How to make a request using HTTP basic authentication with PHP curl?
Last Updated :
29 Jan, 2024
Making secure API requests is a common requirement in web development, and PHP provides a powerful tool for this purpose, which is cURL. The challenge is to seamlessly integrate HTTP basic authentication with PHP cURL for secure API communication. This involves not only transmitting sensitive user credentials securely but also ensuring that the PHP application can successfully retrieve and process API responses. Here, we need to provide a way to address potential security concerns and facilitate the smooth integration of HTTP basic authentication with cURL in PHP.
HTTP Basic Authentication
HTTP basic authentication involves including a username and password in the request header. This is commonly used to secure APIs. PHP cURL provides the necessary functionality to include these credentials in your requests.
We start by initializing a cURL session and setting various options to configure the request. The crucial options include CURLOPT_RETURNTRANSFER for receiving the response as a string, CURLOPT_HTTPAUTH for specifying basic authentication, and CURLOPT_USERPWD for providing the username and password.
Using curl_setopt() Function
The curl_setopt() function empowers developers to tailor and enhance cURL sessions, ensuring resilient authentication mechanisms and robust communication between applications and APIs.
Example 1: Implementation for making a request using HTTP basic authentication with PHP curl.
PHP
<?php
$ch = curl_init( $apiUrl );
curl_setopt( $ch , CURLOPT_RETURNTRANSFER, true);
curl_setopt( $ch , CURLOPT_HTTPHEADER,
[ "User-Agent: phpApplication" ]);
$response = curl_exec( $ch );
if (curl_errno( $ch )) {
echo "Curl error: " . curl_error( $ch );
}
curl_close( $ch );
$data = json_decode( $response , true);
if ( $data ) {
print_r( $data );
} else {
echo "Error fetching data from GitHub API." ;
}
?>
|
Explaination
- Set the GitHub API endpoint for retrieving organizations of the user “hadley.” Initialize a cURL session.
- Configure cURL options, such as returning the response as a string and setting the User-Agent header.
- Execute the cURL request and store the response.
- Check for cURL errors and display an error message if there is an issue.
- Parse the JSON response into a PHP array.
- Check if the decoding was successful and print organizations or an error message accordingly.
Output:
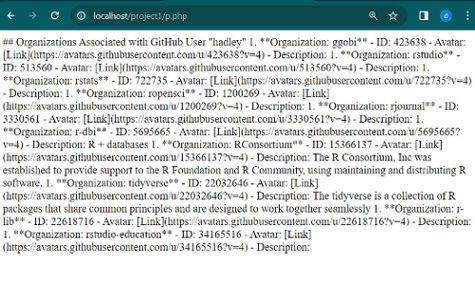
Example 2: Here, we are making a simple GET request to an API endpoint without using any specific authentication credentials.
PHP
<?php
$ch = curl_init( $apiUrl );
curl_setopt( $ch , CURLOPT_RETURNTRANSFER, true);
$response = curl_exec( $ch );
if (curl_errno( $ch )) {
echo "Curl error: " . curl_error( $ch );
}
curl_close( $ch );
echo "API Response:\n" ;
echo $response ;
?>
|
Explaination
- Define API URL which will set the variable $apiUrl with the target API endpoint.
- Initialize cURL that will use curl_init($apiUrl) to start a cURL session.
- Configure Options which use curl_setopt() to set cURL options for GET request and response handling.
- Execute cURL, which use curl_exec($ch) to make the API request and store the response in $response.
- Check for Errors, whiich use curl_errno($ch) to identify and echo any cURL errors.
- Close cURL Session & use curl_close($ch) to finish the cURL session.
- Process Response, and echo the API response ($response) for further use or display.
Output: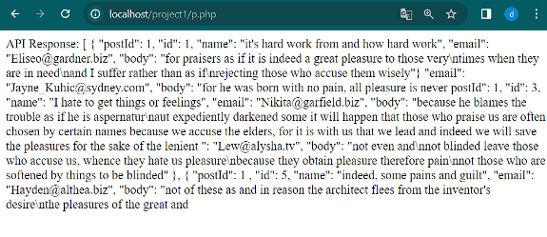
These techniques ensure that your PHP applications communicate securely with external APIs while handling authentication seamlessly.
Share your thoughts in the comments
Please Login to comment...