Spring WebFlux is a responsive framework in the Spring ecosystem and is designed to handle asynchronous, non-blocking, and event-driven web applications in Java programming It can support responsive web applications of size and resources use the minimum function -allowing you to control interactions. In this article, we will explain about then, thenEmpty, thenMany, and flatMapMany in Spring WebFlux. These methods are used to manage the execution of the flow in the reactive programming language. Commonly these methods are used with Mono or Flux publishers which represents asynchronous and non-blocking.
Now, we will learn every method with related examples for better understanding. Below I listed those methods with proper explanation and examples.
Prerequisites:
To understand this article, we need to have some knowledge of the topics mentioned below:
- Basics of Java Programming
- Project creation for Spring reactive framework
- Spring WebFlux
- Spring Reactive Framework
- Working process of Mono and Flux publishers
then() method
The then method is used to execute a subsequent operation after completion of the current Mono or Flux publishers. And This returns a new Mono that completes when both the resources and the other Mono or Flux publishers.
Below, we have provided the related example for reference:
import reactor.core.publisher.Mono;
public class SpringReactiveMethods {
public static void main(String[] args) {
Mono.just("Hello")
.then(Mono.just("World"))
.subscribe(System.out::println);
}
}
Output:
Below we can refer to the console output.

Explanation of the above Program:
- Here, first we have created one Java Class, then in main method, we wrote the logic for then() method.
- In the above example, we have used Mono.just method for taking the String value that is Hello.
- After this, we called then() method after Mono.just() method.
- Next, in .then() method, again we called Mono.just() method with one String Value that is World.
- After that, it prints the result by using the .subscribe() method.
- Here, it first executed the Mono.just() method, after this we called then() on this publisher.
- After this, we called Mono.just() in the then() method again for handling another String value.
- Finally, it prints the result.
thenEmpty() method
This method similar to the then() method, but there is a little bit difference is there that is the thenEmpty() ignores the previous result of Mono.just publisher or Flux publisher. And this method can return empty value only.
Below we have provided the example and related output images with proper explanation for reference.
import reactor.core.publisher.Mono;
public class SpringReactiveMethods {
public static void main(String[] args) {
Mono.just("Hello")
.thenEmpty(Mono.empty())
.subscribe(System.out::println);
}
}
Output:
Note: This piece of Java code returns an empty value only.
Explanation of the above Program:
- In this example, in main method, we called the Mono.just method.
- In that method, we provide the String value.
- After that, we called .thenEmpty() method on Mono publisher. Again in .thenEmpty() method, we called the Mono.empty() again.
- First, we call the Mono.just() after this, we call the .thenEmpty() on this Mono publisher.
- Then, it prints the final result by using the .subscribe() method.
- Now, we call the .subscribe() method, in that method, we call the print statement result with help of the .subscribe() method.
thenMany() method
It is also similar to then() method. But it is used with a Flux publisher instead of Mono publisher. And it waits for both the source Flux and the other Publisher to complete and then emits elements from the Flux publisher.
Below, we have provided the example with related explanation and also, provided the related output image.
import reactor.core.publisher.Flux;
public class SpringReactiveMethods {
public static void main(String[] args) {
Flux.just(1, 2, 3)
.thenMany(Flux.just("A ", " B", " C"))
.subscribe(System.out::print);
}
}
Output:
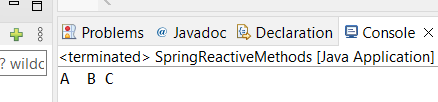
Explanation of the above Program:
- In this piece of code, in main method, we wrote the logic for thenMany() method.
- For this, first we call the Flux.just() method with some numerical elements.
- After this, on this Flux publisher, we call the .thenMany() method. In this method, again we call the Flux.just method with some String values.
- After this, we call the .subscribe() method. In this method, we have provided the print statement for print the final result.
- Basically, it first executes the Flux.just method with some elements, then it will execute the .thenMany method with some String elements.
- Then finally, it prints the result by using the .subscribe() method.
flatMapMany method
Basically, this method is used for transforming the elements emitted by the Flux publisher into other Flux or Mono publisher. Then flatten these into a single Flux. And it is particularly useful when dealing with asynchronous operations and it returns a Flux or Mono themselves. Below, we have provided the example on flatMapMany with related output.
import reactor.core.publisher.Flux;
public class SpringReactiveMethods {
public static void main(String[] args) {
Flux.just(1, 2, 3)
.flatMapMany(num -> Flux.range(num, 2))
.subscribe(System.out::print);
}
}
Output:
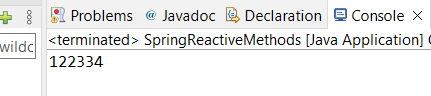
Explanation of the above Program:
- In this example, in main method, we called the Flux.just() method with some numerical elements.
- After this, on this publisher, we called the .flatMapMany() method. In this method, we have created one lambda expression with name num.
- This num lambda expression indicates Flux range from num to 2.
- On this publisher, we call the .subscribe method for printing the final result.
- We got the result as mentioned in the above image.