Output:
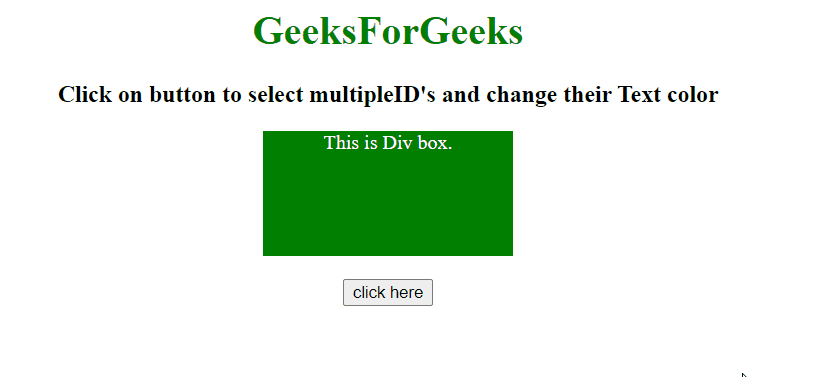
jQuery is an open source JavaScript library that simplifies the interactions between an HTML/CSS document, It is widely famous with it’s philosophy of “Write less, do more”. You can learn jQuery from the ground up by following this jQuery Tutorial and jQuery Examples.
Share your thoughts in the comments
Please Login to comment...