How to convert Number to Boolean in JavaScript ?
Last Updated :
29 Dec, 2023
We convert a Number to Boolean by using the JavaScript Boolean() method and double NOT operator(!!). A JavaScript boolean results in one of two values i.e. true or false. However, if one wants to convert a variable that stores integer “0” or “1” into Boolean Value i.e. “false” or “true”.
Below are the approaches:
Approach 1: Boolean() method
The boolean function returns the boolean value of the variable. It can also be used to find the boolean result of a condition, expression, etc
Syntax:
Boolean(variable/expression)
Example: This example converts a number into a boolean.
HTML
<!DOCTYPE html>
< html >
< body >
< center >
< h1 style = "color:green" >
GeeksforGeeks
</ h1 >
< h4 >
Click the button to change the
number value into boolean.
</ h4 >
< button onclick = "myFunction()" >Change</ button >
< p >The number value of the variable is :</ p >
< p id = "result" ></ p >
< script >
// Initializing boolvalue as true
let numvalue = 1;
// JavaScript program to illustrate boolean
// conversion using ternary operator
function myFunction() {
document.getElementById("result")
.innerHTML = Boolean(numvalue);
}
</ script >
</ center >
</ body >
</ html >
|
Note: If the above Boolean() method is called as Boolean(!numvalue), it shows the result as “false“. Similarly, if it is called Boolean(!!numvalue), it gives the result as “true“.
Output:
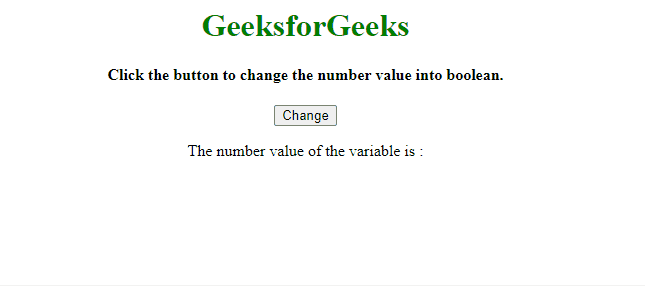
Approach 2: Using double NOT(!!) operator
This operator converts numbers with the value of zero to false and all other numbers to true.
Example: In this example, we will use the double NOT operator to convert the number into a boolean value.
HTML
<!DOCTYPE html>
< html >
< body >
< center >
< h1 style = "color:green" >
GeeksforGeeks
</ h1 >
< h4 >
Click the button to change the
number value into boolean.
</ h4 >
< button onclick = "myFunction()" >Change</ button >
< p >The number value of the variable is :</ p >
< p id = "result" ></ p >
< script >
// Initializing boolvalue as true
let numvalue = (!!1)
// JavaScript program to illustrate boolean
// conversion using ternary operator
function myFunction() {
document.getElementById("result")
.innerHTML = (numvalue);
}
</ script >
</ center >
</ body >
</ html >
|
Output:
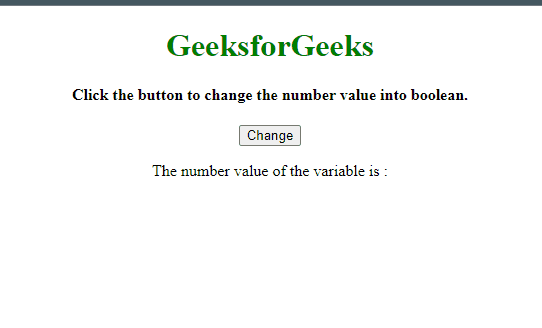
Share your thoughts in the comments
Please Login to comment...