In this article, we will delve into the Spell Corrector GUI method using Streamlit. As we are well aware, human language is a complex and ever-evolving system, resulting in frequent misspellings and grammatical errors. These errors can manifest as spelling, punctuation, grammatical, or word choice mistakes. Here, we will introduce a Grammar Corrector Application built with Python and Machine Learning to address this issue.
Python Spell Corrector GUI Using Streamlit
Below is the step-by-step implementation of Python Spell Corrector GUI using Streamlit in Python:
Step 1: Create a Virtual Environment
First, create the virtual environment using the below commands
python -m venv env
.\env\Scripts\activate.ps1
Step 2: Install Necessary Libraries
Install 'transformers' and 'torch' for NLP/ML, 'autocorrect' for spell-checking, 'spacy' for the 'en' language model, and 'streamlit' for web app development. Incorporate 'Gramformer' from GitHub for advanced grammar correction, creating a user-friendly interface for diverse NLP tasks.
pip install transformers
pip install torch
pip install autocorrect
pip install streamlit
pip install spacy
pip install git+https://github.com/PrithivirajDamodaran/Gramformer.git
After installing the aforementioned packages, execute the following code in your terminal from the project directory. This code installs the 'en' language model using spaCy:
python -m spacy download en
Step 3: Writing Python Code
app.py
Below, Python code employs the Streamlit framework to create a web app titled "Grammar Corrector." Users can input text into a designated area. The `process` function is defined to handle text processing, downloading the 'en' language model for spaCy, initializing Gramformer and Autocorrect models, correcting spelling, and applying grammar correction. When the "Process" button is clicked, the user input undergoes text processing, and the corrected text is displayed under the subheader "Corrected Text" in the Streamlit app.
import streamlit as st
from gramformer import Gramformer
from autocorrect import Speller
import subprocess
st.title("Grammar Corrector")
user_input = st.text_area("Enter Text Here")
def process(text):
command = "python -m spacy download en"
subprocess.run(command, shell=True)
gf = Gramformer(models=1, use_gpu=False)
spell = Speller()
corrected = spell(text)
ans = ""
res = gf.correct(corrected)
for ele in res:
ans += ele
return ans
if st.button("Process"):
processed_text = process(user_input)
st.subheader("Corrected Text:")
st.write(processed_text)
Step 4: Run the Server
For run the server use the below command
streamlit run script_name.py
Output
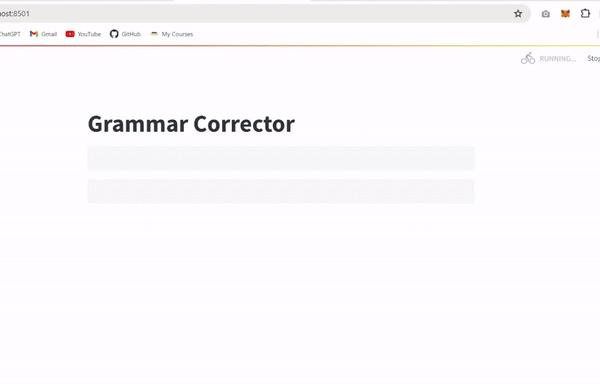
Python – Spell Corrector GUI using Streamlit