Java JScrollBar
Last Updated :
13 Nov, 2023
Java’s Swing library provides various GUI components for creating graphical user interfaces. Among these components, is the JScrollBar class, which allows users to scroll through the content of a component, such as a JScrollPane. In Java JScrollBar is a part of javax.swing package.
Constructor of JScrollBar
1. This is the default constructor that creates a horizontal scrollbar with the default values. Its default orientation is JScrollBar (HORIZONTAL).
JScrollBar()
2. This constructor creates a scrollbar with the specified orientation. The orientation parameter can be one of the following constants: JScrollBar.HORIZONTAL, JScrollBar.VERTICAL
JScrollBar(int orientation)
3. This constructor creates a scrollbar with specified orientation, value, extent, minimum and maximum.
JScrollBar(int orientation, int value, int extent, int min, int max)
Some Methods of the JScrollBar
Methods of the JScrollBar are mentioned below:
- setValue(int value) : Sets the current value of the scrollbar to a specified value.
- getValue() : Retrieves the current value of the scrollbar, which indicates the current position of the thumb.
- setMinimum(int minimum) : Sets the minimum value of the scrollbar, defining the lower boundary of the range.
- getMinimum() : Returns the minimum value, allowing you to retrieve the lower boundary of the range.
- setMaximum(int maximum) : Sets the maximum value of the scrollbar, defining the upper boundary of the range.
- getMaximum() : Retrieves the maximum value, indicating the upper boundary of the range.
Following are the programs to implement JScrollBar
1. Java Program to Implement a Vertical ScrollBar
Java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class VerticalScrollBarDemo {
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> {
JFrame frame = new JFrame( "Vertical ScrollBar Demo" );
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize( 400 , 300 );
JPanel contentPanel = new JPanel();
contentPanel.setLayout( new BorderLayout());
JTextArea textArea = new JTextArea( 10 , 40 );
textArea.setWrapStyleWord( true );
textArea.setLineWrap( true );
JScrollPane scrollPane = new JScrollPane(textArea, JScrollPane.VERTICAL_SCROLLBAR_ALWAYS, JScrollPane.HORIZONTAL_SCROLLBAR_NEVER);
JScrollBar verticalScrollBar = scrollPane.getVerticalScrollBar();
JButton addButton = new JButton( "Add Text" );
addButton.addActionListener( new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
textArea.append( "JScrollBar Example.\n" );
}
});
contentPanel.add(scrollPane, BorderLayout.CENTER);
contentPanel.add(addButton, BorderLayout.SOUTH);
frame.add(contentPanel);
frame.setVisible( true );
});
}
}
|
Output :
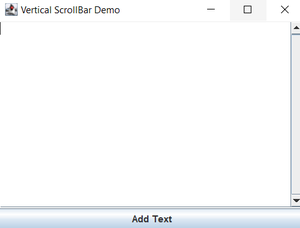
Another Screenshot with Printed Data

2. Java Program to Implement a Horizontal ScrollBar
Below is the implementation of the above mentioned:
Java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class HorizontalScrollBarDemo {
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> {
JFrame frame = new JFrame( "Horizontal ScrollBar Demo" );
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize( 400 , 300 );
JPanel contentPanel = new JPanel();
contentPanel.setLayout( new BorderLayout());
JTextArea textArea = new JTextArea( 10 , 40 );
textArea.setWrapStyleWord( true );
textArea.setLineWrap( false );
JScrollPane scrollPane = new JScrollPane(textArea, JScrollPane.VERTICAL_SCROLLBAR_NEVER, JScrollPane.HORIZONTAL_SCROLLBAR_ALWAYS);
JScrollBar horizontalScrollBar = scrollPane.getHorizontalScrollBar();
JButton addButton = new JButton( "Add Text" );
addButton.addActionListener( new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
textArea.append( "JScrollBar Example. " );
}
});
contentPanel.add(scrollPane, BorderLayout.CENTER);
contentPanel.add(addButton, BorderLayout.SOUTH);
frame.add(contentPanel);
frame.setVisible( true );
});
}
}
|
Output : (Horizontal ScollBar)
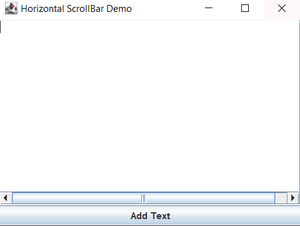
Extended ScrollBar:
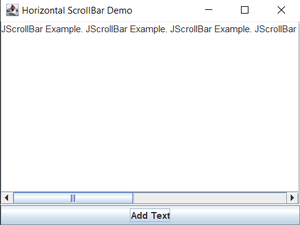
3. Java Program to Implement setMinimum() ,setMaximum() ,setValue() ,getValue() in JScrollBar
Java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class JScrollBarExample {
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> {
JFrame frame = new JFrame( "JScrollBar Example" );
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize( 400 , 300 );
JPanel contentPanel = new JPanel();
contentPanel.setLayout( new BorderLayout());
JScrollBar horizontalScrollBar = new JScrollBar(JScrollBar.HORIZONTAL);
horizontalScrollBar.setMinimum( 10 );
horizontalScrollBar.setMaximum( 100 );
horizontalScrollBar.setValue( 50 );
JLabel valueLabel = new JLabel( "Value: " + horizontalScrollBar.getValue());
horizontalScrollBar.addAdjustmentListener( new AdjustmentListener() {
@Override
public void adjustmentValueChanged(AdjustmentEvent e) {
int value = horizontalScrollBar.getValue();
valueLabel.setText( "Value: " + value);
}
});
contentPanel.add(horizontalScrollBar, BorderLayout.NORTH);
contentPanel.add(valueLabel, BorderLayout.CENTER);
frame.add(contentPanel);
frame.setVisible( true );
});
}
}
|
Output: (Setting Value According to ScrollBar)

Output Showing the value 90:
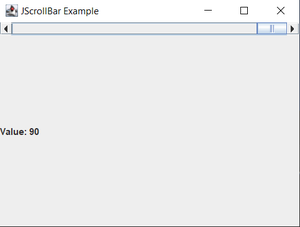
Share your thoughts in the comments
Please Login to comment...