How to Add Headers inside the Dropdown Menu in Bootstrap ?
Last Updated :
17 Apr, 2024
Drop-down menus are a common element used in web development to provide users with a list of options. Adding headers inside a drop-down menu can improve its organization and usability. This means to add headers inside the dropdown menu, use the .dropdown-header class in Bootstrap.
Using HTML and CSS only
To add headers inside the dropdown menu, use the dropdown-header class in Bootstrap. so you can try the following code to implement the dropdown-header class in Bootstrap.
Example: This approach uses HTML utilizes <button> for dropdown activation and <ul> for the menu items. the CSS styles the dropdown to show/hide on hover/focus and positions it below the button.
HTML
<!DOCTYPE html>
<html>
<head>
<title>Bootstrap Example</title>
<link href=
"https://cdn.jsdelivr.net/npm/bootstrap@4.6.0/dist/css/bootstrap.min.css"
rel="stylesheet">
<script src=
"https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js">
</script>
<script src=
"https://cdn.jsdelivr.net/npm/bootstrap@4.6.0/dist/js/bootstrap.bundle.min.js">
</script>
</head>
<body>
<div class="container">
<h2>geeksforgeeks</h2>
<p>The following are the geeksforgeeks dropdown properties:</p>
<div class="dropdown">
<button class="btn btn-primary dropdown-toggle"
type="button"
data-toggle="dropdown">Dropdown
<span class="caret"></span></button>
<ul class="dropdown-menu bg-light">
<li class="dropdown-header text-primary">DSA course</li>
<li><a class="dropdown-item"
href="#">Practice Section</a></li>
<li><a class="dropdown-item"
href="#">Career</a></li>
<li><a class="dropdown-item"
href="#">Menu</a></li>
<div class="dropdown-divider"></div>
<li class="dropdown-header text-primary">Playlist</li>
<li><a class="dropdown-item"
href="#">Interview Preparation</a></li>
<li><a class="dropdown-item"
href="#">STL Concept</a></li>
</ul>
</div>
</div>
</body>
</html>
Output:
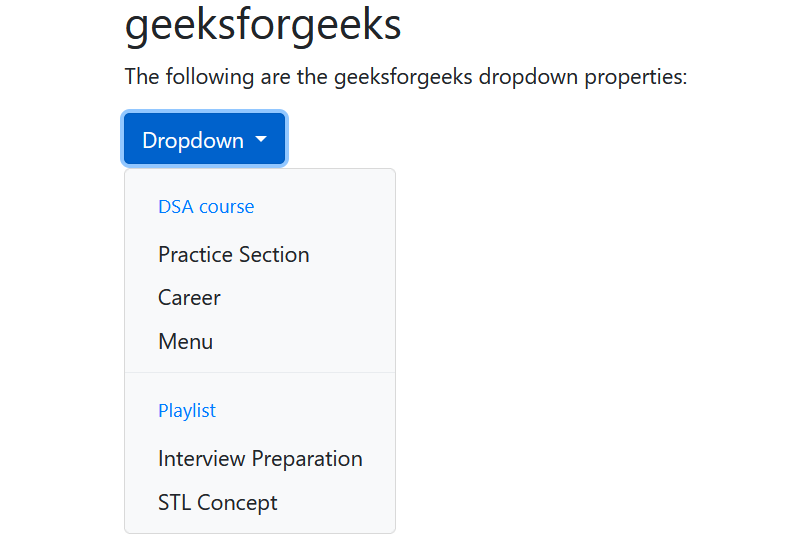
Utilizing JavaScript and jQuery
This method involves using JavaScript and jQuery to dynamically generate drop-down menu items with headers.but JavaScript can manipulate the DOM to insert header elements among the drop-down items.It allows for flexible and dynamic manipulation of dropdown content based on user interactions or data changes.
Example: It dynamically appends items and headers to the dropdown menu using jQuery’s append() method, creating distinct sections.Headers are represented by <div class=”header”> elements, while menu items are <a class=”dropdown-item”> elements.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>Dropdown with Headers - JavaScript/jQuery</title>
<!-- Bootstrap CSS -->
<link href=
"https://cdn.jsdelivr.net/npm/bootstrap@4.6.0/dist/css/bootstrap.min.css"
rel="stylesheet">
</head>
<body>
<h1> geeksforgeeks inside headers</h1>
<div class="dropdown">
<button class="btn btn-primary dropdown-toggle"
type="button"
id="dropdownMenuButton"
data-toggle="dropdown"
aria-haspopup="true"
aria-expanded="false">
Dropdown
</button>
<div class="dropdown-menu"
aria-labelledby="dropdownMenuButton">
</div>
</div>
<!-- Bootstrap JS -->
<script src=
"https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script src=
"https://cdn.jsdelivr.net/npm/bootstrap@4.6.0/dist/js/bootstrap.bundle.min.js"></script>
<script>
$(document).ready(function () {
// Code for the first dropdown menu
$('.dropdown-menu')
.append('<div class="dropdown-header bg-primary text-white">Header 1</div>');
$('.dropdown-menu')
.append('<a class="dropdown-item item" href="#">DSA course</a>');
$('.dropdown-menu')
.append('<a class="dropdown-item item" href="#">practice section</a>');
$('.dropdown-menu')
.append('<div class="dropdown-header bg-primary text-white">Header 2</div>');
$('.dropdown-menu')
.append('<a class="dropdown-item item" href="#">career</a>');
$('.dropdown-menu')
.append('<a class="dropdown-item item" href="#">Action 4</a>');
});
</script>
</body>
</html>
Output:
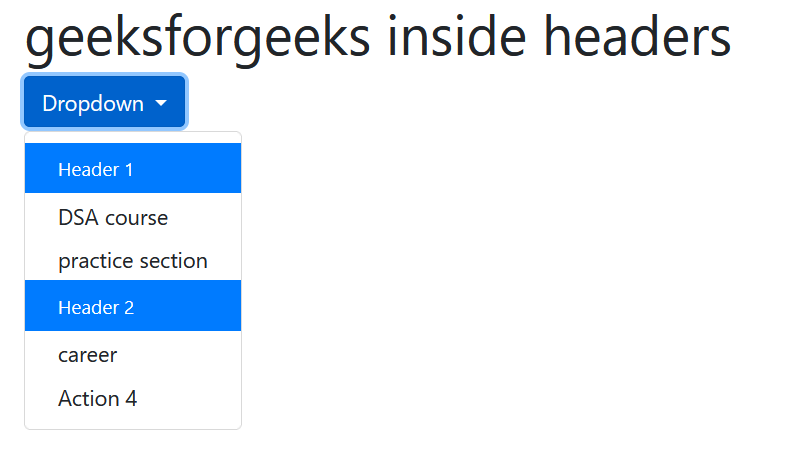
Using Bootstrap 5’s dropdown group component
With Bootstrap 5, you can use the dropdown group component to create dropdown menus with headers. This approach is more streamlined as it utilizes Bootstrap’s built-in functionality. The code defines a dropdown menu with headers and menu items using Bootstrap’s dropdown group classes, making it easy to create distinct sections within the dropdown.
For Example: “Bootstrap’s dropdown menu utilizes classes such as dropdown and dropdown-menu, with headers designated by <h6 class=”dropdown-header”>. Menu items are <a class=”dropdown-item”>, and section dividers are <hr class=”dropdown-divider”>.”
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Dropdown with Headers - Bootstrap 5</title>
<!-- Bootstrap CSS -->
<link href=
"https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha1/dist/css/bootstrap.min.css"
rel="stylesheet">
</head>
<body>
<br>
<h2>Geeksforgeek inside headers</h2>
<div class="dropdown">
<button class="btn btn-secondary dropdown-toggle"
type="button"
id="dropdownMenuButton"
data-bs-toggle="dropdown"
aria-expanded="false">
Dropdown
</button>
<ul class="dropdown-menu"
aria-labelledby="dropdownMenuButton">
<li>
<h6 class="dropdown-header">Header 1</h6>
</li>
<li><a class="dropdown-item"
href="#">Action 1</a></li>
<li><a class="dropdown-item"
href="#">Action 2</a></li>
<li>
<hr class="dropdown-divider">
</li>
<li>
<h6 class="dropdown-header">Header 2</h6>
</li>
<li><a class="dropdown-item"
href="#">Action 3</a></li>
<li><a class="dropdown-item"
href="#">Action 4</a></li>
</ul>
</div>
<!-- Bootstrap JS -->
<script src=
"https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha1/dist/js/bootstrap.bundle.min.js"></script>
</body>
</html>
Output:
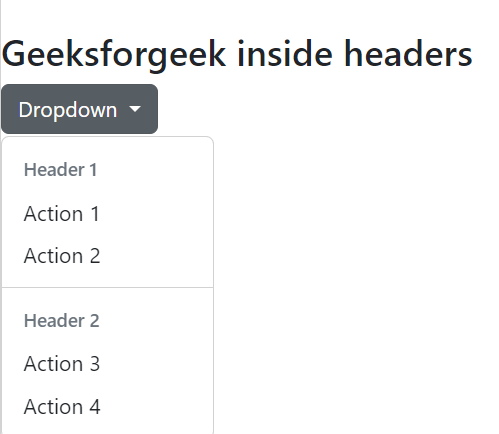
Share your thoughts in the comments
Please Login to comment...