How to add `style=display:“block”` to an element using jQuery?
Last Updated :
18 Dec, 2023
In this article, we will learn how we can add the style=display: “block” inline style to the HTML elements using jQuery. There are multiple approaches to achieve this task as follows:
Using the CSS() method
The css() method of jQuery is used to add the inline styles to the selected element by passing the CSS property and its value as parameters to it.
Syntax:
$('element_selector').css('property_name', 'value');
Example: The below example will illustrate the use of the css() method to add the style=”display: block” to an element using jQuery.
html
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "utf-8" >
< title >
How to add `style=display:“block”`to
an element using jQuery?
</ title >
< style >
p {
color: green;
font-weight: bold;
cursor: pointer;
}
</ style >
< script src =
</ script >
</ head >
< body style = "text-align:center;" >
< h1 style = "color:green;" >
GeeksForGeeks
</ h1 >
< h2 > Using .css()</ h2 >
< div >
< p style = "display: none" >
It is displayed using the
style="display: block"
property added using css() method.
</ p >
< button >click to display</ button >
</ div >
< script >
$('button').click(()=>{
$("p").css("display", "block");
});
</ script >
</ body >
</ html >
|
Output:
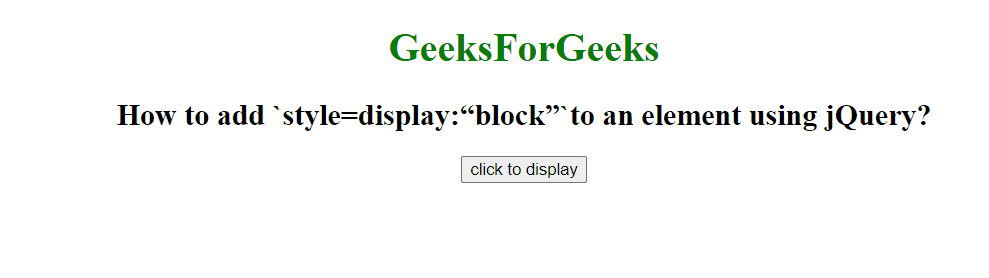
Using the show() method
The show() method can also be used to set the style=”display: block” property to an element.
Syntax:
$('element_selector').show();
Example: The below example will illustrate the use of the show() method to add the style=”display: block” to an element using jQuery.
html
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "utf-8" >
< title >
How to add `style=display:“block”`to
an element using jQuery?
</ title >
< style >
p {
color: green;
font-weight: bold;
cursor: pointer;
}
</ style >
< script src =
</ script >
</ head >
< body style = "text-align:center;" >
< h1 style = "color:green;" >
GeeksForGeeks
</ h1 >
< h2 > How to add `style=display:“block”`to
an element using jQuery? </ h2 >
< div >
< p style = "display: none" >
It is displayed using the
style="display: block"
property added using css() method.
</ p >
< button >click to display</ button >
</ div >
< script >
$('button').click(()=>{
$("p").show();
});
</ script >
</ body >
</ html >
|
Output:
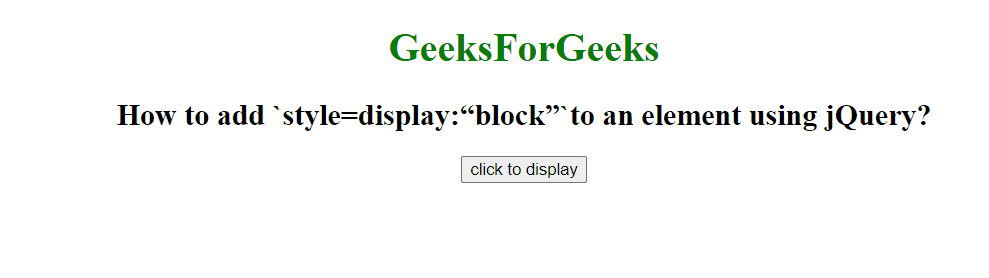
Using the attr() method
The attr() method will also be used to set the style attribute with the passed value.
Syntax:
$('element_selector').attr('attribute', 'value');
Example: The below example will illustrate the use of the attr() method to add the style=”display: block” to an element using jQuery.
html
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "utf-8" >
< title >
How to add `style=display:“block”`to
an element using jQuery?
</ title >
< style >
p {
color: green;
font-weight: bold;
cursor: pointer;
}
</ style >
< script src =
</ script >
</ head >
< body style = "text-align:center;" >
< h1 style = "color:green;" >
GeeksForGeeks
</ h1 >
< h2 > How to add `style=display:“block”`to
an element using jQuery? </ h2 >
< div >
< p style = "display: none" >
It is displayed using the
style="display: block"
property added using css() method.
</ p >
< button >click to display</ button >
</ div >
< script >
$('button').click(()=>{
$("p").attr('style', 'display: block');
});
</ script >
</ body >
</ html >
|
Output:
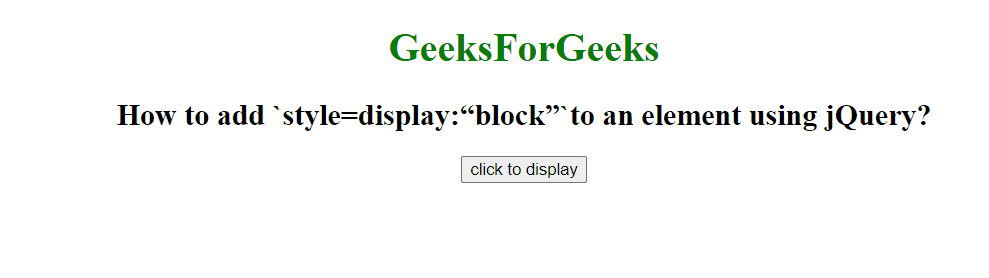
Using the prop() method
The prop() method used to set the style=”display: block” by passing the parameters in the same way as we did in the last example with the attr() method.
Syntax:
$('element_selector').prop('property', 'value');
Example: The below example will illustrate the use of the prop() method to add the style=”display: block” to an element using jQuery.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "utf-8" >
< title >
How to add `style=display:“block”`to
an element using jQuery?
</ title >
< style >
p {
color: green;
font-weight: bold;
cursor: pointer;
}
</ style >
< script src =
</ script >
</ head >
< body style = "text-align:center;" >
< h1 style = "color:green;" >
GeeksForGeeks
</ h1 >
< h2 > How to add `style=display:“block”`to
an element using jQuery? </ h2 >
< div >
< p style = "display: none" >
It is displayed using the
style="display: block"
property added using css() method.
</ p >
< button >click to display</ button >
</ div >
< script >
$('button').click(()=>{
$("p").prop('style', 'display: block');
});
</ script >
</ body >
</ html >
|
Output:
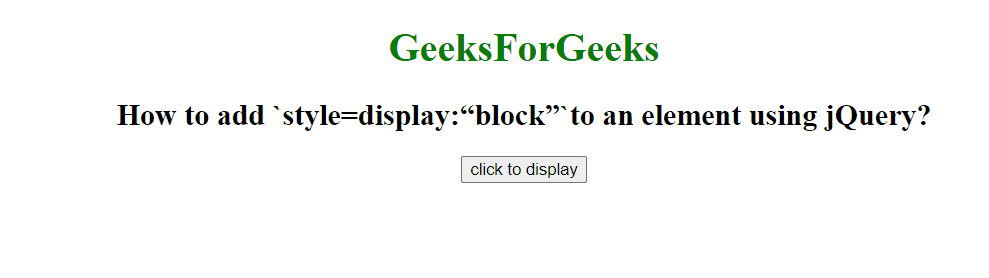
jQuery is an open source JavaScript library that simplifies the interactions between an HTML/CSS document, It is widely famous with it’s philosophy of “Write less, do more”. You can learn jQuery from the ground up by following this jQuery Tutorial and jQuery Examples.
Share your thoughts in the comments
Please Login to comment...