In web development, styling is an important aspect of building visually appealing user interfaces. styled-components, a popular library that allows you to write CSS directly in the JavaScript code. In this article, we'll explore how to use styled-components for global styling in React applications.
Prerequisites:
What are styled components?
styled-components is a library for React and React Native that helps to write CSS code within their JavaScript components. It uses tagged template literals to style components with the full power of JavaScript, including dynamic styles and props-based styling. styled-components promotes component-based styling, encapsulating styles within individual components for improved modularity and reusability.
Approach
- Global Styling: Use
GlobalStyle
from styled-components to set global styles for body margin, color, and font-family. - Custom Button Styling: Define
CustomButton
styled-component for button styles including padding, margin, color, border, and background-color. - Usage: Import and render
GlobalStyle
in the main component for global styles. UtilizeCustomButton
different props for styling variations.
Steps to implement Global styling
Step 1: Create a React Application using the following command
npx create-react-app globalstyles
Step 2: Once the project is created, move to the same directory i.e. globalStyles
cd globalStyles
Step 3: Run the below command in the React terminal to install styled-components to your project
npm install styled-components
Folder Structure
Folder Strucure
Dependencies
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"react": "^18.3.1",
"react-dom": "^18.3.1",
"react-scripts": "5.0.1",
"styled-components": "^6.1.9",
"web-vitals": "^2.1.4"
}
Example: Below example shows implementation of global styling using styled-components module
//App.js
import { CustomButton } from './styledComponents'
import { GlobalStyle } from './styledComponents'
const App = () => (
<>
<GlobalStyle />
<h1 style={{ color: "green" }}>Geeks For Geeks</h1>
<h2>React Global Styling using styled-components</h2>
<CustomButton type="button">Click Me</CustomButton>
<CustomButton type="button" outline>Click Me</CustomButton>
</>
);
export default App;
//styledComponents.js
import styled from "styled-components";
import { createGlobalStyle } from "styled-components";
export const CustomButton = styled.button`
padding:10px;
margin-right:20px;
font-size:15px;
color:${(props) => (props.outline ? "#0070c1" : "#ffffff")};
border-radius:4px;
border:2px solid #0070c1;
background-color: ${(props) => (props.outline ? "#ffffff" : "#0070c1")};
`;
export const GlobalStyle = createGlobalStyle`
body{
margin:0;
color:orange;
font-family: Arial sans-serif;
margin:20px;
}
/* Add more global styles here if needed */
`;
Start your application using the following command:
npm start
Output: Open your browser and go to https://localhost:3000/ you will see the following output
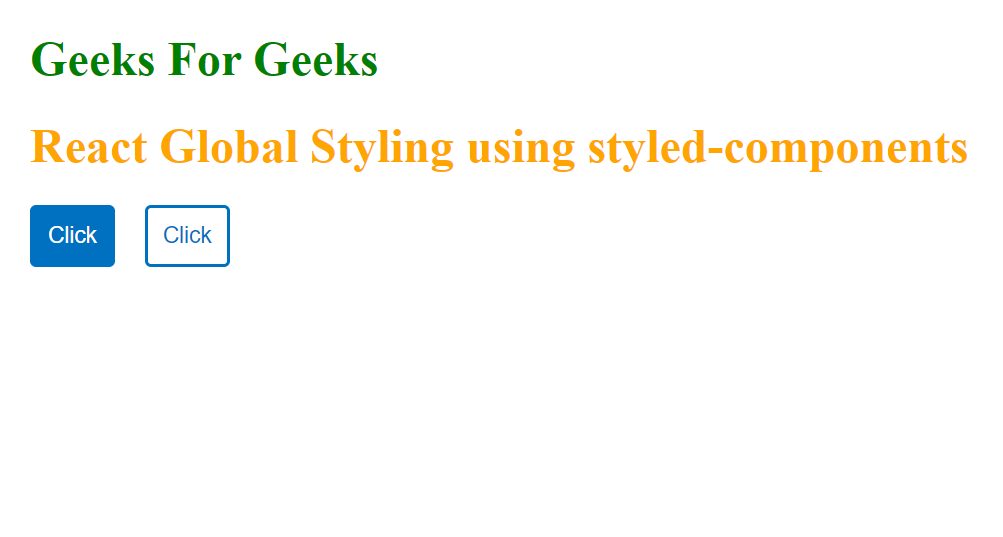
Global Styling with styled-components in React