Difference between @RequestBody and @RequestParam
Last Updated :
23 Apr, 2024
In Spring Boot, the @RequestBody is used for binding the HTTP request body to a parameter in a controller method and it is typically used when we expect the client to send data in the request body when submitting a form or sending JSON data. The @RequestParam is one of the powerful full annotations in Spring Boot. This annotation can enable the Spring Boot framework to extract input data passed through an HTTP URL or passed as a query.
In this article, we will learn about @RequestBody vs @RequestParam annotation.
@RequestBody
The @RequestBody is used to bind the HTTP request body to a parameter in the controller method and is typically used when we expect the client to send data in the request body, such as a form to send or JSON data to send.
- The @RequestBody annotation is used with POST, PUT, and PATCH requests where data is sent in the request body.
- In Spring Boot, an HTTP Request is automatically deserialized into a Java object.
- The @RequestBody annotation translates the HTTP Request body into a domain object.
The Request body look like a below syntax. And The request body is plays an important role in data transform from Client to Server.
{
"username":"John",
"age":"21"
}
Example:
- First, we created a Spring Boot project with the required dependencies.
- Then, in the main package of the project, we created one Java class for the domain object.
- Additionally, we created another Java class for handling API endpoints using RestController.
- The @RestController annotation is used in Spring Boot to create Rest APIs.
Below, we provide an API URL for testing @RequestBody in Spring Boot. This API has a POST type.
http://localhost:8080/user
User.class:
Java
package com.app;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
@AllArgsConstructor
@NoArgsConstructor
@Data
public class User {
private String username;
private int age;
}
- The above code defines a Java class called User which has two fields ie. username and age.
- The class is defined by the Lombok annotations @AllArgsConstructor, @NoArgsConstructor, and @Data, which automatically provide getter/setter methods for all arguments, no-argument constructors, and serialized fields
- This simplifies the boilerplate code and increases readability.
MyHandler.class:
Java
package com.app;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class MyController {
// Endpoint to handle POST requests for creating a user
@PostMapping("/user")
public ResponseEntity<String> createUser(@RequestBody User user) {
// Logic to create a user and return response
return ResponseEntity.ok("User created: " + user.toString());
}
}
- Here, a method defined by @PostMapping, which means it handles HTTP POST requests to the “/user” endpoint.
- The method treats the User object as a request body using the @RequestBody annotation, creates the user, and returns a ResponseEntity with a success message.
Output:
Once the logic is developed, we run this project as a Spring Boot App. Now, we open Postman to test the API endpoint. Upon testing, we received the following output.
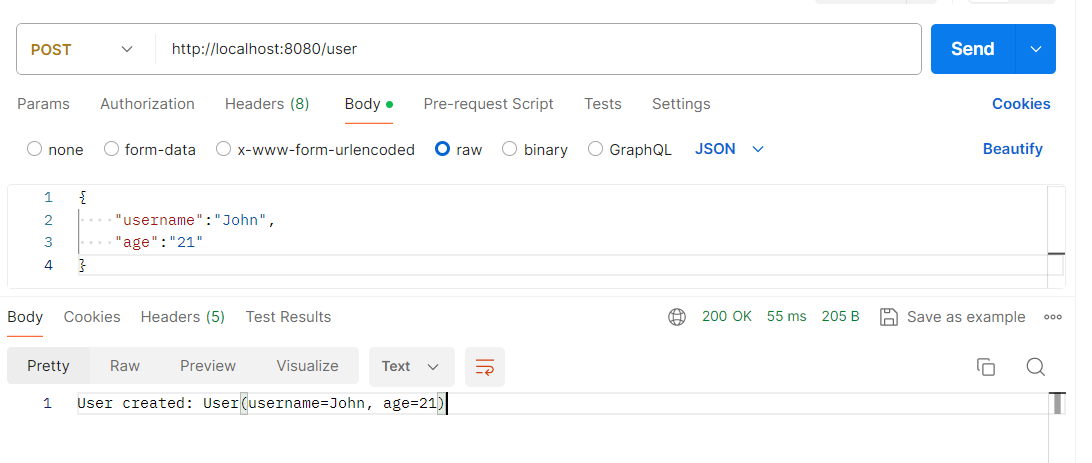
@RequestParam
- The @RequestParam is one of the powerful annotation in Spring Boot.
- This annotation enables the Spring Boot framework to extract input data passed through the HTTP URL or passed as a query.
- Additionally, when a parameter is not specified, the method parameter can be bound to null.
- Below, we provide a simple example along with a related output image.
Example:
Now, we created one more API in the MyController class called “users”.
- For this API, we created one method called “getUser”, which takes one argument value as input, namely the user ID value, using the @RequestParam annotation in Spring Boot.
- Now, open Postman and hit the API to get the output.
- Below, we provide an image of the output.
- Additionally, we used the same domain object for this API, and this API supports only the GET method.
http://localhost:8080/users?id=1
Java
package com.app;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class MyController {
// Define a method to handle GET requests to the "/users" endpoint
@GetMapping("/users")
// Method to retrieve user details based on the provided user ID parameter
public ResponseEntity<String> getUser(@RequestParam("id") Long userId) {
// Return a ResponseEntity with the user details for the specified user ID
return ResponseEntity.ok("User details for ID " + userId);
}
}
- The above code defines a Spring REST controller named
MyController
. - It contains a method
getUser()
annotated with @GetMapping
to handle GET requests to the “/users” endpoint. - The method takes a
userId
parameter annotated with @RequestParam
to retrieve user details based on the provided user ID. - Finally, it returns a ResponseEntity with the user details for the specified user ID.
Output:
Once the logic is developed, we run this project as a Spring Boot App. Now, we open Postman to test the API endpoint. Upon testing, we received the following output.
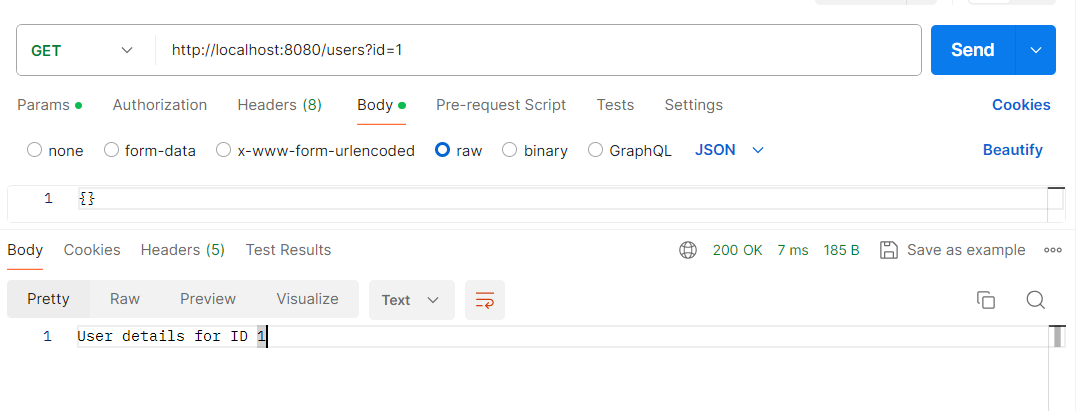
Difference between @RequestBody and @RequestParam
Below, we have provided the difference between @RequestBody and @RequestParam in the Spring Boot framework.
Feature
| @RequestBody
| @RequestParam
|
---|
Usage
| It is used to bind the HTTP request body to the parameter in the controller method.
| It is used to extract parameters from the query string or form data of an HTTP request.
|
---|
HTTP Method
| Used with POST, PUT, and PATCH requests where data is sent in the request body.
| Used with GET, POST, and other types of requests where parameters are sent in the URL.
|
---|
Data Type
| Suitable for complex data types such as objects or lists.
| Used for simple data types such as strings or numbers.
|
---|
Request Format
| Expects data in JSON, XML, or other structured formats in the request body.
| Expects parameters in the query string or form data format.
|
---|
Example
| @PostMapping(“/users”)
| @GetMapping(“/users”)
|
---|
Share your thoughts in the comments
Please Login to comment...