How to Configure AuditListener in Spring Boot Application
Last Updated :
03 Mar, 2024
Spring Boot Data provides support to transparently keep track of who created an entity or changed an entity and when these things happened. All records are maintained clearly. For example, if one user comes into the site and logs in to the site. Then those times are maintained in the database and also when another time the user logs in to the site, those records are also maintained in the database that’s things, we can say auditing.
In this article, we will be implementing the configuration of the AuditListener in the Spring Boot Application.
Step-By-Step Implementation to Configure AuditListener in Spring Boot Application
Below are the steps to Configure AuditListener in a simple Spring Boot application.
Step 1: First, we will create a User Class.
User.java
Java
package org.technous.batchPrac3.model;
import jakarta.persistence.*;
import lombok.AllArgsConstructor;
import lombok.Getter;
import lombok.NoArgsConstructor;
import lombok.Setter;
@Getter
@Setter
@AllArgsConstructor
@NoArgsConstructor
@Entity
@Table (name = "user" )
public class User {
@Id
@GeneratedValue (strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private String email;
}
|
This class is user class to maintain the user details and save these data into the database.
Step 2: Now Implement service class.
Java
package org.technous.batchprac2.service.impl;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.technous.batchprac2.dto.UserDTO;
import org.technous.batchprac2.exception.ResourceNotFoundException;
import org.technous.batchprac2.model.User;
import org.technous.batchprac2.repository.UserRepository;
import org.technous.batchprac2.service.UserService;
import java.util.List;
@Service
public class UserServiceIMPL implements UserService {
@Autowired
private UserRepository userRepository;
public User userToDto(UserDTO userDTO){
User user = new User();
user.setFirstName(userDTO.getFirstName());
user.setLastName(userDTO.getLastName());
user.setEmailId(userDTO.getEmailId());
return user;
}
public UserDTO dtoToUser(User user){
UserDTO userDTO= new UserDTO();
userDTO.setFirstName(user.getFirstName());
userDTO.setLastName(user.getLastName());
userDTO.setEmailId(user.getEmailId());
return userDTO;
}
@Override
public List<UserDTO> getAllUsers() {
List<User> user = userRepository.findAll();
return null ;
}
@Override
public UserDTO getUserById(Long userId) throws ResourceNotFoundException {
User user = userRepository.findById(userId)
.orElseThrow((()-> new ResourceNotFoundException( "Employee not Found" )));
return dtoToUser(user);
}
@Override
public UserDTO createUser(UserDTO userDTO) {
User ruser = userToDto(userDTO);
User rruser = userRepository.save(ruser);
return dtoToUser(rruser);
}
@Override
public UserDTO updateUser(Long userId, UserDTO user) throws ResourceNotFoundException {
User useru = userRepository.findById(userId)
.orElseThrow(() -> new ResourceNotFoundException( "User not found :: " + userId));
useru.setEmailId(user.getEmailId());
useru.setLastName(user.getLastName());
useru.setFirstName(user.getFirstName());
User updatedUser = userRepository.save(useru);
return dtoToUser(updatedUser);
}
}
|
The above code is for the service layer, where we write all the business logic to save user data into database.
Step 3: Now we will create the Endpiont
UserController.java
Java
package org.technous.batchprac2.controller;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.*;
import org.technous.batchprac2.dto.UserDTO;
import org.technous.batchprac2.exception.ResourceNotFoundException;
import org.technous.batchprac2.model.User;
import org.technous.batchprac2.service.UserService;
import java.util.List;
@RestController
@RequestMapping ( "/api" )
public class UserController {
@Autowired
private UserService userService;
@GetMapping ( "/users" )
public List<UserDTO> getAllUsers() {
return userService.getAllUsers();
}
@GetMapping ( "/users/{id}" )
public ResponseEntity<UserDTO> getUserById(
@PathVariable (value = "id" ) Long userId) throws ResourceNotFoundException {
UserDTO user = userService.getUserById(userId);
return ResponseEntity.ok().body(user);
}
@PostMapping ( "/users" )
public UserDTO createUser( @RequestBody UserDTO userDTO) {
return userService.createUser(userDTO);
}
@PutMapping ( "/users/{id}" )
public ResponseEntity<UserDTO> updateUser(
@PathVariable (value = "id" ) Long userId,
@RequestBody UserDTO userDetails) throws ResourceNotFoundException {
UserDTO user = userService.updateUser(userId,userDetails);
return ResponseEntity.ok(user);
}
}
|
This code is the Endpoint and RestController class to call API and our user save into database. Now we need to save our user with auditing means we are tracking information when our user has saved when they update, so automatically they update time and data of the system.
Step 4: Now, create the Auditable class, that continuously monitor the application.
Java
package org.technous.batchprac2.audit;
import jakarta.persistence.EntityListeners;
import jakarta.persistence.MappedSuperclass;
import jakarta.persistence.Temporal;
import lombok.Getter;
import lombok.Setter;
import org.springframework.data.annotation.CreatedBy;
import org.springframework.data.annotation.CreatedDate;
import org.springframework.data.annotation.LastModifiedBy;
import org.springframework.data.annotation.LastModifiedDate;
import org.springframework.data.jpa.domain.support.AuditingEntityListener;
import java.util.Date;
import static jakarta.persistence.TemporalType.TIMESTAMP;
@Setter
@Getter
@MappedSuperclass
@EntityListeners (AuditingEntityListener. class )
public abstract class AudiTable<U> {
@CreatedBy
protected U createdBy;
@CreatedDate
@Temporal (TIMESTAMP)
protected Date createdDate;
@LastModifiedBy
protected U lastModifiedBy;
@LastModifiedDate
@Temporal (TIMESTAMP)
protected Date lastModifiedDate;
}
|
This Entitylistner continuously monitor our user when they come into the system.
Step 5: Now, implement the AuditAware Interface (in-built interface).
Java
package org.technous.batchprac2.audit;
import java.util.Optional;
import org.springframework.data.domain.AuditorAware;
public class AuditorAwareImpl implements AuditorAware<String> {
@Override
public Optional<String> getCurrentAuditor() {
return Optional.of( "Geeksforgeek" );
}
}
|
The AuditorAware interface is in-built interface they monitor whom want to modify the system data and save whatever name your provided.
Step 6: Now, set the Spring Boot Main Application.
Java
package org.technous.batchprac2;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.EnableAutoConfiguration;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.annotation.Bean;
import org.springframework.data.domain.AuditorAware;
import org.springframework.data.jpa.repository.config.EnableJpaAuditing;
import org.technous.batchprac2.audit.AuditorAwareImpl;
@EnableJpaAuditing (auditorAwareRef = "auditorAware" )
@SpringBootApplication
public class AditingExampleApplication {
@Bean
public AuditorAware<String> auditorAware() {
return new AuditorAwareImpl();
}
public static void main(String[] args) {
SpringApplication.run(Batchprac2Application. class , args);
}
}
|
In the main application, we need to make a bean like the above. Through this thing, all application in auditing will be saved and also the updates will be saved.
Now, if we run this application, automatically createdBy, createdOn, updatedBy, updatedOn this field fill according to our System Time.
Call the Rest API
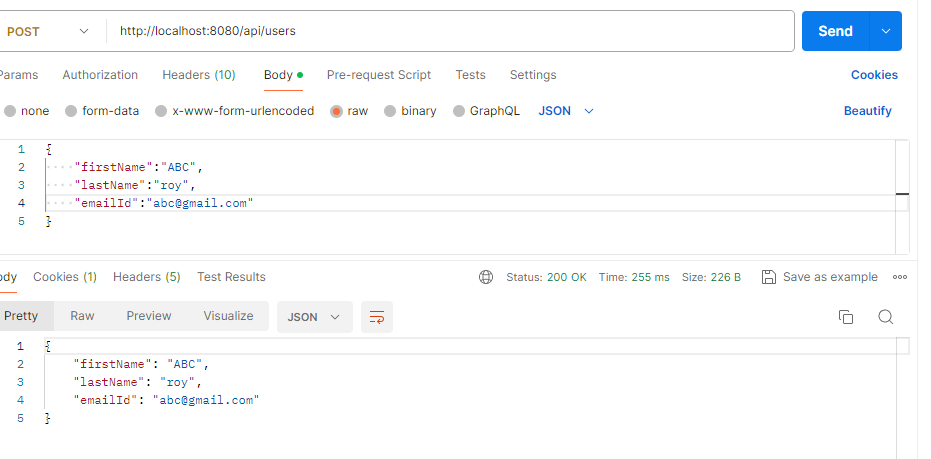
Now, in MySQL Database we can see createdBy, createdOn, updatedBy and updatedOn included.
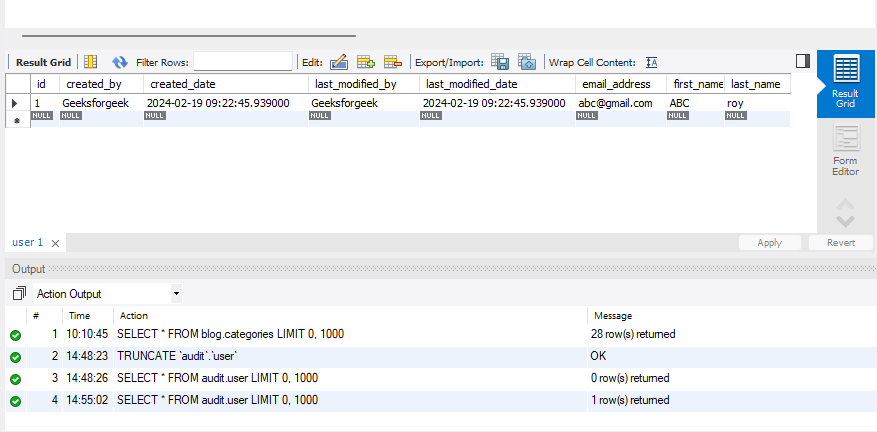
If any user tries to update their data, the lastUpdatedTime will get change. This way we can Audit anything here.
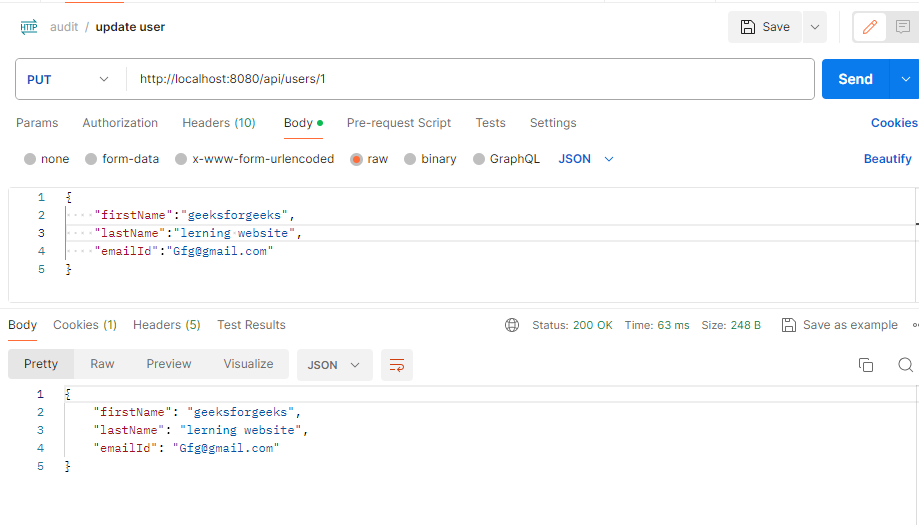
Now, we are updating the user, so automatically lastModified time also updated.

Share your thoughts in the comments
Please Login to comment...