How to Handle Memory Leaks in JavaScript ?
Last Updated :
12 Apr, 2024
JavaScript, unlike some other programming languages, uses automatic memory management. This means the developer doesn’t have to explicitly free up memory when they’re done with it. However, memory leaks can still happen in JavaScript if objects are not properly cleaned up. A memory leak is a situation where memory is allocated for objects that are no longer being used by the program, but the garbage collector can’t reclaim that memory because the objects are still being referenced somewhere. This can lead to performance slowdowns and crashes as the application continues to run.
The benefit of Addressing Memory Leaks in JavaScript
Addressing memory leaks is crucial for maintaining the efficiency and stability of JavaScript applications and many more.
- Improved Performance: Memory leaks can gradually degrade the performance of a JavaScript application over time by consuming more memory than necessary. By addressing memory leaks, you can prevent excessive memory usage and maintain optimal performance.
- Stability: Memory leaks can lead to unpredictable behavior in an application, such as crashes or freezes, especially in long-running applications or web pages.
- Faster Page Load Times: When memory is abundant, your web pages can load faster. Handling memory leaks ensures enough memory is available for efficient page loading, leading to a better user experience.
- Reduced Resource Consumption: Memory leaks can cause your application to consume more and more memory over time.
- Enhanced User Experience: Memory leaks can negatively impact the user experience by causing slowdowns, crashes, or unexpected behavior. By handling memory leaks, you can provide a smoother and more reliable user experience, leading to higher user satisfaction and retention.
There are several approaches available in JavaScript to handle memory leak which are as follows:
Clearing Event Listeners
Event listeners are often attached to DOM elements to handle user interactions such as clicks, mouse movements, etc. If these event listeners are not removed properly when the associated DOM elements are removed or no longer needed, they can lead to memory leaks. Remove event listeners using the removeEventListener() method when the associated DOM element is removed or when the event listener is no longer needed.
Syntax:
element.removeEventListener(eventType, eventHandler);
Example: Dynamic Event Listener Removal
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>Clearing Event Listeners Example</title>
<style>
body {
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
gap: 10px;
}
button {
padding: 10px 20px;
border-radius: 5px;
background-color: green;
color: white;
cursor: pointer;
}
button:hover {
background-color: rgb(226, 231, 228);
color: black;
}
</style>
</head>
<body>
<div>
<h2>Click the Button</h2>
</div>
<button id="myButton">Click Me</button>
<script>
// Assume we have a button
// element with id="myButton"
let button = document
.getElementById('myButton');
// Assume we have a function named
// handleClick that was used as the event listener
function handleClick(event) {
alert('Button clicked');
}
// Add the event
// listener to the button
button
.addEventListener('click', handleClick);
// Later, if we want
// to remove the event listener
let timeOutId = setTimeout(() => {
button
.removeEventListener('click', handleClick);
alert('Removing button event listner.')
clearTimeout(timeOutId)
}, 7000);
</script>
</body>
</html>
Output
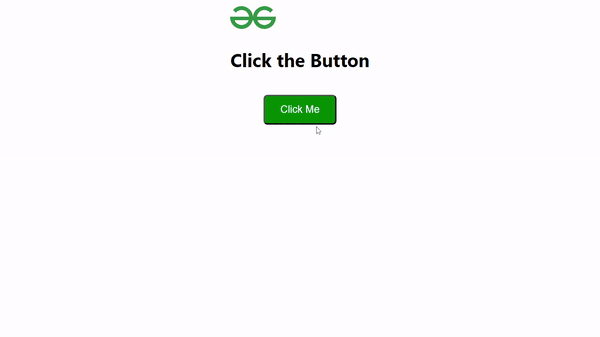
Clearing Event Listener Output
Avoiding Global Variables
Global variables in JavaScript are variables declared outside of any function or block scope. They persist throughout the lifecycle of the application and can potentially lead to memory leaks if they hold references to objects that are no longer needed. Avoiding excessive use of global variables is crucial for managing memory effectively. To avoid memory leaks caused by global variables in JavaScript, it’s essential to minimize their usage and scope. This involves encapsulating variables within functions or modules to limit their visibility and lifespan.
Syntax:
let globalVar = 'This is a global variable'; // this is a global variable
globalVar = null; // free up space
Example: Define variables within a function scope to avoid memory leaks. To avoid this, use function-level-variable, meaning that variables declared within a function are only accessible within that function.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>Using Scope Variables</title>
<style>
body {
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
gap: 10px;
}
button {
padding: 10px 20px;
border-radius: 5px;
background-color: green;
color: white;
cursor: pointer;
}
button:hover {
background-color: rgb(226, 231, 228);
color: black;
}
</style>
</head>
<body>
<div>
<img src=
"https://media.geeksforgeeks.org/gfg-gg-logo.svg"
alt="gfg_logo">
<h2>Click the Button</h2>
</div>
<button id="increment">Increment Counter</button>
<button id="decrement">Decrement Counter</button>
<p>Counter Value: <span id="counter"></span></p>
<script>
; (function () {
// Define a scope variable
// to hold the counter value
let counter = 0;
// Function to increment the counter
function incrementCounter() {
counter++;
updateCounterDisplay();
}
// Function to decrement the counter
function decrementCounter() {
counter--;
updateCounterDisplay();
}
// Function to update the
// counter display in the HTML
function updateCounterDisplay() {
document
.getElementById('counter')
.textContent = counter;
}
document
.getElementById('increment')
.addEventListener('click', incrementCounter)
document
.getElementById('decrement')
.addEventListener('click', decrementCounter)
})()
</script>
</body>
</html>
Output
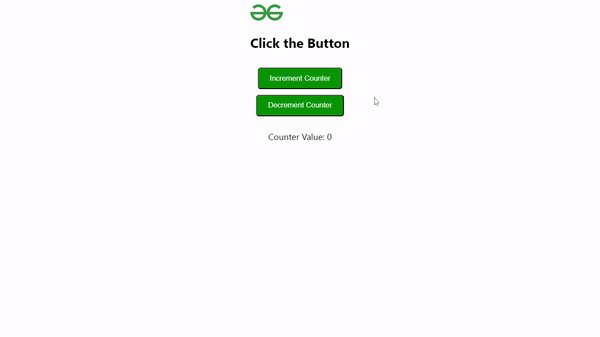
Counter Project Output
Managing DOM References
When working with the Document Object Model (DOM) in JavaScript, it’s common to create references to DOM elements for manipulation. However, if these references are not properly managed, they can lead to memory leaks when the associated DOM elements are removed from the document. The approach involves ensuring that references to DOM elements are properly cleaned up when the elements are no longer needed or when they are removed from the DOM. This typically involves setting the references to null or removing them entirely.
Syntax:
element.parentNode.removeChild(element);
// OR
element.innerHTML = '';
// OR
element = null;
Example: Null the variables those hold DOM references
HTML
<!DOCTYPE html>
<html>
<head>
<title>Page Title</title>
</head>
<body>
<h2>Welcome To GFG</h2>
<ul id="itemList">
<li id="item1">Item 1</li>
<li id="item2">Item 2</li>
<li id="item3">Item 3</li>
</ul>
</body>
<script>
// Get a reference
// to the list
let list = document
.getElementById('itemList');
// Function to remove
// an item from the list
function removeItem(itemId) {
let itemToRemove = document
.getElementById(itemId);
list
.removeChild(itemToRemove);
// Ensure reference is cleaned up
itemToRemove = null;
}
// Remove an item from
// the list after 5 seconds
setTimeout(function() {
removeItem('item2');
}, 5000);
</script>
</html>
Output
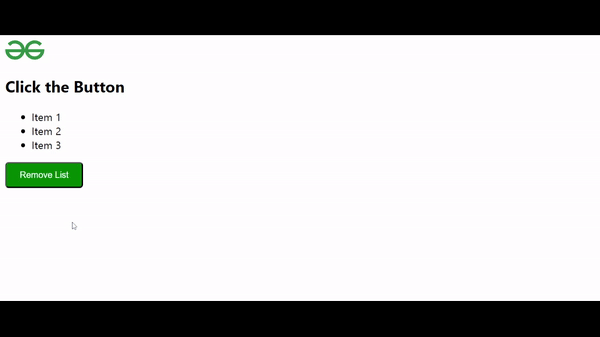
Managing DOM Reference Output
WeakMap
WeakMap is a collection of key-value pairs where keys are objects and values can be arbitrary values. Unlike regular maps, the keys in a WeakMap are weakly held, meaning they won’t prevent garbage collection of the key object. WeakMap is often used to associate additional data with objects without preventing those objects from being garbage collected.
Syntax:
let weakMap = new WeakMap();
let obj = {};
weakMap.set(obj, 'metadata');
Example: Private Data Management with WeakMap
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>WeakMap Example</title>
<style>
body {
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
gap: 10px;
}
button {
padding: 10px 20px;
border-radius: 5px;
background-color: green;
color: white;
cursor: pointer;
}
button:hover {
background-color: rgb(226, 231, 228);
color: black;
}
</style>
</head>
<body>
<div>
<img src=
"https://media.geeksforgeeks.org/gfg-gg-logo.svg"
alt="gfg_logo">
<h2>Click the Button</h2>
</div>
<button id="btn1">Button 1</button>
<button id="btn2">Button 2</button>
<script>
// Create a WeakMap to store
// private data associated with DOM elements
let privateData = new WeakMap();
// Function to associate
// private data with a DOM element
function setPrivateData(element, data) {
privateData.set(element, data);
}
// Function to retrieve private
// data associated with a DOM element
function getPrivateData(element) {
return privateData.get(element);
}
// Example usage:
// Retrieve the buttons from the DOM
let button1 = document
.getElementById('btn1');
let button2 = document
.getElementById('btn2');
// Associate private data with each button
setPrivateData(button1,
{ secretInfo: 'Button 1 is sensitive' });
setPrivateData(button2,
{ secretInfo: 'Button 2 is confidential' });
// Click event listener for button 1
button1.addEventListener('click', function () {
// Retrieve and log private
// data associated with button 1
let data = getPrivateData(button1);
alert(data.secretInfo);
});
// Click event listener for button 2
button2.addEventListener('click',
function () {
// Retrieve and log private
// data associated with button 2
let data = getPrivateData(button2);
alert(data.secretInfo);
});
// 7 second button 2 is deleted
let timeOutId = setTimeout(() => {
button2
.parentElement
.removeChild(button2)
clearTimeout(timeOutId)
alert('Button 2 is removed')
}, 7000);
</script>
</body>
</html>
Output
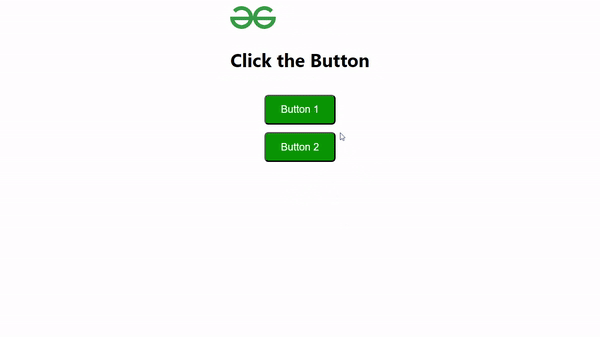
Weak Map Output
WeakSet
WeakSet is a collection of objects where each object can occur only once. Similar to WeakMap, the objects stored in a WeakSet are weakly held, meaning they won’t prevent garbage collection. WeakSet is useful when you need to store a collection of objects but want those objects to be garbage collected when no longer referenced elsewhere in the code.
Syntax:
let weakSet = new WeakSet();
let obj1 = {};
let obj2 = {};
weakSet.add(obj1);
weakSet.add(obj2);
Example: Efficient Event Listener Management with WeakSet
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>WeakSet Example</title>
<style>
body {
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
gap: 10px;
}
button {
padding: 10px 20px;
border-radius: 5px;
background-color: green;
color: white;
cursor: pointer;
}
button:hover {
background-color: rgb(226, 231, 228);
color: black;
}
</style>
</head>
<body>
<div>
<img src=
"https://media.geeksforgeeks.org/gfg-gg-logo.svg"
alt="gfg_logo">
<h2>Click the Button</h2>
</div>
<button id="btn1">Button 1</button>
<button id="btn2">Button 2</button>
<script>
// Create a WeakSet
// to store event listeners
let eventListeners = new WeakSet();
// Function to add an event
// listener to a DOM element
function addEventListener(element, eventName, callback) {
// Create a listener function t
// hat wraps the original callback
function listener(event) {
callback(event);
}
// Add the listener to the element
element
.addEventListener(eventName, listener);
// Add the listener to the WeakSet
eventListeners.add(listener);
}
// Example usage:
// Retrieve the buttons from the DOM
let button1 = document
.getElementById('btn1');
let button2 = document
.getElementById('btn2');
// Function to handle button clicks
function handleClick(event) {
alert(`Button clicked: ${event.target.textContent}`);
}
// Add event listeners to the buttons
addEventListener(button1, 'click', handleClick);
addEventListener(button2, 'click', handleClick);
// At this point, the event
// listeners are attached to the buttons
// Remove the first button from the document
let timeOutId = setTimeout(() => {
button1
.parentNode
.removeChild(button1);
clearTimeout(timeOutId)
alert('Removing Button 2');
}, 8000)
</script>
</body>
</html>
Output
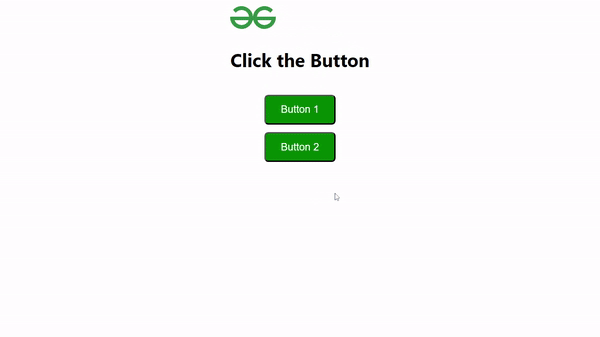
Weak Set Output
Share your thoughts in the comments
Please Login to comment...