Java JViewport
Last Updated :
26 Nov, 2023
Swing is a Java GUI (Graphical User Interface) toolkit that includes many components and tools for developing dynamic and user-friendly desktop applications. JViewPoint is majorly used for arranging and displaying large or scrollable content.
What is a JViewPort?
JViewport is mostly used to give a window into a larger view when the content exceeds the available screen area.
The fundamental scrolling model is defined by the JViewport class, which is made to support both logical and pixel-based scrolling. It is widely used within JScrollPane, a container that allows you to add scrollbars to a GUI component when the viewable area is exceeded.
Syntax of JViewPort
public class JViewport extends JComponent implements Accessible
The JViewPort class inherits the properties and attributes of the JComponent class, making it a graphical component for Java Swing applications. It also implements the Accessible interface which improves the accessibility for the users by offering appropriate interactive features.
Constructor of JViewPort
The table provides an overview of all the available constructors present for JViewport() along with their descriptions.
Constructs an instance of JViewPort. |
Nested Classes of JViewPort
The table provides an overview of all the available Classes present for JViewport() along with their description.
JViewport.AccessibleJViewport |
This class adds an accessibility functionality to the JViewport class. |
JViewport.ViewListener |
Adds a listener to the ViewPort. |
The nested classes offered by Java JViewPort are inherited from:
- javax.swing.JComponent
- java.awt.Container
- java.awt.Component
Fields of JViewPort
The table provides an overview of all the available Fields present for JViewport() along with their description.
BACKINGSTORE_SCROLL_MODE |
Creates a viewport image that is offscreen. |
backingStoreImage |
Basically, it is used to create a backup store |
BLIT_SCROLL_MODE |
Utilize graphics.copyArea where scrolling will be used. |
isViewSizeSet |
Returs True after determining the viewport’s dimensions. |
lastPaintPosition |
The last viewPosition that is painted, so we know how much of the backing store image is valid. |
scrollUnderway |
ScrollUnderway is used by components such as JList. |
SIMPLE_SCROLL_MODE |
Used to re-draw content of Scrollpane for each Scroll |
The fields of Java JViewPort are inherited from:
- java.awt.Component
- java.awt.image.ImageObserver
- javax.swing.JComponent
Methods of JViewPort
Following are the methods associated with the JViewPort class along with their detailed explanation:
void addChangeListener​(ChangeListener l) |
Adds a ChangeListener to detect any change in viewport’s extent size, position, or size, all at once to modify it accordingly. |
protected void addImpl​(Component child, Object constraints, int index) |
JViewport’s single lightweight child is set possibly that is null. |
protected JViewport.ViewListener createViewListener​() |
Creates a listener for the view. |
protected void firePropertyChange​(String propertyName, Object oldValue, Object newValue) |
Alerts listeners of a change in property. |
AccessibleContext getAccessibleContext​() |
Returns the AccessibleContext linked to the JViewport instance. |
ChangeListener[] getChangeListeners​() |
Returns back an array containing every ChangeListener that addChangeListener() adds to this JViewport. |
Dimension getExtentSize​() |
In view coordinates, it returns the size of the visible portion of the view. |
Insets getInsets​() |
Since borders are not allowed on a JViewport, the insets (border) dimensions are returned as (0,0,0,0). |
Insets getInsets​(Insets insets) |
Returns an Insets object with the values from the JViewports inset. |
int getScrollMode​() |
Gets the current scrolling mode. |
ViewportUI getUI​() |
L&F object is responsible for rendering Returnsstartsthe element. |
Component getView​() |
Returns null or the only child of the JViewport. |
Point getViewPosition​() |
Returns 0,0 in the event that there is no view, or the view coordinates that are shown in the viewport’s top left corner. |
Rectangle getViewRect​() |
Returns a rectangle with the size getExtentSize() and the origin getViewPosition(). |
Dimension getViewSize​() |
Return the desired size if the view’s size hasn’t been explicitly specified, and return the view’s current size otherwise. |
protected boolean isPaintingOrigin​() |
Returns true so that the painting start from JViewport or one of its predecessors if the scroll mode is BACKINGSTORE_SCROLL_MODE. |
void remove​(Component child) |
Eliminates the single lightweight child’s viewport. |
void removeChangeListener​(ChangeListener l) |
will delete the ChangeListener if it receives any notifications for a change to the viewport’s extent size, position, or size. |
void reshape​(int x, int y, int w, int h) |
Defines this viewport’s boundaries. |
void scrollRectToVisible​(Rectangle contentRect) |
Makes the rectangle inside the view visible by scrolling the view. |
void setExtentSize​(Dimension newExtent) |
Uses the view coordinates to set the size of the visible portion of the view. |
void setScrollMode​(int mode) |
Maintains the content of the viewport being scrolled. |
void setUI​(ViewportUI ui) |
This object specifies the L&F that renders this component. |
void setView​(Component view) |
Sets the single lightweight child (view) of the JViewport (can be null also). |
void setViewPosition​(Point p) |
Sets the view coordinates that show up in the viewport’s top left corner. If there is no view, nothing happens. |
void setViewSize​(Dimension newSize) |
Sets the size of the view. |
void updateUI​() |
The UI attribute is reset to a value from the current look and feel. |
The methods corresponding to Java JViewPort are inherited from packages like:
- java.awt.Container
- java.awt.Component
- javax.swing.JComponent
- java.lang.Object
Example of Java JViewport
In the given example, the Swing application created by this Java code demonstrates the use of JViewport. It creates a JFrame with a JViewport that has two labels: one labeled “A computer science portal for geeks!” underneath it, and a big one labeled “GeeksforGeeks” centered as the view. This code offers a basic example of how to create a scrollable view inside of a GUI by demonstrating how to define various parameters like font, color, and scroll mode respectively.
Java
import java.awt.*;
import javax.swing.*;
public class Main {
public static void main(String[] args)
{
JFrame f = new JFrame( "JViewport Example" );
f.setLayout( new BorderLayout());
JLabel l1 = new JLabel( "GeeksforGeeks" );
l1.setFont( new Font( "Arial" , Font.BOLD, 40 ));
l1.setForeground(Color.GREEN);
l1.setHorizontalAlignment(JLabel.CENTER);
JLabel l2 = new JLabel(
"A computer science portal for geeks!" );
l2.setFont( new Font( "Arial" , Font.PLAIN, 14 ));
JViewport j = new JViewport();
j.setView(l1);
j.setViewPosition( new Point( 50 , 25 ));
j.setPreferredSize( new Dimension( 350 , 200 ));
j.setScrollMode(JViewport.SIMPLE_SCROLL_MODE);
JPanel p = new JPanel();
p.add(j);
p.add(l2);
f.add(p, BorderLayout.CENTER);
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
f.setSize( 400 , 400 );
f.setVisible( true );
}
}
|
You can execute the code by running the following commands:
Javac Main.java
Java Main
Output:
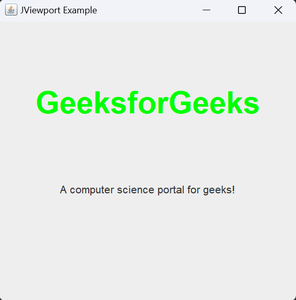
Share your thoughts in the comments
Please Login to comment...