Don’t repeat yourself(DRY) in Software Development
Last Updated :
22 Feb, 2024
“Don’t Repeat Yourself” (DRY) is a software development principle that encourages developers to avoid duplicating code in a system. The main idea behind DRY is to reduce redundancy and promote efficiency by ensuring that a particular piece of knowledge or logic exists in only one place within a codebase. When developers adhere to the DRY principle, they aim to create reusable components, functions, or modules that can be utilized in various parts of the codebase. This not only makes the code more maintainable but also minimizes the chances of errors since changes or updates only need to be made in one location.
DRY is closely related to the concept of modular programming and the creation of functions, classes, or modules that encapsulate specific functionality. By following DRY, developers can enhance code readability, decrease the likelihood of bugs caused by inconsistent updates, and facilitate easier maintenance and collaboration within a development team. An alternative principle, the “Single Responsibility Principle” (SRP), is often mentioned in conjunction with DRY. SRP suggests that a module, class, or function should have only one reason to change, further emphasizing the need for focused, modular, and reusable code. Together, DRY and SRP contribute to creating more robust and maintainable software systems.
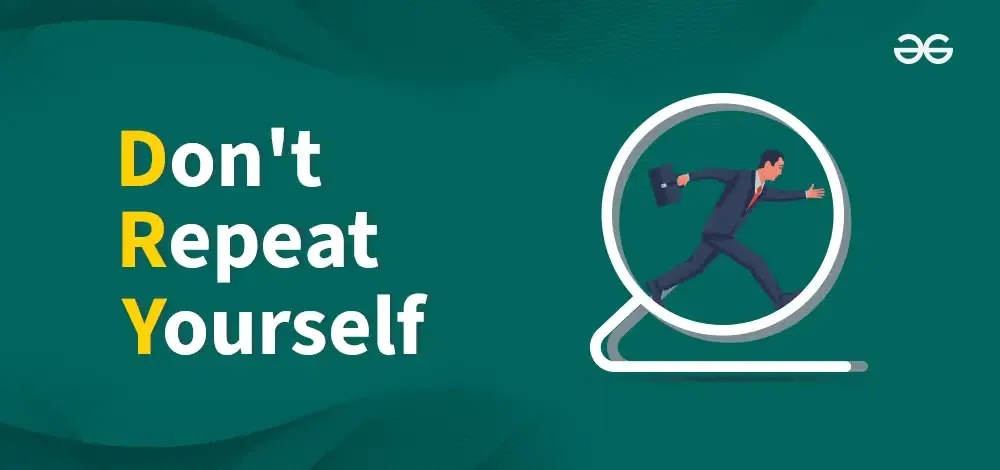
Don’t Repeat Yourself (DRY)
Key features of the “Don’t Repeat Yourself” (DRY)
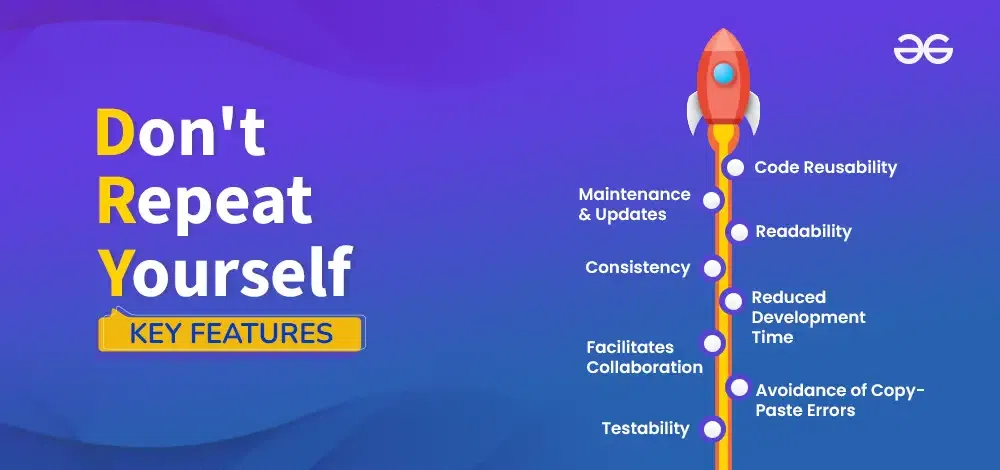
Key Features of DRY
The key features of the “Don’t Repeat Yourself” (DRY) principle in software development include:
- Code Reusability: DRY encourages developers to write code in a way that minimizes redundancy. Instead of duplicating code, developers should create reusable components, functions, or modules that can be shared and applied in multiple parts of the codebase.
- Maintenance and Updates: By adhering to DRY, developers reduce the likelihood of errors and bugs that can arise from inconsistent updates. Since a particular piece of logic or knowledge exists in only one place, any changes or enhancements can be made in a centralized location, making maintenance more efficient.
- Readability: DRY contributes to code readability by eliminating unnecessary repetition. When developers follow the principle, it becomes easier for others (or even themselves) to understand and navigate the codebase since there are fewer instances of similar or identical code scattered throughout.
- Consistency: DRY promotes consistency in the codebase. When a specific functionality is encapsulated in a single location, it ensures that all instances of that functionality behave consistently. This is crucial for creating reliable and predictable software.
- Reduced Development Time: By reusing code instead of rewriting it, developers can significantly reduce the time and effort required for development. This is particularly valuable when building large and complex software systems, as it streamlines the development process.
- Facilitates Collaboration: DRY enhances collaboration among team members. When code is modular and reusable, it becomes easier for multiple developers to work on different parts of the system without interfering with each other. Each developer can focus on a specific component or module without duplicating efforts.
- Avoidance of Copy-Paste Errors: Copying and pasting code can introduce errors, especially if changes are made inconsistently across duplicated sections. DRY minimizes the need for copy-pasting by encouraging the creation of reusable units of code, reducing the risk of introducing errors.
- Testability: Reusable and modular code is often easier to test since specific functionalities are encapsulated in distinct units. This makes it simpler to write unit tests and ensure that changes do not inadvertently affect unrelated parts of the system.
Why Write DRY Code:
- Maintainability: DRY code is easier to maintain because changes or updates only need to be made in one place, reducing the risk of inconsistencies.
- Readability: DRY promotes cleaner and more readable code by eliminating unnecessary repetition, making it easier for developers to understand the logic.
- Efficiency: Reusing existing code instead of duplicating it saves development time and effort, resulting in more efficient and productive workflows.
- Consistency: DRY ensures consistent behavior throughout the codebase, as a specific piece of logic exists in only one place.
- Reduced Bugs: DRY reduces the chances of introducing bugs or errors caused by inconsistent changes in duplicated code.
Approaches to Resolving Duplication by DRY:
- Create Functions or Methods: Identify repeated logic and encapsulate it in functions or methods that can be called from multiple locations.
- Use Classes and Inheritance: For more complex scenarios, use classes and inheritance to create reusable components that share common functionality.
- Extract Constants or Configurations: If certain constants or configurations are repeated, centralize them to a single source to avoid redundancy.
- Modularization: Break down the code into modular components, each responsible for a specific task. This promotes reusability and modularity.
Aspect |
DRY (Don’t Repeat Yourself) |
WET (Write Everything Twice) |
AHA (Avoid Hasty Abstractions) |
Definition |
Promotes code reuse and avoids redundancy. |
Implies repetitive code, where similar logic is duplicated. |
Warns against premature abstractions that might be unnecessary. |
Goal |
Eliminate code duplication for improved maintainability. |
Indicates redundancy and the need for refactoring. |
Discourages unnecessary abstraction, focusing on simplicity. |
Focus |
Reusable components, functions, or modules. |
Repetitive code, indicating potential issues. |
Avoiding unnecessary abstraction and complexity. |
Outcome |
Clean, maintainable, and efficient code. |
Code smells and potential maintenance challenges. |
Balanced, straightforward solutions without premature abstractions. |
Example of DRY:
Consider a scenario where the same validation logic for email addresses is required in multiple parts of your code:
Python3
def validate_email_format_1(email):
if "@" in email and "." in email:
return True
else :
return False
def validate_email_format_2(email):
if "@" in email and "." in email:
return True
else :
return False
def validate_email_format(email):
if "@" in email and "." in email:
return True
else :
return False
|
In the non-DRY example, the same validation logic is repeated in multiple functions. With DRY, the validation logic is encapsulated in a single function, promoting code reuse and reducing redundancy
“Don’t Repeat Yourself” (DRY) is one of several software development principles that guide developers in writing high-quality, maintainable, and efficient code. While DRY emphasizes the avoidance of code duplication, there are other principles that focus on different aspects of software design and development. Here’s how DRY differs from some other prominent software development principles:
- Single Responsibility Principle (SRP):
- DRY: DRY focuses on code duplication and encourages the reuse of code to avoid redundancy.
- SRP: SRP states that a class or module should have only one reason to change, emphasizing that each component should have a single responsibility. This encourages modularity and helps in building maintainable and adaptable systems.
- Open/Closed Principle (OCP):
- DRY: DRY aims to eliminate duplicated code by promoting the creation of reusable components.
- OCP: OCP suggests that software entities (classes, modules, functions) should be open for extension but closed for modification. It encourages the use of abstraction and interfaces to allow for adding new functionality without modifying existing code.
- Liskov Substitution Principle (LSP):
- DRY: DRY deals with code duplication and redundancy.
- LSP: LSP emphasizes that objects of a superclass should be replaceable with objects of a subclass without affecting the correctness of the program. It focuses on maintaining the expected behavior of a program when using polymorphism.
- Interface Segregation Principle (ISP):
- DRY: DRY is concerned with avoiding code duplication and promoting code reuse.
- ISP: ISP states that a class should not be forced to implement interfaces it does not use. It promotes the creation of specific interfaces for clients to implement, avoiding unnecessary dependencies.
- Dependency Inversion Principle (DIP):
- DRY: DRY addresses code duplication and encourages the creation of reusable components.
- DIP: DIP suggests that high-level modules should not depend on low-level modules, but both should depend on abstractions. It promotes the use of dependency injection and inversion of control to achieve flexibility and maintainability.
- KISS (Keep It Simple, Stupid):
- DRY: DRY focuses on reducing redundancy and avoiding unnecessary repetition.
- KISS: KISS encourages simplicity in design and implementation. It suggests that systems and designs should be as simple as possible to achieve their goals, avoiding unnecessary complexity.
- YAGNI (You Ain’t Gonna Need It):
- DRY: DRY is concerned with eliminating code duplication.
- YAGNI: YAGNI advises against adding features or functionality until they are actually needed. It discourages over-engineering and unnecessary complexity in anticipation of potential future requirements.
In summary, DRY is just one of several principles that collectively contribute to the development of high-quality, maintainable, and scalable software. Each principle addresses different aspects of software design and encourages best practices in various areas of development. It’s common for developers to consider and apply multiple principles in conjunction to create robust and well-designed software systems.
“Don’t Repeat Yourself” (DRY) Vs “Single Responsibility Principle” (SRP)
“Don’t Repeat Yourself” (DRY) and “Single Responsibility Principle” (SRP) are two distinct software development principles, each addressing different aspects of code quality and maintainability. Let’s explore the key differences between DRY and SRP:
DRY
|
SRP
|
DRY is primarily concerned with eliminating redundancy in code. It advocates for the reuse of code to avoid duplicating the same logic or information in multiple places within a codebase.
|
SRP is focused on the concept of a class or module having a single responsibility. It encourages designing classes or modules that do one thing and do it well, promoting a separation of concerns.
|
DRY addresses the issue of code duplication and aims to improve maintainability and readability by avoiding repetition of logic or information.
|
SRP addresses the issue of code organization and design by advocating for classes or modules with a clear and singular responsibility. It aims to enhance modularity and maintainability by avoiding classes that do too much.
|
DRY focuses on the redundancy of code within a codebase. It aims to minimize the repetition of code snippets, functions, or logic to avoid inconsistencies and make maintenance more straightforward.
|
SRP focuses on the responsibilities of classes or modules. It suggests that a class should have only one reason to change, meaning it should encapsulate a single responsibility or concern.
|
DRY operates at a more granular level, dealing with specific lines of code, functions, or modules that may be duplicated.
|
SRP operates at a higher level of abstraction, focusing on the design of classes or modules and their responsibilities within the overall architecture.
|
DRY is more about implementation details, suggesting ways to organize and structure code to avoid redundancy.
|
SRP is more about design principles and how classes or modules should be structured to achieve clarity, maintainability, and adaptability.
|
DRY is less concerned with abstraction and more focused on the concrete implementation of code and its reuse.
|
SRP is closely related to the use of abstraction and interfaces, as it encourages designing classes or modules with well-defined responsibilities and interactions.
|
In practice, DRY and SRP are often used together to create clean, maintainable, and efficient code. While DRY addresses the need for code reuse and elimination of redundancy, SRP focuses on the higher-level design principles that contribute to modular, well-organized code. Applying both principles contributes to the development of robust and maintainable software systems
Advantages and Disadvantages of “Don’t Repeat Yourself” (DRY)
Advantages of DRY:
- Code Maintainability: DRY promotes code maintainability by reducing redundancy. Updates and changes can be made in one place, making it easier to manage and maintain the codebase.
- Readability: DRY enhances code readability by eliminating unnecessary duplication. This makes it easier for developers to understand the code and reduces the likelihood of errors caused by inconsistent variations of the same logic.
- Consistency: DRY ensures consistency in the behavior of code since a particular piece of logic or knowledge exists in only one place. This consistency contributes to a more reliable and predictable software system.
- Code Reusability: DRY encourages the creation of reusable components, functions, or modules. This reusability reduces development time and effort, as developers can leverage existing code instead of writing the same logic from scratch.
- Facilitates Collaboration: DRY facilitates collaboration within a development team. Code that follows DRY principles is modular and can be worked on independently by different team members, leading to more efficient teamwork.
- Reduced Errors: DRY reduces the likelihood of errors caused by inconsistent updates. Since changes are made in one location, there is less chance of overlooking or forgetting to update duplicated code.
Disadvantages and Challenges of DRY:
- Over-Abstraction: Striving for excessive reusability can lead to over-abstraction, making the code overly complex. Developers need to strike a balance between reusability and simplicity to avoid introducing unnecessary complications.
- Premature Optimization: Focusing too much on DRY principles in the early stages of development might lead to premature optimization. Developers should prioritize clarity and simplicity initially and refactor for reusability when patterns emerge.
- Learning Curve: Enforcing DRY can have a learning curve for developers who are new to the codebase. Understanding how and where to create reusable components may take time, especially in complex systems.
- Dependency Challenges: Overly tight coupling between components can arise when enforcing DRY too rigorously. Dependencies between modules may increase, making it challenging to modify one part without affecting others.
- Code Bloat: In some cases, efforts to avoid duplication might lead to code bloat, especially if creating overly generic or complex abstractions. This can negatively impact performance and increase the cognitive load on developers.
- Context-Specific Cases: There are scenarios where adhering strictly to DRY may not be suitable, such as when dealing with context-specific variations. In such cases, a balance needs to be struck between DRY and accommodating specific requirements.
- Tooling and Infrastructure: Implementing and maintaining DRY may require additional tooling and infrastructure to manage reusable components effectively. This can add complexity to the development process.
Conclusion: Don’t repeat yourself
In conclusion, the “Don’t Repeat Yourself” (DRY) approach promotes readability, maintainability, and code efficiency and is a fundamental guideline in software development. DRY lowers the chance of errors, improves collaboration, and facilitates maintenance by encouraging code reuse and decreasing redundancy. The advantages of DRY must be weighed against any potential drawbacks, such as dependency problems, over-abstraction, and premature optimisation. In the end, using DRY in conjunction with other complimentary concepts encourages the development of reliable, scalable, and maintainable software systems. Developers are contributing to a culture of excellence and efficiency in software engineering as they continue to perfect their processes and embrace DRY.
FAQs On Don’t repeat yourself
What is the DRY and wet principle?
- DRY: “Don’t Repeat Yourself” principle advocates for avoiding code duplication by creating reusable components.
- WET: “Write Everything Twice” (or “We Enjoy Typing”) indicates redundant code, where similar logic is repeated, leading to maintenance challenges.
What is the opposite of don’t repeat yourself
The opposite of DRY is WET, where code redundancy is embraced rather than avoided.
What is an example of a DRY principle?
Creating a function for email validation that is used in multiple parts of a codebase instead of repeating the validation logic in each location.
What is the KISS design principle?
KISS stands for “Keep It Simple, Stupid” and advocates for simplicity in design and development, prioritizing straightforward solutions over unnecessary complexity.
Share your thoughts in the comments
Please Login to comment...