How to Write an ArrayList of Strings into a Text File?
Last Updated :
02 Feb, 2024
In Java, ArrayList is a class in the java.util
package. It facilitates the creation of dynamic arrays that can dynamically grow or shrink as elements are added or removed. This class is part of the Java Collections Framework.
An ArrayList of strings is converted into a text file and implemented using the BufferWriter class along with the framework.
BufferWriter and FileWriter
- BufferWriter: It is a class in Java that is used to provide buffering for Writer instances. It makes the writing process more efficient to write the character to the file.
- FileWriter: It is a class in Java. It is used for writing character data into a file. It is a subclass of the Writer class and is part of the java.io package.
Program to write an ArrayList of Strings into a text file in Java
Java
import java.io.BufferedWriter;
import java.io.FileWriter;
import java.io.IOException;
import java.util.ArrayList;
public class GFGArrayListToTextFile {
public static void main(String[] args) {
ArrayList<String> strlst = new ArrayList<>();
strlst.add( "Welcome to" );
strlst.add( "Greeks for Greeks" );
strlst.add( "Java" );
strlst.add( "tutorial" );
String filePath = "C:/Users/mahalaxmi/Desktop/programs/output.txt" ;
try (BufferedWriter writer = new BufferedWriter( new FileWriter(filePath)))
{
for (String str : strlst) {
writer.write(str);
writer.newLine();
}
System.out.println( "ArrayList written to file successfully." );
} catch (IOException e) {
e.printStackTrace();
}
}
}
|
Executing the above Program:
javac GFGArrayListToTextFile.java
java GFGArrayListToTextFile
Output Text File:
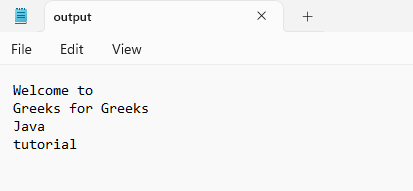
Explanation of the above Program:
- The above program is example of the ArrayList string convert into the text file using the BufferWriter along with FileWriter.
- We have created one ArrayList names as strlst, after that using taken one string as defined the file path where the text file will be saved then using BufferWriter and FileWriter to convert the ArrayList String into the text file.
Share your thoughts in the comments
Please Login to comment...