How to check if an array includes an object in JavaScript ?
Last Updated :
30 Nov, 2023
In this article, we will check if an array includes an object or not in JavaScript. There are various methods to check whether an array includes an object or not.
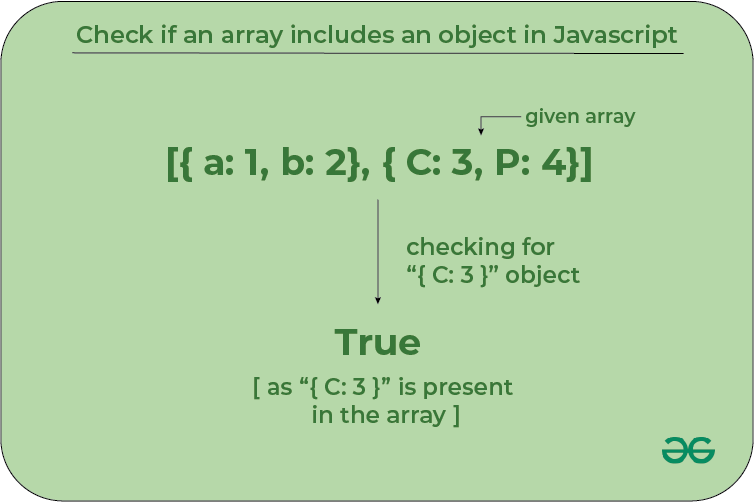
How to check if an array includes an object in JavaScript ?
These are the following approaches by using these we can check if an array includes an object in JavaScript:
If the array contains an object/element can be determined by using the includes() method. This method returns true if the array contains the object/element else return false.
Syntax:Â
array.includes( element/object, startingPosition )
Example: This example shows the use of the above-explained approach.
Javascript
let obj = { "geeks1" : 10, "geeks2" : 12 }
let gfg = [ "geeks1" , "geeks2" , obj];
let num = gfg.includes(obj, 0);
console.log(num)
|
The some() method uses the function for its evaluation and it executes the function once for each element present in the array. If it finds the object/element in the array then it returns true and stops the execution for the remaining elements otherwise returns false.
Syntax:Â Â
array.some( function(currValue, arrIndex, arrObj), this )
Example:Â This example shows the use of the above-explained approach.
Javascript
let arr = [ "geeks1" , "geeks2" , "geeks3" ,
{ 1: "geeks4" , 2: "geeks5" }];
let boolVar = arr.some(
value => { return typeof value == "object" });
console.log(boolVar);
|
The filter() method creates the array of all those elements/objects that pass the checking condition.
Syntax:Â
array.filter( function(currValue, arrIndex, arrObj), this )
Example: This example shows the use of the above-explained approach.
Javascript
let obj = { "geeks1" : 10, "geeks2" : 12 }
let arr = [ "geeks1" , "geeks2" , "geeks3" , obj];
if (arr.filter(value => value == obj).length > 0)
console.log( "true" );
else
console.log( "false" );
|
The findIndex() method returns the position of searched object/element if it is present in the array and stops the execution for the rest elements. If the element/object is not found then return -1.
Syntax:Â
array.findIndex( function(currValue, arrIndex, arrObj), this )
Example: This example shows the use if the above-explained approach.
Javascript
let arr = [ "geeks1" , "geeks2" , "geeks3" ,
{ "geeks1" : 10, "geeks2" : 12 }];
let num = arr.findIndex(
value => { return typeof value == "object" });
console.log( "Object found at position: " + num);
|
Output
Object found at position: 3
we are using _.find() method which helps us to find out that the given value is present in the array or not. and lodash is third party and javascript library.
Syntax:
_.find(collection, predicate, [fromIndex=0])
Example: This example shows the use if the above-explained approach.
Javascript
const _ = require( "lodash" );
let arr = [ "geeks1" , "geeks2" , "geeks3" ,
{ "geeks1" : 10, "geeks2" : 12 }];
let obj = { "geeks1" : 10, "geeks2" : 12 }
console.log(_.find(arr, obj));
|
Output:
{ "geeks1": 10, "geeks2": 12 }
Share your thoughts in the comments
Please Login to comment...