This article is about building a Dashboard with Dynamic Data Updates using useEffect hook. In this project, I have used a weather API that fetches weather details of different cities. I took five cities fetched the weather data of each city in 6-second intervals and displayed the weather details on the dashboard.
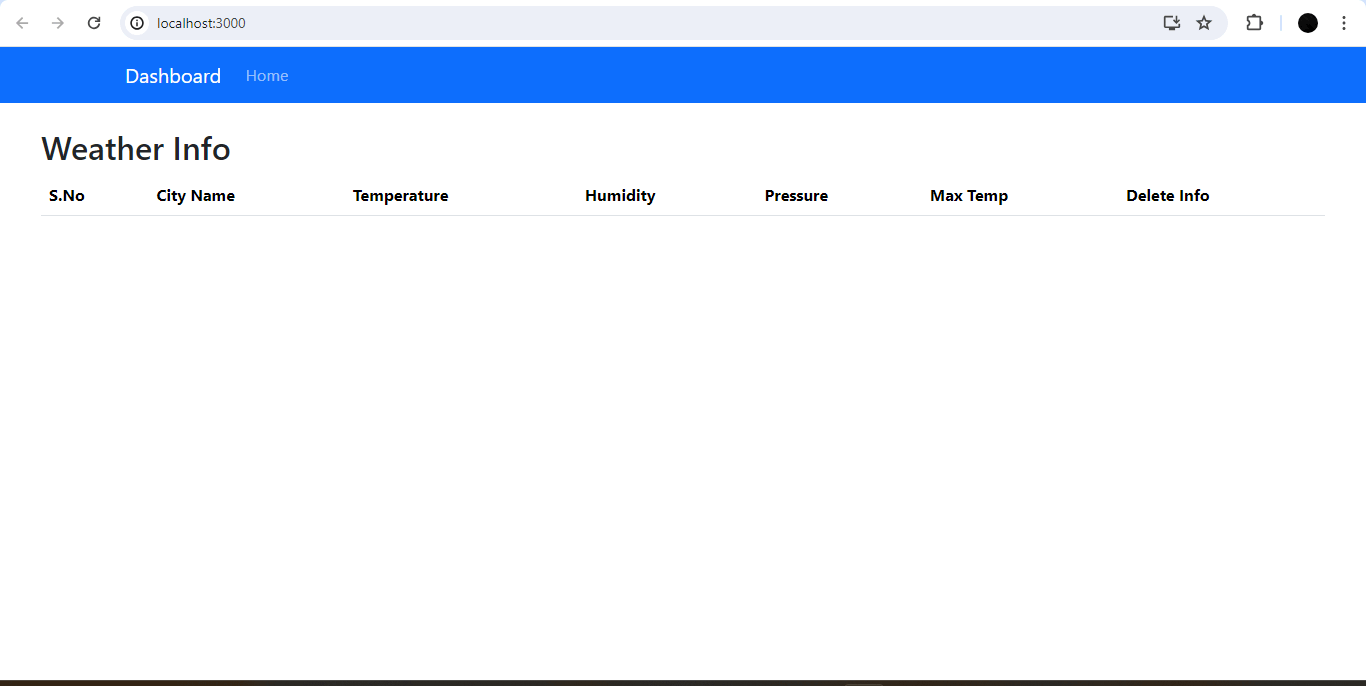
Final output
Prerequisites:
Approach:
- Install the required packages , we are using axios and react bootstrap. so install these packages.
- We are using two components a NavBar component and WeatherInfo component which has all the weather info of cities.
- The main code is in app.js file, in which we are fetching the weather data of each city within a interval and passing the weather data to weather info component as props.
Steps to create the project:
Step1: create the react app using the command
npx create-react-app weather-app
cd weather-app
Step2: Install the required dependencies
npm install react-bootstrap bootstrap
npm install axios
Project Structure:
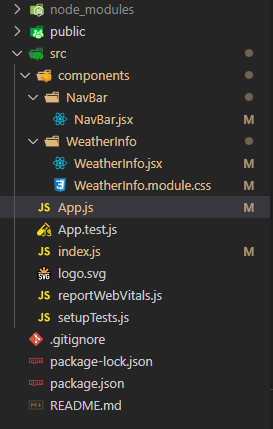
Project Structure
The updated dependencies in package.json file looks like this:
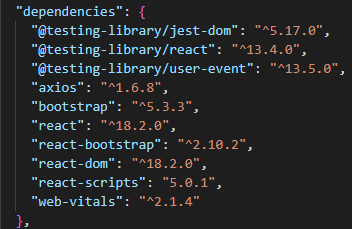
project-dependencies
Example: To demonsrtate building a dashboard with dynamic data updates using useEffects.
/* src/components/WeatherInfo/WeatherInfo.module.css */
.main{
margin-top: 4vh;
margin-left: 3vw;
margin-right: 3vw;
}
//index.js
import React from "react";
import ReactDOM from "react-dom/client";
import App from "./App";
import reportWebVitals from "./reportWebVitals";
import "bootstrap/dist/css/bootstrap.min.css";
const root = ReactDOM.createRoot(document.getElementById("root"));
root.render(
<React.StrictMode>
<App />
</React.StrictMode>
);
// If you want to start measuring performance in your app, pass a function
// to log results (for example: reportWebVitals(console.log))
// or send to an analytics endpoint. Learn more: https://bit.ly/CRA-vitals
reportWebVitals();
// app.js
import NavBar from "./components/NavBar/NavBar";
import WeatherInfo from "./components/WeatherInfo/WeatherInfo";
import { useEffect, useState } from "react";
import axios from "axios";
function App() {
const [cityNames, setCityNames] = useState([
"delhi",
"hyderabad",
"kerala",
"mumbai",
"chennai",
]);
const [citiesWeatherData, setCitiesWeatherData] = useState([]);
useEffect(() => {
const interval = setInterval(() => {
let i = 0;
const randomNumber = Math.floor(Math.random() * cityNames.length);
const city = cityNames[randomNumber];
getweatherData(city);
}, 6000);
return () => clearInterval(interval);
}, []);
async function getweatherData(cityName) {
axios
.get(
`https://api.openweathermap.org/data/2.5/weather?q=${cityName}&appid=b1541e5619f8ac25fa684285493a4352`
)
.then((res) => {
const city = res?.data;
const cityWeatherData = {};
cityWeatherData.humidity = city?.main?.humidity;
cityWeatherData.temperature = city?.main?.temp;
cityWeatherData.pressure = city?.main?.pressure;
cityWeatherData.min_temp = city?.main?.temp_min;
cityWeatherData.max_temp = city?.main?.temp_max;
cityWeatherData.cityName = cityName;
setCitiesWeatherData((prevCitiesWeatherData) => [
...prevCitiesWeatherData,
cityWeatherData,
]);
})
.catch((err) => {
console.log("errorr ", err);
});
}
return (
<div className="App">
<NavBar />
<WeatherInfo
citiesWeatherData={citiesWeatherData}
setCitiesWeatherData={setCitiesWeatherData}
/>
</div>
);
}
export default App;
//src/components/NavBar/NavBar.jsx
import Container from 'react-bootstrap/Container';
import Nav from 'react-bootstrap/Nav';
import Navbar from 'react-bootstrap/Navbar';
function NavBar() {
return (
<>
<Navbar bg="primary" data-bs-theme="dark">
<Container>
<Navbar.Brand href="#home">Weather App</Navbar.Brand>
<Nav className="me-auto">
<Nav.Link href="#home">Home</Nav.Link>
</Nav>
</Container>
</Navbar>
</>
);
}
export default NavBar;
// src/components/WeatherInfo/WeatherInfo.jsx
import React from 'react'
import axios from 'axios'
import Table from 'react-bootstrap/Table';
import Button from 'react-bootstrap/Button';
import Style from './WeatherInfo.module.css'
export default function WeatherInfo({ citiesWeatherData, setCitiesWeatherData }) {
return (
<div className={Style.main}>
<h2>Weather Info</h2>
<Table responsive="sm">
<thead>
<tr>
<th>S.No</th>
<th>City Name</th>
<th>Temperature</th>
<th>Humidity</th>
<th>Pressure</th>
<th>Max Temp</th>
<th>Delete Info</th>
</tr>
</thead>
<tbody>
{citiesWeatherData.map((city, index) => (
<tr key={index}>
<td>{index + 1}</td>
<td>{city.cityName}</td>
<td>{city.temperature}</td>
<td>{city.humidity}</td>
<td>{city.pressure}</td>
<td>{city.max_temp}</td>
<td> <Button variant="danger" onClick={(index) => setCitiesWeatherData(prevCitiesWeatherData => {
const updatedCitiesWeatherData = [...prevCitiesWeatherData];
updatedCitiesWeatherData.splice(index, 1);
return updatedCitiesWeatherData;
})}>Delete</Button></td>
</tr>
))}
</tbody>
</Table>
</div >
)
}
To Start Project:
npm start
Output:
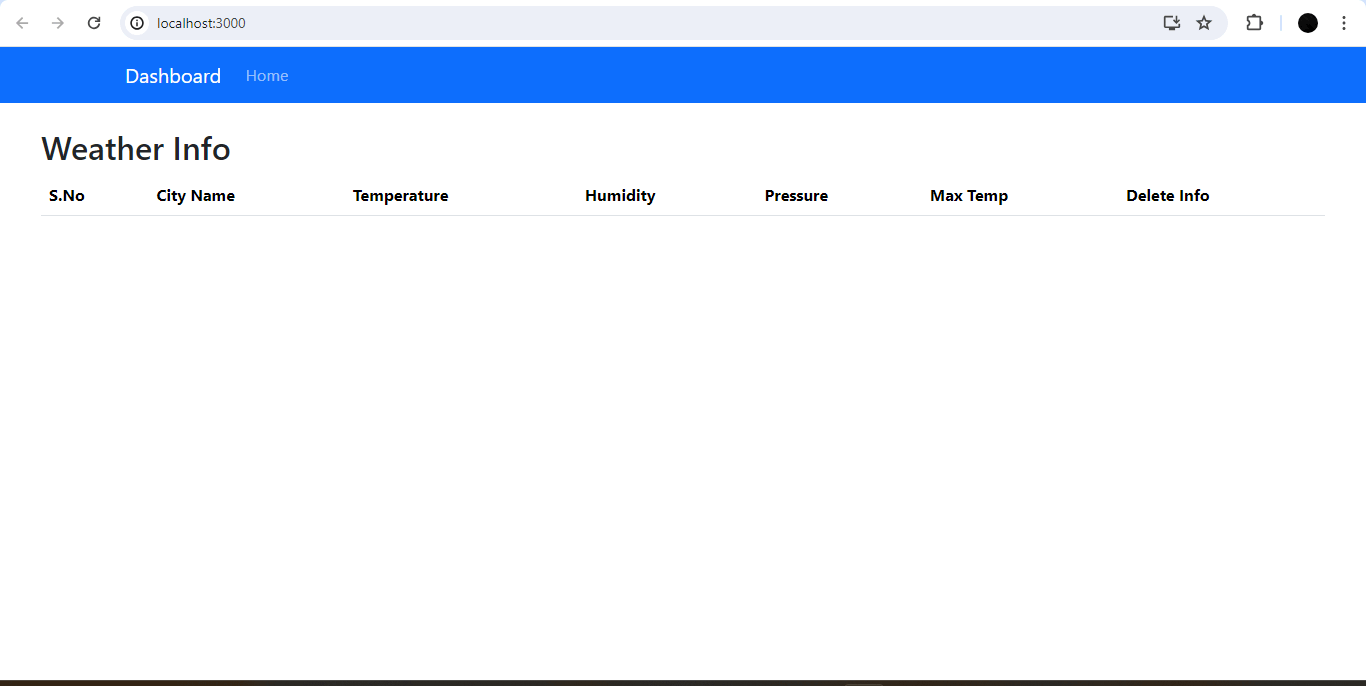
Final Output