Angular provides powerful tools for handling forms, with FormControl and FormGroup being fundamental building blocks. While both serve similar purposes, they have distinct roles and functionalities within Angular applications. In this article, we'll learn more about FormControl and FormGroup and will also see the differences between them.
Steps to Create Angular Application
Step 1: Create a basic angular application
ng new my-app
Step 2: Move to the project directory.
cd my-app
Step 3: Start the application using the following command.
ng serve --open
Folder Structure:
The updated Dependencies in package.json file.
"dependencies": {
"@angular/animations": "^17.2.0",
"@angular/common": "^17.2.0",
"@angular/compiler": "^17.2.0",
"@angular/core": "^17.2.0",
"@angular/forms": "^17.2.0",
"@angular/platform-browser": "^17.2.0",
"@angular/platform-browser-dynamic": "^17.2.0",
"@angular/platform-server": "^17.2.0",
"@angular/router": "^17.2.0",
"@angular/ssr": "^17.2.3",
"express": "^4.18.2",
"ngx-webstorage": "^13.0.1",
"rxjs": "~7.8.0",
"tslib": "^2.3.0",
"zone.js": "~0.14.3"
}
FormControl
A FormControl represents a single form control element, such as an input field, a dropdown, or a checkbox. It tracks the value and validation status of that input field.
Syntax:
import { FormControl } from '@angular/forms';
const myFormControl = new FormControl('Initial Value', [Validators]);
Features:
- Tracks the value changes of a single form control.
- Provides built-in and custom validation for individual form controls.
- Emits value changes and validation status changes as observables.
- Can be used with directives like ngModel or formControlName in template-driven or reactive forms.
- Supports synchronous and asynchronous validators for real-time validation.
Example:
//app.component.ts
import { Component } from '@angular/core';
import { FormControl } from '@angular/forms';
@Component({
selector: 'app-root',
template: `
<input type="text" [formControl]="myControl">
<p>Value: {{ myControl.value }}</p>
`
})
export class AppComponent {
myControl = new FormControl('Initial Value');
}
Output:
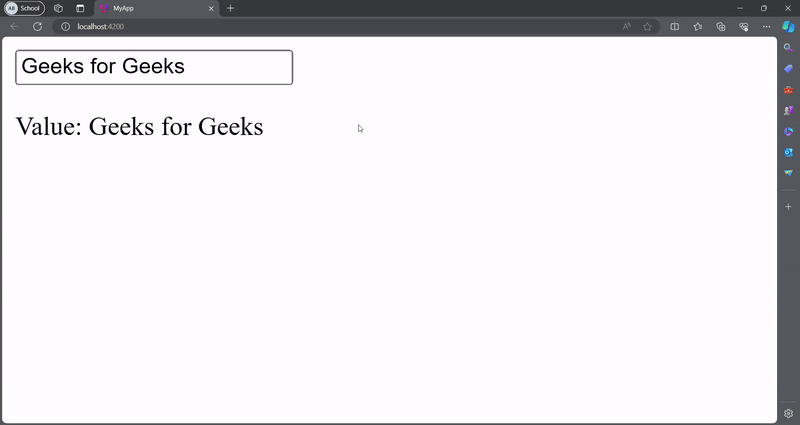
FormControl Output
FormGroup
A FormGroup represents a collection of form controls as a single unit, allowing you to manage and validate multiple form controls together. It's used to group related form controls together.
Syntax:
import { FormGroup, FormControl } from '@angular/forms';
const myFormGroup = new FormGroup({
control1: new FormControl('Value 1'),
control2: new FormControl('Value 2')
});
Features:
- Groups related form controls together for easier management.
- Tracks the value and validity of the entire group of form controls.
- Can be nested to create hierarchical forms.
- Provides methods to retrieve form control values and set their values programmatically.
- Supports validation at the group level, in addition to individual control validation.
Example:
//app.component.ts
import { Component } from '@angular/core';
import { FormGroup, FormControl } from '@angular/forms';
@Component({
selector: 'app-root',
template: `
<form [formGroup]="myForm">
<input type="text" formControlName="name">
<input type="text" formControlName="email">
<p>Form Value: {{ myForm.value | json }}</p>
</form>
`
})
export class AppComponent {
myForm = new FormGroup({
name: new FormControl('Batman'),
email: new FormControl('bruce.wayne@dc.inc')
});
}
Output:
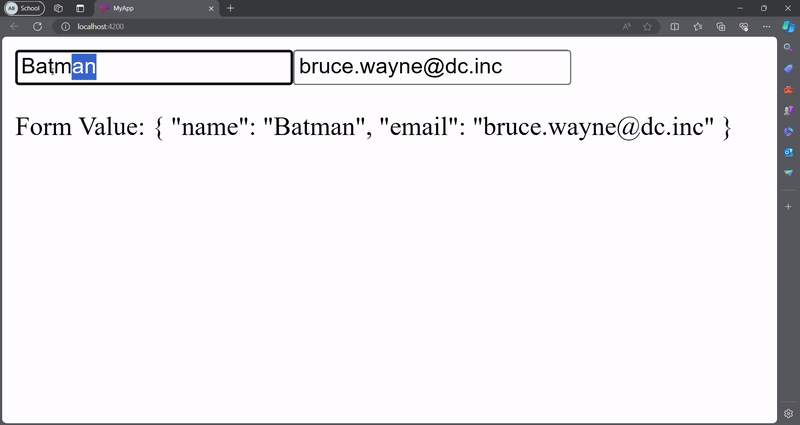
FormGroup Output
Differences Between FormControl and FormGroup
Feature | FormControl | FormGroup |
---|---|---|
Representation | Single form control element | Group of form controls |
Value Access | Provides access to the control's value | Provides access to values of all controls in the group |
Validation | Can have synchronous and asynchronous validators | Can have synchronous and asynchronous validators for the entire group |
Nested Structure | Cannot contain nested form controls | Can contain nested FormGroups and FormArrays |
Event Observables | Provides value changes and status changes for the control | Provides value changes and status changes for the entire group |
Conclusion
In Angular forms, FormControl and FormGroup play distinct roles in managing form controls and their validation. While FormControl is used for individual form controls, FormGroup is used to group related controls together. Understanding the differences between FormControl and FormGroup is important for effectively designing and implementing forms in Angular applications, ensuring better organization, validation, and user interaction.