C++23 Library – <spanstream> Header
Last Updated :
06 May, 2023
The <spanstream> header is a new addition to C++ 23 Standard Libraries Collection. It provides fixed character buffer streams for input and output.
It is a collection of classes and function templates that let you manipulate letter stretch as if they were streams, much like <stringstream> or <istringstream>. However, it works with std::span<char> rather than pulling from or writing to a string or buffer.
The following image illustrates the inheritance diagram of the <spanstream> header file:
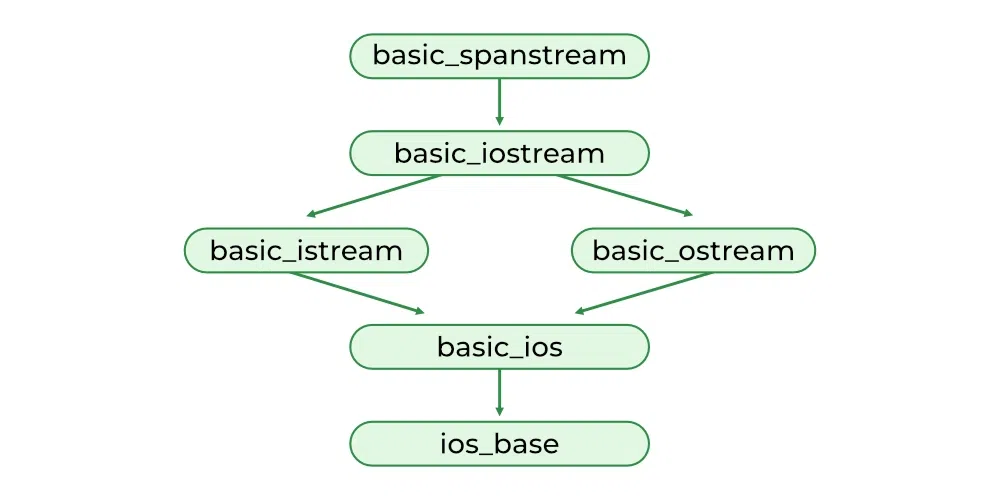
Class Templates in <spanstream>
Following is the list of classes defined inside the <spanstream> header file:
- basic_spanbuf
- basic_ispanstream
- basic_ospanstream
- basic_spanstream
- spanbuf
- wspanbuf
- ispanstream
- wispanstream
- spanstream
- wspanstream
All these classes have their own member functions and work.
std::spanstream
The std:;spanstream is a typedef name defined for basic_spanstream<char> that is nothing but a span-based string I/O buffer. We can initialize the objects of this class using std::span objects and then we can use it like a separate string buffer for input and output.
Example of <spanstream>
The following C Program demonstrates the use of spanstream class:
C++
#include <iostream>
#include <spanstream>
int main()
{
std::string data = "3.45 5.67 4.64" ;
std::span< char > inSpan(data);
std::spanstream inSpanStream(inSpan);
double geeks_double1, geeks_double2, geeks_double3;
inSpanStream >> geeks_double1 >> geeks_double2
>> geeks_double3;
std::cout << "geeks_double1 = " << geeks_double1
<< "\n" ;
std::cout << "geeks_double2 = " << geeks_double2
<< "\n" ;
std::cout << "geeks_double3 = " << geeks_double3
<< "\n" ;
std::string data_t = "This is a test" ;
std::span< char > outSpan(data_t);
std::spanstream outSpanstream(outSpan);
outSpanstream << "GeeksforGeeks " ;
std::cout << "Result: " << outSpanstream.rdbuf()
<< "\n" ;
return 0;
}
|
Output
geeks_double1 = 3.45
geeks_double2 = 5.67
geeks_double3 = 4.64
Result: GeeksforGeeks
In the above example, we have used spanstream case to perform both input and output using >> and << operators.
Note: The above code can only be run on the compilers with C++ 23 standard support such as GCC 12 and MSVC 19.31 and onwards.
Share your thoughts in the comments
Please Login to comment...