How to get the POST values from serializeArray in PHP ?
Last Updated :
24 Apr, 2024
When working with forms in web development, it’s common to use JavaScript to serialize form data into a format that can be easily sent to the server. One popular method for serializing form data is using jQuery’s serializeArray() function.
However, once the data is serialized and sent to the server via a POST request, you need to retrieve and process it in your PHP script.
Below are the different approaches for extracting and handling the POST values from serializeArray() in PHP.
By Parsing the Serialized Data Manually
In this approach, you manually parse the serialized data in PHP by splitting the string and extracting the key-value pairs.
Example: This example shows the implementation of the above approach.
PHP
<?php
$serializedData = 'name=John&email=john@example.com&age=30';
$pairs = explode('&', $serializedData);
$data = [];
foreach ($pairs as $pair) {
list($key, $value) = explode('=', $pair);
$data[urldecode($key)] = urldecode($value);
}
print_r($data);
?>
Output:
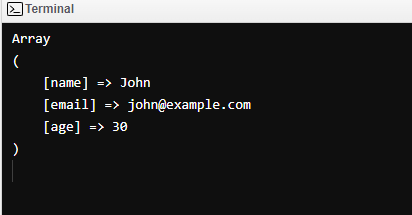
Using the parse_str() Function
The parse_str() function in PHP can be used to parse query strings into variables. This function can also be used to parse serialized form data.
Syntax:
parse_str($serializedData, $data);
Example: This example shows the implementation of the above approach.
PHP
<?php
$serializedData = 'name=John&email=john@example.com&age=30';
parse_str($serializedData, $data);
print_r($data);
?>
OutputArray
(
[name] => John
[email] => john@example.com
[age] => 30
)
Using json_decode() method
If you’re serializing form data using serializeArray() and sending it as JSON data, you can decode the JSON string in PHP to retrieve the values.
Syntax:
json_decode($serializedJSON, true);
Example: This example shows the implementation of the above approach.
PHP
<?php
$serializedJSON = '{"name":"John",
"email":"john@example.com",
"age":"30"}';
$data = json_decode($serializedJSON, true);
print_r($data);
?>
Output: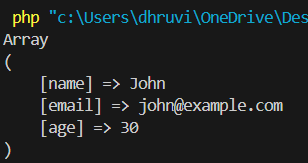
Share your thoughts in the comments
Please Login to comment...