How to remove portion of a string after certain character in JavaScript ?
Last Updated :
15 Feb, 2023
Given a URL and the task is to remove a portion of URL after a certain character using JavaScript.
- split() method: This method is used to split a string into an array of substrings, and returns the new array. Syntax:
string.split(separator, limit)
- Parameters:
- separator: It is optional parameter. It specifies the character, or the regular expression, to use for splitting the string. If not used, the whole string will be returned (an array with only one item).
- limit: It is optional parameter. It specifies the integer that specifies the number of splits, items beyond the split limit will be excluded from the array.
- JavaScript String substring() Method: This method gets the characters from a string, between two defined indices, and returns the new sub string. This method gets the characters in a string between “start” and “end”, excluding “end” itself. Syntax:
string.substring(start, end)
- Parameters:
- start: It is required parameter. It specifies the position from where to start the extraction. Index of first character starts from 0.
- end: It is optional parameter. It specifies the position (excluding) where to stop the extraction. If not used, it extracts the whole string.
Example 1: This example uses the substring() method to remove the portion of the string after certain character (?).
html
<!DOCTYPE HTML>
< html >
< head >
< title >
Remove portion of string after
certain characters
</ title >
</ head >
< body style = "text-align:center;">
< h1 style = "color:green;" >
GeeksForGeeks
</ h1 >
< p id = "GFG_UP" style = "font-size: 15px; font-weight: bold;">
</ p >
< button onclick = "GFG_click()">
click to remove
</ button >
< p id = "GFG_DOWN" style =
"color:green; font-size: 20px; font-weight: bold;">
</ p >
< script >
var el_up = document.getElementById("GFG_UP");
var el_down = document.getElementById("GFG_DOWN");
var s = '/path/action?id=11612&value=44944';
el_up.innerHTML = s;
function GFG_click() {
s = s.substring(0, s.indexOf('?'));
el_down.innerHTML = "String = "+s;
}
</ script >
</ body >
</ html >
|
Output:
- Before clicking on the button:
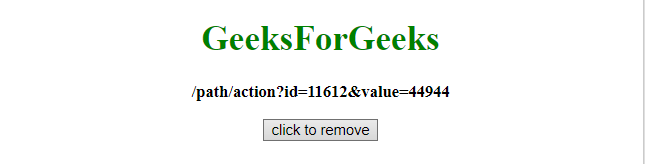
- After clicking on the button:
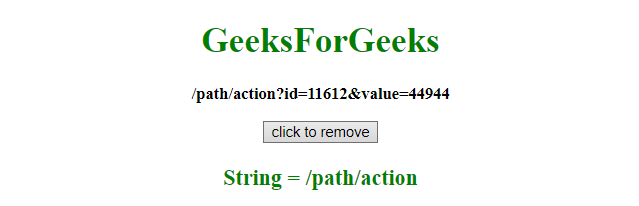
Example 2: This example uses the split() method to remove the portion of the string after certain character (?).
html
<!DOCTYPE HTML>
< html >
< head >
< title >
Remove portion of string after
certain character
</ title >
</ head >
< body style = "text-align:center;">
< h1 style = "color:green;" >
GeeksForGeeks
</ h1 >
< p id = "GFG_UP" style = "font-size: 15px; font-weight: bold;">
</ p >
< button onclick = "GFG_click()">
click to remove
</ button >
< p id = "GFG_DOWN" style =
"color:green; font-size: 20px; font-weight: bold;">
</ p >
< script >
var el_up = document.getElementById("GFG_UP");
var el_down = document.getElementById("GFG_DOWN");
var s = '/path/action?id=11612&value=44944';
el_up.innerHTML = s;
function GFG_click() {
s = s.split('?')[0]
el_down.innerHTML = "String = " + s;
}
</ script >
</ body >
</ html >
|
Output:
- Before clicking on the button:
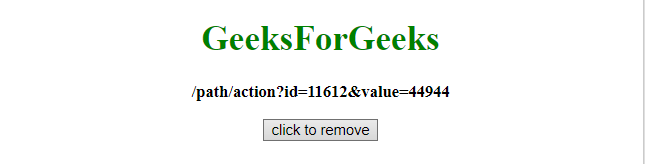
- After clicking on the button:
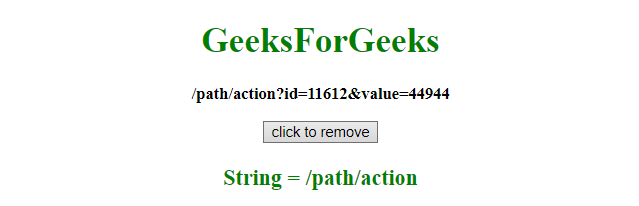
Share your thoughts in the comments
Please Login to comment...