Bootstrap 5 Modal Via JavaScript
Last Updated :
06 May, 2024
Bootstrap 5 modal via JavaScript allows for the dynamic creation of models using JavaScript. Utilize Bootstrap’s modal API to trigger modal display, content loading, and event handling for interactive and responsive user experiences.
Prerequisite:
Bootstrap 5 Modal Via JavaScript Classes & Methods: To use the class you need to have knowledge about Bootstrap 5 Modal, and Bootstrap 5 Modal Usage Methods.
Syntax:
var myModal = new bootstrap.Modal(document.getElementById('modal-id'));
Example 1: In this example, we are initializing the Modal using JavaScript and using its toggle() method to toggle its visibility.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible"
content="IE=edge">
<meta name="viewport"
content="width=device-width,
initial-scale=1.0">
<link href=
"https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"
rel="stylesheet">
<script src=
"https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.min.js">
</script>
</head>
<body>
<div class="container">
<div>
<h5>Bootstrap 5 Modal Via JavaScript</h5>
</div>
<button class="btn btn-success" onclick="toggleModal1()">
Toggle Modal
</button>
<div id="gfg" class="modal" tabindex="-1">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title">
GeeksforGeeks
</h5>
</div>
<div class="modal-body">
<p>
Bootstrap 5 is the newest version
of bootstrap it is a free open
source front-end development framework
</p>
</div>
</div>
</div>
</div>
</div>
<script>
var modal1 = new bootstrap.Modal(document.getElementById('gfg'));
function toggleModal1() {
// Toggle Modal
modal1.toggle();
// Toggle the modal again after 3 seconds
setTimeout(() => {
modal1.toggle();
}, 3000)
}
</script>
</body>
</html>
Output:
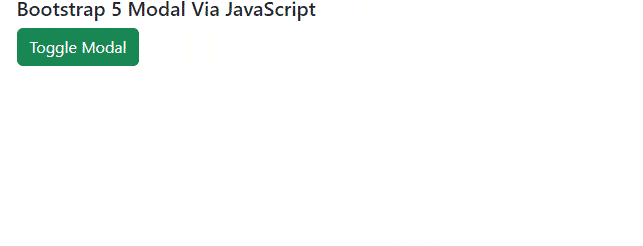
Bootstrap Modal Via JavaScript Example Output
Example 2: In this example, the modal is initialized and the visibility is changed using its show() and hide() methods via JavaScript.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible"
content="IE=edge">
<meta name="viewport"
content="width=device-width,
initial-scale=1.0">
<link href=
"https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"
rel="stylesheet">
<script src=
"https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.min.js">
</script>
</head>
<body>
<div class="container">
<div class="header">
<h3 class="text-success">GeeksforGeeks</h3>
<h5>Bootstrap 5 Modal Via JavaScript</h5>
</div>
<button class="btn btn-success" onclick="showhide()">
Show and after 5 seconds Hide the Modal
</button>
<div id="gfg" class="modal" tabindex="-1">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title">
GeeksforGeeks
</h5>
</div>
<div class="modal-body">
<p>
it is a dialog box/popup window
that is displayed
on top of the current page.
</p>
</div>
</div>
</div>
</div>
</div>
<script>
var modal1 = new bootstrap.Modal(document.getElementById('gfg'));
function showhide() {
// show Modal
modal1.show();
// hide the modal after 5 seconds
setTimeout(() => {
modal1.hide();
}, 5000)
}
</script>
</body>
</html>
Output:
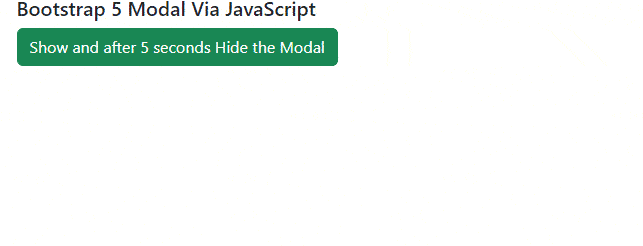
Bootstrap 5 Modal Via JavaScript Example Output
Share your thoughts in the comments
Please Login to comment...