How to Receive JSON Data at Server Side ?
Last Updated :
10 Mar, 2022
JavaScript Object Notation (JSON) is a data transferring format used to send data to or from the server. It is commonly utilized in API integration due to its benefits and simplicity. In this example, we will utilize XML HttpRequest to deliver data to the server.
Frontend: We will use a simple form containing name and email as an input and submit button to send it to the server. The data that is sent to a web server must be a string. As a result, we use the JSON.stringify() function to transform data to a string and transmit it to the server via an XHR request.
HTML
<!DOCTYPE html>
< html >
< body >
< h1 >GeeksForGeeks</ h1 >
< input type = "text" id = "name"
placeholder = "Your name" />
< input type = "email" id = "email"
placeholder = "Email" />
< button onclick = "sendJSONdata()" >
Submit
</ button >
< script >
function sendJSONdata() {
var name = document.getElementById("name");
var email = document.getElementById("email");
// Creating a XHR object
var xhr = new XMLHttpRequest();
// open a connection
xhr.open("POST", "/userdata");
// Setting the request header
xhr.setRequestHeader(
"Content-Type", "application/json"
);
// Converting JSON data to string
var data = JSON.stringify(
{ name: name.value, email: email.value }
);
xhr.send(data);
name.value = "";
email.value = "";
}
</ script >
</ body >
</ html >
|
Backend: We will use the Express framework and NodeJS to create the server which is running on port 3000. The post request by front-end on the /userdata route is handled here and one can see the data sent by the frontend by logging it with the help of the console.log() method.
Javascript
const express = require( "express" );
const bodyParser = require( "body-parser" );
const app = express();
app.use(bodyParser.json());
app.get( "/" , function (req, res) {
res.sendFile(__dirname + "/index.html" );
});
app.post( "/userdata" , function (req, res) {
console.log(req.body);
});
app.listen(3000, function () {
console.log( "Server started on port 3000" );
});
|
Output:
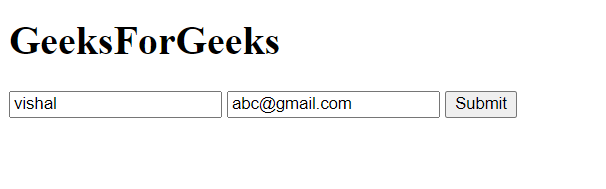
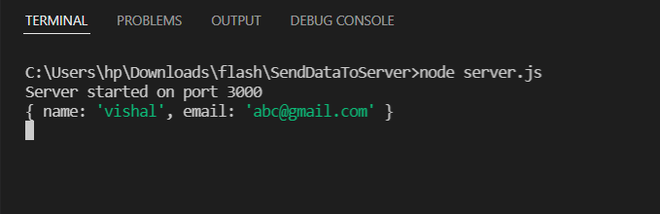
Share your thoughts in the comments
Please Login to comment...