The Spring WebFlux is a part Spring Reactive framework. In Spring WebFlux, we can redirect requests by using the ServerResponse. This is one of the classes in Spring WebFlux which provides methods for creating responses. To direct a request in Spring WebFlux, we typically return the ServerResponse with appropriate HTTP status codes. These HTTP status codes can decide whether a request is successfully redirected or not. The HTTP status code and a location header indicating the URL to which the client request should be redirected.
In this article, we will learn about how to redirect a request in spring webflux. Below we have provided an example related to redirecting a request in Spring WebFlux.
Prerequisites:
To understand how to redirect a request in spring webflux, we need to have good knowledge of this list of topics.
- Spring WebFlux
- Spring Framework
- Spring WebFilter
- HTTP Codes
- Spring ServerWebExchange
- Error Handling in Spring WebFlux
Redirect a Request in Spring WebFlux
In the Spring Reactive project, we configured a REST controller to handle HTTP endpoints. Using @GetMapping we define a GET API endpoint, and internally, we create a method called redirectToAnotherUrl that returns void using the Mono publisher. This method takes a ServerWebExchange object as an argument.
We specified a target HTTP URL, for example, "https://www.geeksforgeeks.org/". We retrieve the response through the ServerWebExchange object and set the status code to HttpStatus.FOUND for a successful redirect. If the URL is not found, a status of "Not Found" is returned.
If the HTTP URL is available, we are able to redirect to the target HTTP URL using the setLocation() method of the exchange object. Finally, we return a response from the exchange object.
- WebFilter: The WebFilter is the interface that is used to define the filters that can be applied to the incoming HTTP requests. And it allows us to intercept and modify both incoming and outgoing responses. This filter method can be able to filter HTTP requests by using the ServerWebExchange object. In the Below code, you can see exchange object the getResponse method which can handle the HTTP requests.
- ServerWebExchange: The ServerWebExchange is also an interface which is used for represents the context of an HTTP requests and responses in the WebFlux. And It can provide the access to various requests and responses like header, body, URL and other attributes. In the java code I use getRequest() and getResponse(). The getRequest method is dealing incoming HTTP request and The getResponse method is dealing for outgoing HTTP response.
Program Implementation to Redirect a Request in Spring WebFlux
import java.net.URI;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.annotation.Bean;
import org.springframework.http.HttpStatus;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.server.ServerWebExchange;
import org.springframework.web.server.WebFilter;
import reactor.core.publisher.Mono;
@SpringBootApplication
public class RedirectRequest {
public static void main(String[] args) {
SpringApplication.run(RedirectRequestApplication.class, args);
}
@RestController
public static class MyController {
@GetMapping("/redirect")
public Mono<Void> redirectToAnotherUrl(ServerWebExchange exchange) {
// Redirect to another URL
String redirectUrl = "https://www.geeksforgeeks.org/";
// Set HTTP status code to 302 (Found) for redirection
exchange.getResponse().setStatusCode(HttpStatus.FOUND);
exchange.getResponse().getHeaders().setLocation(URI.create(redirectUrl));
// End response processing
return exchange.getResponse().setComplete();
}
}
@Bean
public WebFilter redirectFilter() {
return (exchange, chain) -> {
// Intercept requests and redirect if necessary
if (exchange.getRequest().getURI().getPath().equals("/old-url")) {
// Redirect to another URL
String redirectUrl = "https://example.com/new-url";
// Set HTTP status code to 302 (Found) for redirection
exchange.getResponse().setStatusCode(HttpStatus.FOUND);
exchange.getResponse().getHeaders().setLocation(URI.create(redirectUrl));
// End response processing
return exchange.getResponse().setComplete();
}
return chain.filter(exchange);
};
}
}
Explanation of the Code:
- First, we create one Rest Controller by using @RestController Spring annotation.
- After that, we have created one HTTP end point for redirect the request in Spring WebFlux.
- Then, we create a method with mono publisher which returns a response from HTTP status code.
- After this, we define the logic in that method by using ServerWebExchange interface.
- This interface is used for handling the incoming and outgoing requests and responses.
- And also, we define the required HTTP URL in that method.
- Then, we define that WebFilter by using @Bean Spring Annotation.
- This bean can handle the filtering process while HTTP URL redirecting.
- Finally, it returns the ServerWebExchange response through its object.
- Finally, it redirects the request in spring webflux with targeted HTTP URL.
Output:
Below we have provided the GIF as an output. After running the project as spring application, open browser and type below HTTP URL then we will see the output.
http://localhost:8080/redirect
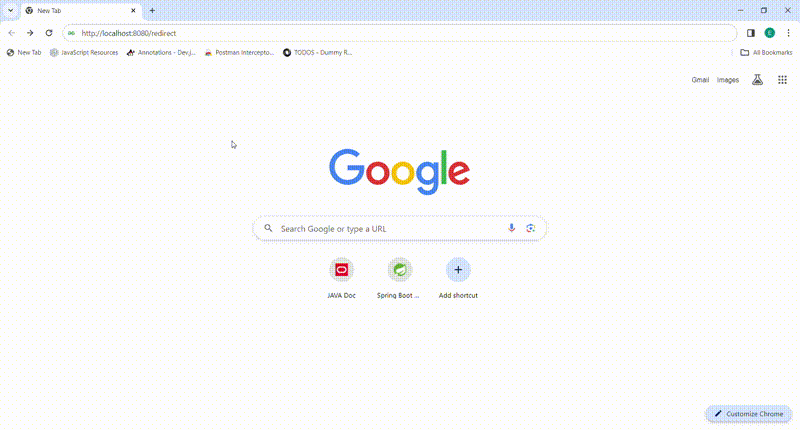