Java Program to Add Tables to a Word Document
Last Updated :
16 Nov, 2022
OpenCV (Open Source Computer Vision Library) is an open-source computer vision and machine learning software library. The library has more than 2500 optimized algorithms, which includes a comprehensive set of both classic and state-of-the-art computer vision. It has C++, Python, Java, and MATLAB interfaces and supports Windows, Linux, Android, and Mac OS.
OpenCV provides us various classes and interfaces to add/create a document in word. In, this article, we will be discussing all these classes as well as their implementation and the steps that how can we add word document using Java.
Accessing word file using java
A Jar file is an Zip archive containing one or multiple java class files. This makes the usage of libraries (consisting of multiple classes) more handy. Directories and Jar files are added to the classpath and available to the ClassLoader at runtime to find particular classes inside of it. We generally use .jar files to distribute Java applications or libraries, in the form of Java class files and associated metadata and resources (text, images, etc.). you can say JAR = Java ARchive.
We can read or access a java file using apache poi
Step 1: Download Apache POI API. You can download it from here
Step 2: Import .jar files. Extract jar files for your project, and add the following jar to your build path:
- poi
- poi-ooxml
- poi-ooxml-schemas
- poi-scratchpad
- ooxml-lib–>xmlbeans
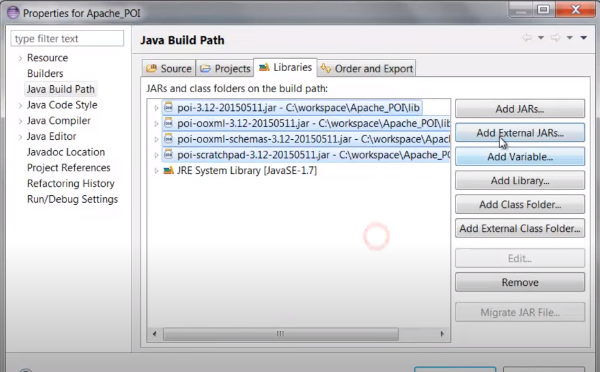
Step 3: Create a new java file to write the code in it and add it to your project
Java classes for adding a table
XWPFDocument
- org.apache.poi.xwpf.usermodel.XWPFDocument
- used to create a document
XWPFTable
- org.apache.poi.xwpf.usermodel.XWPFTable
- used to create a simple table
XWPFTableRow
- org.apache.poi.xwpf.usermodel.XWPFTableRow
- used to add row to the table
Procedure: Below are the steps to implement the classes to add a document using Java
Step 1: Import classes like XWPFDocument, XWPFTable, XWPFTableRow of io.File package which helps in creating a document, adding the table to the document, and then adding rows to it.
Step 2: Create a document using class XWPFDocument and store it in a variable
Step 3: Create table using class XWPFTable
Step 4: Add rows
Add rows using class XWPFTableRow. We can add as much row as we want to and every time before creating a new row, we have to call the class XWPFTableRow
Implementation:
Sample input Image: In which rows are to be inserted
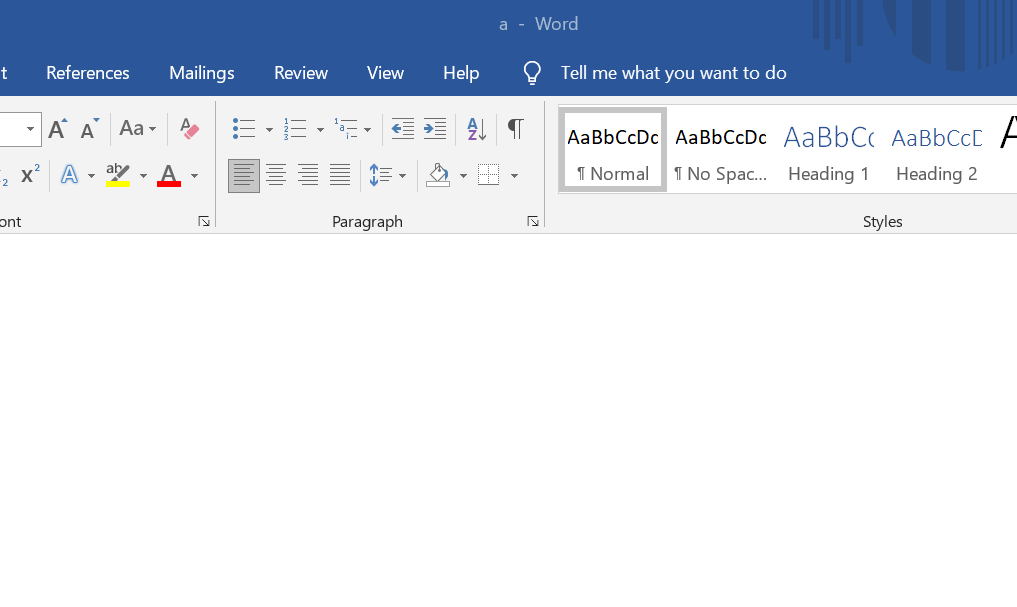
Example:
Java
import java.io.File;
import java.io.FileOutputStream;
import org.apache.poi.xwpf.usermodel.XWPFDocument;
import org.apache.poi.xwpf.usermodel.XWPFTable;
import org.apache.poi.xwpf.usermodel.XWPFTableRow;
public class GFG {
public static void main(String[] args) throws Exception
{
XWPFDocument document = new XWPFDocument();
FileOutputStream out = new FileOutputStream(
new File( "create_table.docx" ));
XWPFTable table = document.createTable();
XWPFTableRow tableRowOne = table.getRow( 0 );
tableRowOne.getCell( 0 ).setText( "Geeks (0,0)" );
tableRowOne.addNewTableCell().setText( "For (0,1)" );
tableRowOne.addNewTableCell().setText(
"Geeks (0,2)" );
XWPFTableRow tableRowTwo = table.createRow();
tableRowTwo.getCell( 0 ).setText( "Geeks (1,0)" );
tableRowTwo.getCell( 1 ).setText( "For (1,1)" );
tableRowTwo.getCell( 2 ).setText( "Geeks (1,2)" );
XWPFTableRow tableRowThree = table.createRow();
tableRowThree.getCell( 0 ).setText( "Geeks (2,0)" );
tableRowThree.getCell( 1 ).setText( "For (2,1)" );
tableRowThree.getCell( 2 ).setText( "Geeks (2,2)" );
document.write(out);
out.close();
System.out.println(
"create_table.docx written successfully" );
}
}
|
Output: Image adding up rows in the input
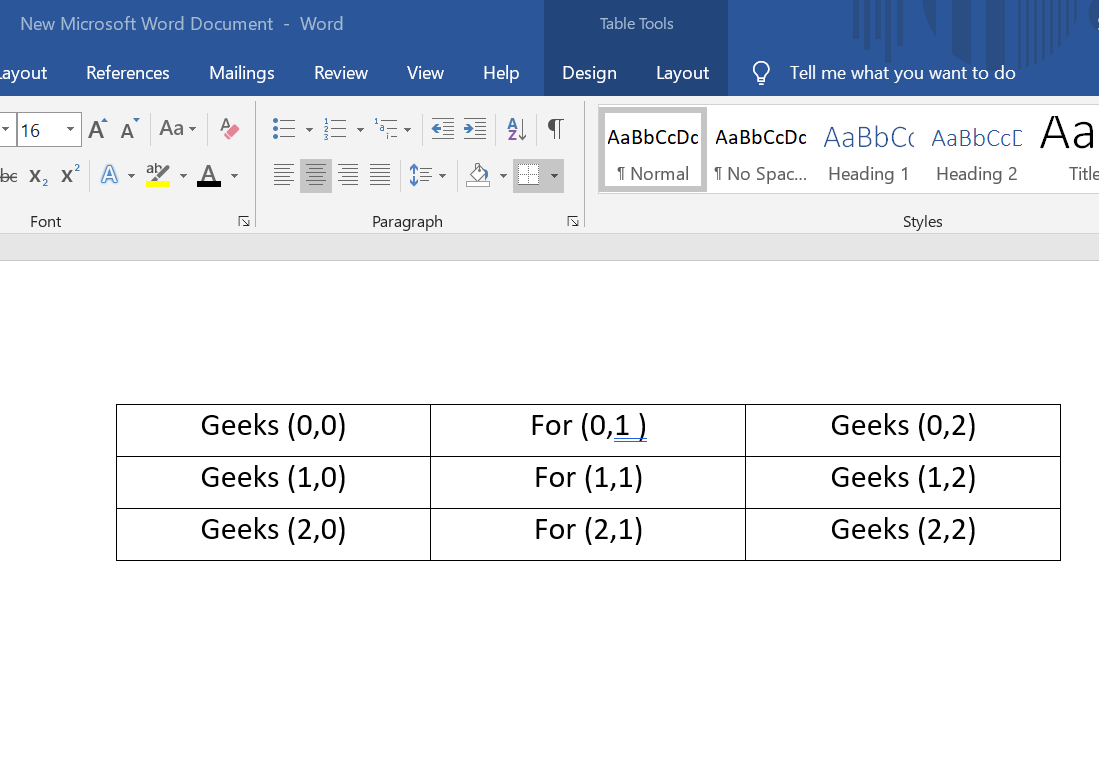
Share your thoughts in the comments
Please Login to comment...