Introduction to Modularity and Interfaces In System Design
Last Updated :
02 Apr, 2024
In software design, modularity means breaking down big problems into smaller, more manageable parts. Interfaces are like bridges that connect these parts together. This article explains how using modularity and clear interfaces makes it easier to build and maintain software, with tips for making systems more flexible and easy to understand.
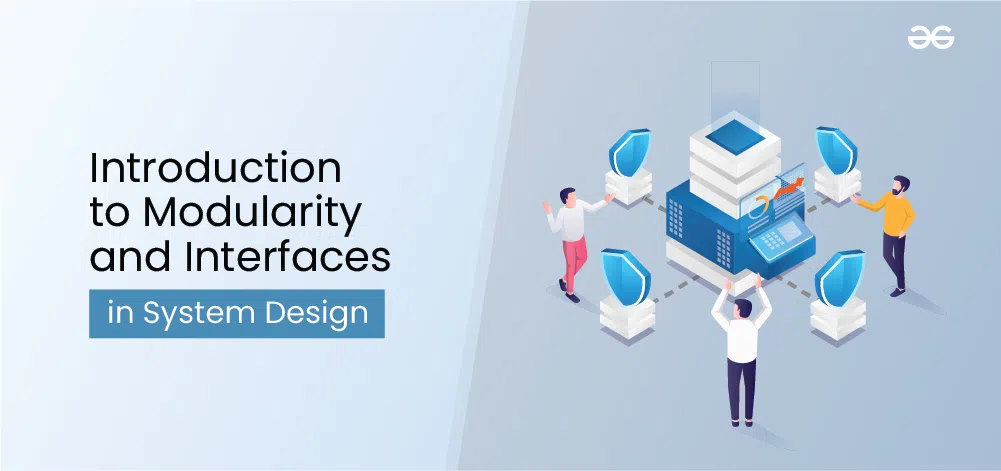
Important Topics for Modularity and Interfaces In System Design
What is Modularity?
Modularity in system design refers to the practice of breaking down a complex system into smaller, more manageable components or modules. Each module is designed to perform a specific function or task, and these modules are designed to work together to achieve the overall functionality of the system.
This approach is used in a variety of fields, including software engineering, mechanical engineering, and architecture, to simplify the development and maintenance process, reduce costs, and improve the reliability and flexibility of the system.Â
For example, in an object-oriented programming language like Java, a module might be represented by a class, which defines the data and behavior of a particular type of object.
Java
// Module 1: Addition module
public class AdditionModule {
public static int add(int a, int b) { return a + b; }
}
// Module 2: Subtraction module
public class SubtractionModule {
public static int subtract(int a, int b)
{
return a - b;
}
}
// Module 3: Multiplication module
public class MultiplicationModule {
public static int multiply(int a, int b)
{
return a * b;
}
}
// Module 4: Division module
public class DivisionModule {
public static double divide(int a, int b)
{
if (b != 0) {
return (double)a / b;
}
else {
System.out.println("Cannot divide by zero");
return Double.NaN; // Not a Number
}
}
}
// Main program
public class Main {
public static void main(String[] args)
{
int num1 = 10;
int num2 = 5;
// Using addition module
int resultAdd = AdditionModule.add(num1, num2);
System.out.println("Addition result: " + resultAdd);
// Using subtraction module
int resultSubtract
= SubtractionModule.subtract(num1, num2);
System.out.println("Subtraction result: "
+ resultSubtract);
// Using multiplication module
int resultMultiply
= MultiplicationModule.multiply(num1, num2);
System.out.println("Multiplication result: "
+ resultMultiply);
// Using division module
double resultDivide
= DivisionModule.divide(num1, num2);
System.out.println("Division result: "
+ resultDivide);
}
}
Characteristics of Modularity
The key characteristics of modularity include:
- Flexibility: Allows for easy customization and adaptation to changing requirements.
- Abstraction: Modules provide clear, high-level interfaces abstracting complex functionality.
- Collaboration: Facilitates parallel development by enabling teams to work on different modules independently.
- Testing: Modular systems are easier to test as each module can be tested separately, promoting robustness.
- Documentation: Encourages better documentation practices as module interfaces need to be well-defined and documented.
- Interchangeability: Modules can be swapped or upgraded without affecting the overall system functionality, promoting interoperability.
Key Components of Modular Design
Below are the key components of Modular Design
- Modules: These are the smaller, independent units that make up the overall system. Each module is designed to perform a specific function and is self-contained, with well-defined interfaces to other modules.
- Interfaces: These are the points of communication between modules. Interfaces define how the modules interact with each other and can include electrical, mechanical, or software connections.
- Subsystems: These are groups of modules that work together to perform a specific function within the overall system.
- Integration: This is the process of combining the individual modules into a cohesive whole and testing the overall system to ensure that it is functioning properly.
- Maintenance: This involves monitoring and updating the system as needed to ensure that it continues to operate effectively. This may involve modifying or replacing individual modules as needed.
- Documentation: This includes all of the technical and operational information about the system, including schematics, manuals, and instructions for use.
Overall, the goal of a modular design is to create a system that is flexible, efficient, reliable, and easy to maintain and update.
How does modular design work?
Modular design works by dividing a complex system into smaller, independent modules that can be developed and tested separately, and then integrated into the overall system. Each module is designed to perform a specific function and is self-contained, with well-defined interfaces to other modules. This allows different teams to work on different modules concurrently and enables the system to be easily modified or expanded by adding or replacing individual modules.
The process of modular design usually involves the following steps:
- Step1: Identify the functions and requirements of the system.
- Step2: Divide the system into smaller, independent modules based on function and/or requirement.
- Step3: Define the interfaces between the modules.
- Step4: Develop and test each module individually.
- Step5: Integrate the modules into the overall system and test the system as a whole.
- Step6: Maintain and update the system by modifying or replacing individual modules as needed.
Modular design can be applied to a wide range of systems, including mechanical systems, software systems, and buildings. It is often used to simplify the development and maintenance process, reduce costs, and improve the reliability and flexibility of the system.
Real-World Examples of Modular Design
Below are some of the real-world examples of Modular Design:
- Modular buildings: Prefabricated buildings that are constructed off-site and then assembled on-site using standardized components.
- Modular cars: Cars that are made up of interchangeable components, such as engines and transmissions, which can be easily replaced or upgraded.
- Modular electronics: Electronic devices that are made up of interchangeable modules, such as cell phones with removable batteries and interchangeable camera modules.
- Modular software: Software that is divided into independent modules that can be developed and tested separately and then integrated into the overall system.
Benefits of Modularity
- Improved flexibility: Modular designs allow individual components or modules to be easily added, removed, or replaced, making it easy to modify the product to meet changing needs or requirements.
- Increased efficiency: Modular designs enable different parts of the product to be developed and tested independently, allowing for faster development and more efficient use of resources.
- Improved quality: Modular designs can improve the overall quality of a product by allowing for more thorough testing of individual components and facilitating the use of higher-quality materials and construction techniques.
- Enhanced scalability: Modular designs can make it easier to scale a product up or down in terms of size, capacity, or functionality, by allowing for the addition or removal of modules as needed.
What are Interfaces?
In system design, an Interface is a set of rules or guidelines that define how different components of a system interact with each other. Interfaces specify the inputs, outputs, and behaviors of a component, as well as the ways in which other components in the system can use it.
By defining clear interfaces between components, designers can ensure that the different parts of a system work together seamlessly, even if they were developed by other teams or at different times.
For Example:
The code below defines a “Shape"
interface with methods for calculating area and perimeter, implemented by the “Circle"
class, which computes these values for a circle based on its radius. The Main
class demonstrates polymorphism by creating a Circle
object through the Shape
interface and invoking its methods.
Java
// Interface: Shape
interface Shape {
double calculateArea();
double calculatePerimeter();
}
// Class: Circle implementing Shape interface
class Circle implements Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
@Override
public double calculateArea() {
return Math.PI * radius * radius;
}
@Override
public double calculatePerimeter() {
return 2 * Math.PI * radius;
}
}
// Main program
public class Main {
public static void main(String[] args) {
Shape circle = new Circle(5);
System.out.println("Circle Area: " + circle.calculateArea());
System.out.println("Circle Perimeter: " + circle.calculatePerimeter());
}
}
Characteristics of Interfaces
- Abstraction: Interfaces provide a way to define a contract for functionality without specifying the implementation details. They define what operations are available without specifying how those operations are carried out.
- Encapsulation: Interfaces encapsulate the essential behavior of an entity. They hide the internal details of how a class or module achieves its functionality, allowing for a clear separation of concerns and promoting modular design.
- Polymorphism: Interfaces enable polymorphic behavior, allowing objects of different classes to be treated interchangeably if they implement the same interface. This promotes flexibility and reusability in code.
- Contract: Interfaces establish a contract between the implementing class and the rest of the system. Any class that implements an interface is obligated to provide implementations for all methods declared in that interface, ensuring consistency and predictability.
- Flexibility: Interfaces facilitate loose coupling between components by allowing classes to interact with each other based on the interfaces they implement rather than their concrete types. This promotes easier maintenance, testing, and evolution of software systems.
Real-World Example of Interface
USB (Universal Serial Bus) standard. USB defines a set of protocols and specifications for communication between devices and a host controller.
- The USB standard serves as the interface specification, defining the rules and protocols that devices must follow to communicate with each other.
- Various devices such as keyboards, mice, printers, cameras, and storage devices implement this interface specification. Each device adheres to the USB standard, ensuring compatibility and interoperability with other USB-compliant devices.
- From the perspective of the host controller (e.g., a computer or smartphone), it interacts with these devices through the common interface provided by the USB standard, regardless of the specific functionality or manufacturer of each device.
- This abstraction allows the host controller to communicate with a wide range of devices without needing to know the intricate details of each device’s implementation, demonstrating the principle of encapsulation and abstraction provided by interfaces in software design.
How Modularity and Interfaces work together?
Interfaces and modularity work together to promote flexible, maintainable, and scalable software systems through clear separation of concerns and well-defined points of interaction.
- Encapsulation:
- Interfaces define the external contract for modules or components, specifying the methods or operations they must implement. This encapsulation hides the internal details of each module, allowing them to be treated as black boxes.
- Modularity, on the other hand, breaks down the system into smaller, more manageable modules. Interfaces provide the means for these modules to interact with each other without exposing their internal implementations.
- Loose Coupling:
- Modularity aims to minimize dependencies between modules, reducing the risk of ripple effects when making changes to the system.
- Interfaces play a crucial role in achieving loose coupling by defining well-defined points of interaction between modules. This allows modules to communicate with each other based on contracts defined by interfaces, rather than relying on direct dependencies.
- Flexibility and Reusability:
- Interfaces enable polymorphism, allowing different modules to be substituted or replaced with alternative implementations as long as they adhere to the same interface. This promotes flexibility and reusability of modules within the system.
- Modularity further enhances this flexibility by allowing modules to be developed, tested, and maintained independently, facilitating easier integration and evolution of the system over time.
- Standardization and Documentation:
- Interfaces serve as standardized communication channels between modules, providing clear documentation of the expected behavior and interactions. This standardization promotes consistency and interoperability within the system.
- Modularity, by breaking down the system into modular components, facilitates the organization and management of these interfaces, making it easier for developers to understand and work with the system.
- Scalability:
- Interfaces and modularity together support the scalability of software systems. Modularity allows for incremental development and addition of new features by adding or modifying modules. Interfaces ensure that new modules can seamlessly integrate with existing ones as long as they adhere to the established interface contracts, enabling the system to grow and adapt to changing requirements without compromising its overall architecture.
Conclusion
In summary, modularity and interfaces are key techniques for designing and building complex systems. They allow teams to work on different parts of a system in parallel, and they provide a way for the different components to communicate and work together. Modularity and interfaces are often used together in system design, with modular components being connected through well-defined interfaces. This allows for greater flexibility and reuse of components, as well as easier debugging and maintenance of the overall system.
Share your thoughts in the comments
Please Login to comment...