Java JScrollPane
Last Updated :
13 Nov, 2023
Java JScrollPane is a component in the Java Swing library that provides a scrollable view of another component, usually a JPanel or a JTextArea. it provides a scrolling functionality to the display for which the size changes dynamically. It is useful to display the content which exceeds the visible area of the window. In this article, we are going to see some constructors, methods, and examples of JScrollPane.
Constructor of JScrollPane
JScrollPane()
|
It is a default constructor that creates an empty JScrollPane.
|
JScrollPane(Component comp)
|
This constructor creates a JScrollPane with the specified view component as the scrollable content.
|
JScrollPane(int vertical, int horizontal)
|
This constructor creates an empty JScrollPane with the specified vertical and horizontal scrollbar.
|
JScrollPane(LayoutManager layout)
|
This constructor creates a JScrollPane with the specified layout manager.
|
Methods of JScrollPane
void setVerticalScrollBarPolicy(int vertical)
|
Sets the vertical scrollbar policy
|
void setHorizontalScrollBarPolicy(int horizontal)
|
Sets the horizontal scrollbar policy
|
void setColumnHeaderView(Component comp)
|
sets the column header for the JScrollPane
|
void setRowHeaderView(Component comp)
|
sets the rowheader for the JScrollPane
|
setCorner(String key, Component corner)
|
It is used to set a component to be displayed in one of the corners of the scroll pane
|
Component getCorner(String key)
|
It is used to retrieve the component that has been previously set in one of the corners of the scroll pane using the setCorner method
|
void setViewportView(Component comp)
|
It is used to set the component that will be displayed in the viewport of the scroll pane
|
Following are the programs to implement JScrollPane
1. Java Program to demonstrate simple JscrollPane
Below is the implementation of the above topic:
Java
import javax.swing.*;
import java.awt.*;
public class SimpleJScrollPaneExample {
public static void main(String[] args) {
JFrame frame = new JFrame( "Simple JScrollPane Example" );
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize( 300 , 200 );
JPanel panel = new JPanel();
panel.setLayout( new BoxLayout(panel, BoxLayout.Y_AXIS));
for ( int i = 1 ; i <= 50 ; i++) {
JLabel label = new JLabel( "Label " + i);
panel.add(label);
}
JScrollPane scrollPane = new JScrollPane(panel);
frame.add(scrollPane);
frame.setVisible( true );
}
}
|
Output:
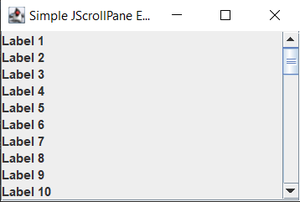
2. Java Program to Implementing a JTextArea to JScrollPane
Below is the implementation of the above topic:
Java
import javax.swing.*;
public class JTextAreaExample {
public static void main(String[] args)
{
JFrame frame = new JFrame( "Java JTextArea in JScrollPane" );
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize( 400 , 300 );
JTextArea textArea = new JTextArea( 10 , 30 );
JScrollPane scrollPane = new JScrollPane(textArea);
frame.add(scrollPane);
frame.setVisible( true );
}
}
|
Output:
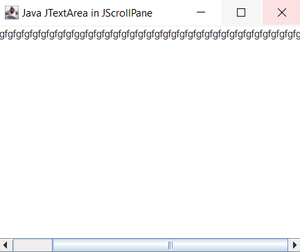
Share your thoughts in the comments
Please Login to comment...