strcat() Function in C++
Last Updated :
04 Apr, 2024
The strcat() function in C++ is a predefined function in the <cstring> header file that is used to concatenate two strings, by appending a copy of the source string to the end of the destination string.
This function works by adding all the characters till the null character of the source string at the position where the null character is present in the destination string. Due to this, strcat() can only be used on null-terminated strings (Old C-Style Strings).
Syntax of strcat() in C++
char *strcat(char *destination, const char *source);
Parameters of strcat() in C++
- destination: It is a pointer to the destination string. It should be large enough to store the concatenated string.
- source: It is a pointer to the source string which is appended to the destination string.
Return Value of strcat() in C++
The strcat() function returns a pointer to the destination string.
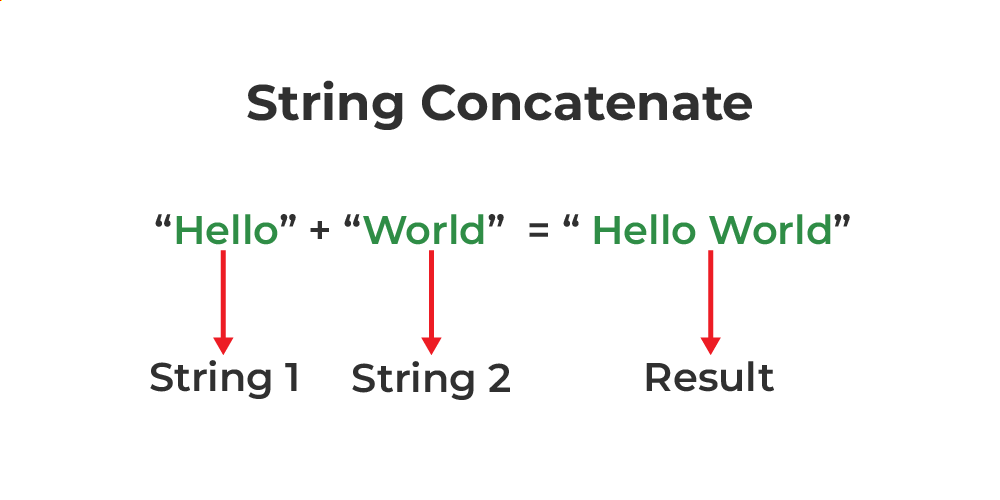
String Concatenation in C++
Example to Use strcat() in C++
Input:
src = "GeeksforGeeks is an"
dest = "Online Learning Platform"
Output:
GeeksforGeeks is an Online Learning Platform
The below example illustrates how we can use strcat() function to concatenate or append two strings in C++.
C++
// C++ Program to use strcat() function to concatenate two
// strings in C++
#include <cstring>
#include <iostream>
using namespace std;
int main()
{
// C-style destination string
char dest[50] = "GeeksforGeeks is an";
// C-style source string
char src[50] = " Online Learning Platform";
// concatenating both strings
strcat(dest, src);
// printing the concatenated string
cout << dest;
return 0;
}
OutputGeeksforGeeks is an Online Learning Platform
Note: The behavior of strcat() is undefined if the destination array is not large enough to store the contents of both source and destination string, or if the strings overlap.
There is also a function similar to strcat() which only appends the given number of characters from the source string to the destination string. It is strncat().
Share your thoughts in the comments
Please Login to comment...