How to Create a New Line in JavaScript ?
Last Updated :
23 Jan, 2024
In JavaScript, a new line is typically represented by a specific escape sequence or character within a string. This is commonly used in various programming languages to control the formatting of text and create multiline strings. A new line can be made in JavaScript using the below-discussed methods.
Using Escape Sequence `\n`
The \n escape sequence represents a newline character in JavaScript strings and it can used to render a new line in JavaScript.
Example: The below code uses the escape sequence `\n` to add a new line in JavaScript.
Javascript
console.log( "GFG\nGeeksforGeeks is a computer science portal for geeks." );
|
Output
GFG
GeeksforGeeks is a computer science portal for geeks.
Using ECMAScript6 (ES6) template literals
In ECMAScript6, the backticks (“) are used to create a template literal. Template literals can span multiple lines without the need for escape characters, making it easier to write and read multiline strings in JavaScript.
Example: The below code uses the ES6 template literals to add a new line in JavaScript.
Javascript
console.log(`Q: What is GeeksforGeeks?
A: GeeksforGeeks is a computer science portal for geeks.`);
|
Output
Q: What is GeeksforGeeks?
A: GeeksforGeeks is a computer science portal for geeks.
Using JavaScript console.log() Method
The console.log() method is a part of the JavaScript Console API, which provides methods for interacting with the browser’s console. It also returns a new line when the method is called and utilized so this approach can be used in place of an escape character.
Example: The below code uses the console.log() method to add a new line in JavaScript.
Javascript
console.log( 'GFG' );
console.log();
console.log( 'GeeksforGeeks' );
|
Using JavaScript document.write() Method
The document.write() method is a part of the JavaScript Document Object Model (DOM) API. It is used to dynamically write content to a document, specifically the HTML document. This method is especially useful if a new line has to be added or rendered on an HTML page using the <br> tag.
Example: The below code uses the document.write() method to add a new line in an HTML document using JavaScript.
Javascript
document.write ( "A computer science portal" );
document.write ( "<br>" );
document.write ( "for geeks." );
|
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content = "width=device-width, initial-scale=1.0" >
< title >HTML Document</ title >
</ head >
< body >
< h1 style = "color:green;" >GeeksforGeeks</ h1 >
< script src = "./script.js" ></ script >
</ body >
</ html >
|
Output:
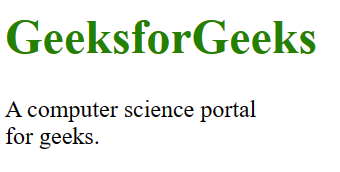
Using JavaScript document.writeln() Method
This method is especially useful if a new line has to be added to an HTML page. The only difference between this method and document.write() is that the document.writeln() automatically adds a new line after the rendered content instead of adding the <br> tag as done in the previous approach.
Example: The below code uses the document.writeln() method to add a new line in an HTML document using JavaScript.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content =
"width=device-width, initial-scale=1.0" >
< title >HTML Document</ title >
</ head >
< body >
< h1 style = "color:green;" >
GeeksforGeeks
</ h1 >
< script >
document.writeln
("A computer science");
document.writeln
("portal for geeks.");
</ script >
</ body >
</ html >
|
Output:
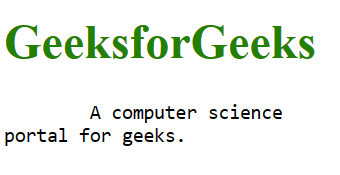
Share your thoughts in the comments
Please Login to comment...