How to Handle Network Timeouts and Retries in Java?
Last Updated :
26 Apr, 2024
In Java, handling network timeouts and retries can be achieved the using different libraries and techniques. One common approach involved using built-in classes such as “HttpURLConnection” or external libraries such as Apache HttpClient. By setting appropriate timeouts and implementing the retry logic and developers can enhance the resilience and reliability of the Java applications and ensure smooth operations even the adverse network conditions.
Prerequisites:
The following are the prerequisites to handle network timeouts and retries in Java.
- Networking Fundamentals
- Exception Handling
- Basic Java Syntax
- Maven or Gradle (Optional)
- HTTP Concepts (Optional)
Example
Step 1: Create a Java Class file in a New Java Project in Eclipse IDE
- Open Eclipse, Create a Java project, and name it “NetworkHandler“.
- After that create a new Java class file, and name it “NetworkHandler“.
Here is the path for the Java class file in a Java project:
Step 2: Implement the code
Java
// Java Program to handle network
// timeouts and retries
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
import java.net.SocketTimeoutException;
// Class to handle network connections
public class NetworkHandler {
// Main method to demonstrate network connection handling
public static void main(String[] args) {
// Call the method to handle network connection
handleConnection();
}
// Method to handle network connection
public static void handleConnection() {
// URL to connect to
String urlStr = "http://google.com";
// Maximum number of retries
int maxRetries = 3;
// Interval between retries in milliseconds (1 second)
int retryInterval = 1000;
try {
// Create URL object from the URL string
URL url = new URL(urlStr);
// Perform multiple retries
for (int i = 0; i < maxRetries; i++) {
// Open connection to the URL
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
// Set connection timeout to 5 seconds
connection.setConnectTimeout(5000);
// Set read timeout to 5 seconds
connection.setReadTimeout(5000);
// Get response code from the connection
int responseCode = connection.getResponseCode();
// Check if response code is HTTP_OK (200)
if (responseCode == HttpURLConnection.HTTP_OK) {
// If successful, read the response
BufferedReader reader = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String line;
StringBuilder response = new StringBuilder();
while ((line = reader.readLine()) != null) {
response.append(line);
}
reader.close();
// Handle the response here (e.g., print it)
System.out.println("Response from server:");
System.out.println(response.toString());
// Close the connection
connection.disconnect();
// Break the loop as the request was successful
break;
} else {
// Retry logic can be added here for
// specific response codes if needed
}
}
} catch (SocketTimeoutException e) {
// Handle timeout exception
System.out.println("Timeout occurred");
} catch (IOException e) {
// Handle other IO exceptions
e.printStackTrace();
}
}
}
Explanation of the above Program:
- In the above example, after establish the connection, it will be check the response code with the help of “connection.getResponseCode()” method.
- If the response code will be “HTTP_OK” (200), it will read the response from the input stream using the “BufferedReader“.
- The response is then the processed as needed. In the above example, it is simply print the response in the console window.
- Finally, the connection will be closed with the help of “connection.disconnect()” method.
Step 3: Run the Code
- After implement the code, you need to run the code.
- For run the code, right click on the java project and then select Run As > Java Application.
If the connection to the “http://google.com” is successful.
The output will be shown in your console window in your Eclipse IDE.
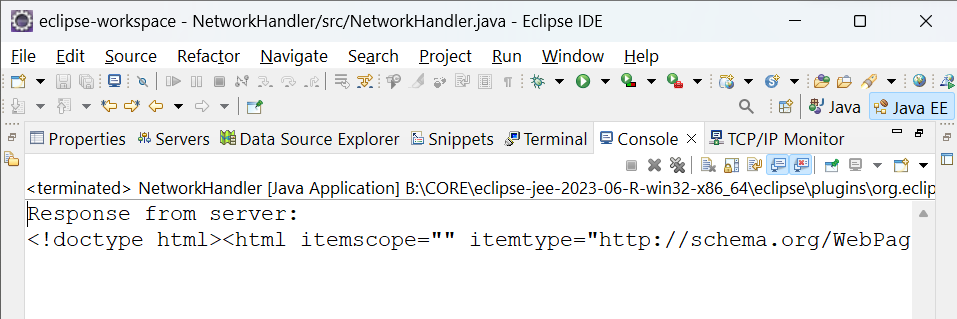
Output
Share your thoughts in the comments
Please Login to comment...