Java JComponent
Last Updated :
27 Dec, 2023
In Java Swing, JComponent is an abstract class that programmers can use to modify to build unique components that are suited to the particular requirements of their applications. JComponent and other Swing components are lighter than their AWT equivalents. The Java runtime renders lightweight components directly, independent of the host operating system.
All Swing components, with the exception of top-level containers, are included in this core class. With the exception of JFrame and JDialog, which are top-level containers by definition and cannot be put under JComponent, all swing components with the letter “J” in their names are classified as JComponent.
JComponent offers event handling, helping developers respond to user events like mouse clicks, key presses, and other inputs.
Syntax of JComponent
public abstract class JComponent extends
Container implements Serializable
In the syntax given above, the JComponent is declared as an abstract class, extending the Container class and implementing the Serializable interface. This format indicates that JComponent supports serialization for object persistence and provides a foundation for building GUI components that are customizable. It also inherits container capability.
Nested Class of JComponent
The nested class of JComponent is given below which provides its internal class hierarchy.
Class
|
By default, the JComponent’s inner class is used to offer accessibility.
|
The nested classes of Java JComponent are inherited from the classes given below:
- java.awt.Container
- java.awt.Component
Constructor of JComponent
It is a default constructor initializes an empty JComponent.
|
JComponent Fields
Given below are the fields of JComponent that collects key information and attributes which affects the behavior of the component.
protected EventListenerList
|
A record of this component’s event listeners
|
static String
|
The label that appears when the cursor is over an element. It is referred to as a ‘value tip’, ‘flyover help’, or ‘flyover label’
|
protected ComponentUI
|
This component’s appearance and texture are assigned.
|
static int
|
Some APIs utilize this constant to indicate the absence of a specific condition.
|
static int
|
Always utilized for registerKeyboardAction. It indicates that the command must be executed when either the receiving component is the focused component or it is the focused component’s ancestor.
|
static int
|
It is the registerKeyboardAction constant that indicates the command should be executed when the component is the center of attention.
|
static int
|
The constant used for registerKeyboardAction indicates that the command should be executed when the receiving component is in the window with the focus or is the focused component itself.
|
The fields supported by the JComponent are inherited from:
- Class – java.awt.Component
- Interface – java.awt.image.ImageObserver
JComponent Methods
The JComponent class offers various methods. Following are some of the commonly used methods:
void
|
Alerts the component to the presence of a parent component.
|
boolean
|
Allows the UI delegate to specify this component’s exact form for mouse processing purposes.
|
float
|
Overrides Container.getAlignmentX to return the horizontal alignment.
|
float
|
Overrides Container.getAlignmentY to return the vertical alignment.
|
AncestorListener[]
|
Provides an array containing all of the registered ancestor listeners on this component.
|
boolean
|
Gets the autoscrolls property.
|
Border
|
Returns this component’s boundary or null if none is set at this time.
|
Rectangle
|
Returns rv after storing the component’s boundaries in “return value” rv.
|
Graphics
|
Specifies the visual context for this component, allowing you to draw on it.
|
int
|
Returns the current height of the component.
|
Dimension
|
Returns rv after storing the width and height of this component in the “return value” field.
|
ComponentUI
|
Returns the delegate’s appearance and feel used to render this component.
|
int
|
Returns the x coordinate of the component’s origin.
|
int
|
Returns the y coordinate of the component’s origin.
|
boolean
|
If this component is fully opaque, returns true.
|
void
|
Called by Swing to draw components.
|
protected void
|
Paints the border of the component.
|
protected void
|
Paints the children of the component.
|
void
|
To print the component to the designated Graphics, use this technique.
|
void
|
Use this method to print the component.
|
void
|
Defines the color of this component’s background.
|
void
|
Sets the border of this component.
|
void
|
Determines the font for this element.
|
void
|
Fix the foreground color of this component.
|
void
|
It points whether the component is visible or not visible.
|
void
|
The ActionMap is set to am.
|
void
|
It sets this component’s maximum size to a fixed number.
|
void
|
It sets this component’s minimum size to a constant value.
|
The methods supported my JComponent are inherited from the classes:
- java.awt.Component
- java.awt.Container
- java.lang.Object
Example of Java JComponent
Let us have a look at a simple example to demonstrate the use of JComponent easily.
Below is the Example of Java JComponent:
Java
import java.awt.*;
import javax.swing.*;
import javax.swing.JComponent;
public class Component extends JComponent {
protected void paintComponent(Graphics g)
{
super .paintComponent(g);
g.setColor(Color.BLUE);
g.fillRect( 20 , 20 , 50 , 50 );
g.setColor(Color.BLACK);
g.drawString( "Square" , 30 , 100 );
g.setColor(Color.RED);
g.fillOval( 90 , 20 , 50 , 50 );
g.setColor(Color.BLACK);
g.drawString( "Circle" , 100 , 100 );
int xPoints[] = { 150 , 200 , 250 };
int yPoints[] = { 20 , 70 , 20 };
g.setColor(Color.GREEN);
g.fillPolygon(xPoints, yPoints, 3 );
g.setColor(Color.BLACK);
g.drawString( "Triangle" , 170 , 100 );
}
public static void main(String[] args)
{
JFrame f = new JFrame( "JComponent Example" );
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
Component customComponent = new Component();
f.add(customComponent);
f.setSize( 300 , 200 );
f.setVisible( true );
}
}
|
Output of JComponent Example:
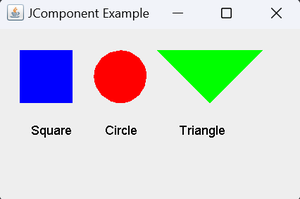
Explanation of the above Program:
In the above code, we have created a class named Component which extends the JComponent class. The paintComponent() method is overridden in the class. Swing calls this method automatically in order to render custom visuals. This method’s creates a green triangle, a red circle, and a blue square on the component. The main method instantiates an object of the Component class, adds it to the frame, and creates a JFrame. It also sets its properties, including the title and closing operation.
Demo GIF showing the Execution of the above Program:
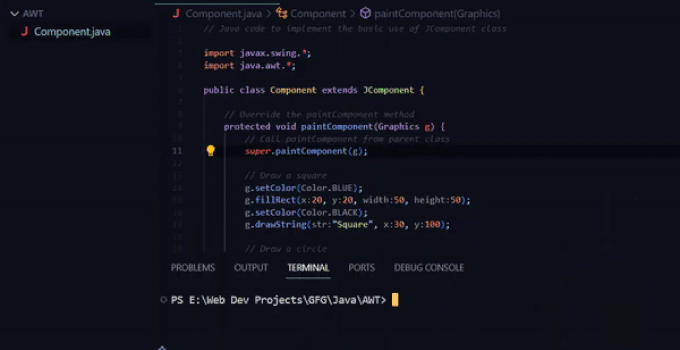
Share your thoughts in the comments
Please Login to comment...