LinkedList add() Method in Java With Examples
Last Updated :
15 Nov, 2021
LinkedList is a class implementation of the LinkedList data structure which is a linear data structure where the elements are not stored in contiguous locations and every element is a separate object with a data part and address part. As we all know that class contains various methods so do here we will be discussing and implementing add() method to grasp a better understanding of how elements are added in a LinkedList.
For that refer to the below flowchart to get a better understanding of any methods. Note that it is very important to go through flowcharts in programming . Here two cases arises that is default addition of elements and custom addition of elements. Here we will be covering both of them as follows:
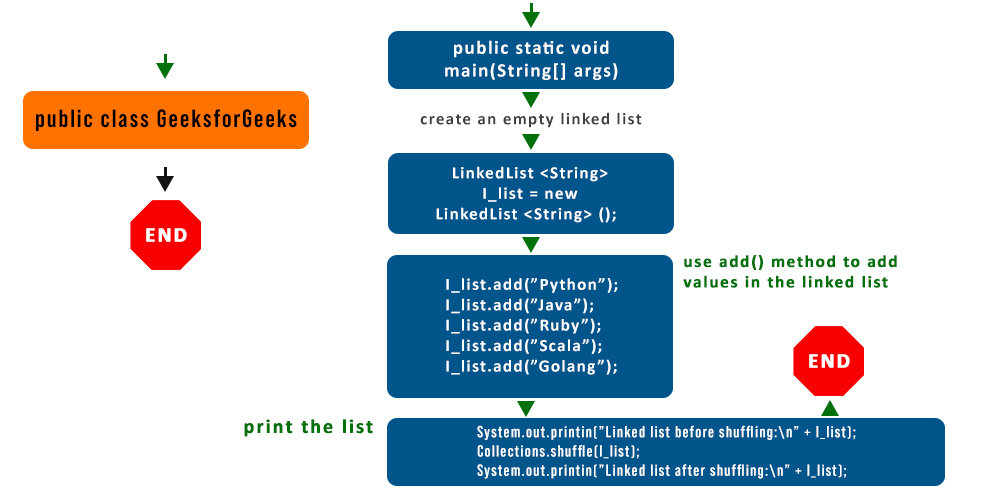
Case 1: Default addition by adding at last of List
This method appends the specified element to the end of this list. This function accepts a single parameter element as shown in the above syntax
Syntax:
boolean add(Object element)
Parameters: The element specified by this parameter is appended to the end of the list.
Return Value: Boolean true after execution.
Example:
Java
import java.io.*;
import java.util.LinkedList;
public class GFG {
public static void main(String args[])
{
LinkedList list = new LinkedList();
list.add( "Geeks" );
list.add( "for" );
list.add( "Geeks" );
list.add( "10" );
list.add( "20" );
System.out.println( "The list is:" + list);
list.add( "Last" );
list.add( "Element" );
System.out.println( "The new List is:" + list);
}
}
|
Output:
The list is:[Geeks, for, Geeks, 10, 20]
The new List is:[Geeks, for, Geeks, 10, 20, Last, Element]
Case 2: Adding at the specified index
This method inserts an element at a specified index in the list. It shifts the element currently at that position (if any) and any subsequent elements to the right (will add one to their indices).
Syntax:
void add(int index, Object element)
Parameters: This method accepts two parameters as described below.
- index: The index at which the specified element is to be inserted.
- element: The element which is needed to be inserted.
Return Value: Boolean true after execution.
Example:
Java
import java.util.LinkedList;
import java.io.*;
class GFG {
public static void main(String[] args) {
LinkedList<String> ll = new LinkedList();
ll.add( "Geeks" );
ll.add( "For" );
ll.add( "Geeks" );
System.out.println(ll);
ll.add( 2 , "Java" );
System.out.println(ll);
}
}
|
Output:
[Geeks, For, Geeks]
[Geeks, For, Java, Geeks]
Share your thoughts in the comments
Please Login to comment...