The Validators class in Angular provides a set of built-in validation functions that can be used to validate form controls and user input. It is part of the @angular/forms module and is commonly used in conjunction with Angular's Reactive Forms or Template-driven Forms.
Prerequisites
Key Functions of Validators Class
The Validators class provides several static methods that return validation functions. These validation functions can be used to validate form controls based on different criteria, such as required fields, minimum and maximum values, pattern matching, and more.
Some of the commonly used validation functions provided by the Validators class include:
- required: Validates that the control value is not null or an empty string.
- minLength: Validates that the control value has a minimum length.
- maxLength: Validates that the control value does not exceed a maximum length.
- pattern: Validates that the control value matches a regular expression pattern.
- email: Validates that the control value is a valid email address.
- min: Validates that the control value is greater than or equal to a specified minimum value.
- max: Validates that the control value is less than or equal to a specified maximum value.
Features of Validator Class
The Validators class provides several benefits and features:
- Built-in Validation Functions: Angular comes with a set of built-in validation functions that cover many common validation scenarios, reducing the need to write custom validation logic.
- Reusable Validation Functions: The validation functions provided by the Validators class are reusable across different form controls and components.
- Composable Validation Functions: Multiple validation functions can be combined to create more complex validation rules using the Validators.compose function.
- Cross-Field Validation: Angular also supports cross-field validation, which allows you to validate multiple form controls based on their combined values.
Steps to implement Validators
Step 1: Set up a new Angular application
ng new my-app
cd validators-example
Folder Structure
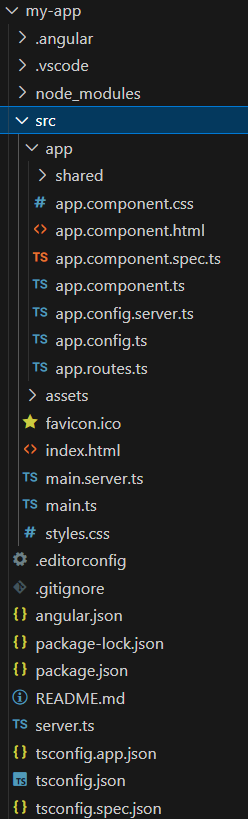
folder structure
Dependencies
"dependencies": {
"@angular/animations": "^17.3.0",
"@angular/common": "^17.3.0",
"@angular/compiler": "^17.3.0",
"@angular/core": "^17.3.0",
"@angular/forms": "^17.3.0",
"@angular/platform-browser": "^17.3.0",
"@angular/platform-browser-dynamic": "^17.3.0",
"@angular/platform-server": "^17.3.0",
"@angular/router": "^17.3.0",
"@angular/ssr": "^17.3.3",
"express": "^4.18.2",
"rxjs": "~7.8.0",
"tslib": "^2.3.0",
"zone.js": "~0.14.3"
}
Example
<!-- app.component.html -->
<form [formGroup]="userForm" (ngSubmit)="onSubmit()">
<div>
<label for="name">Name</label>
<input id="name" type="text" formControlName="name" />
<div *ngIf="name?.invalid && (name?.dirty || name?.touched)">
<div *ngIf="name?.errors?.['required']">Name is required.</div>
<div *ngIf="name?.errors?.['minlength']">
Name must be at least 3 characters long.
</div>
<div *ngIf="name?.errors?.['maxlength']">
Name cannot exceed 20 characters.
</div>
</div>
</div>
<div>
<label for="email">Email</label>
<input id="email" type="email" formControlName="email" />
<div *ngIf="email?.invalid && (email?.dirty || email?.touched)">
<div *ngIf="email?.errors?.['required']">Email is required.</div>
<div *ngIf="email?.errors?.['email']">Invalid email format.</div>
</div>
</div>
<div>
<label for="password">Password</label>
<input id="password" type="password" formControlName="password" />
<div *ngIf="password?.invalid && (password?.dirty || password?.touched)">
<div *ngIf="password?.errors?.['required']">Password is required.</div>
<div *ngIf="password?.errors?.['pattern']">
Password must contain at least one uppercase letter, one lowercase
letter, one digit, and one special character.
</div>
</div>
</div>
<div>
<label for="age">Age</label>
<input id="age" type="number" formControlName="age" />
<div *ngIf="age?.invalid && (age?.dirty || age?.touched)">
<div *ngIf="age?.errors?.['required']">Age is required.</div>
<div *ngIf="age?.errors?.['min']">Age must be at least 18.</div>
<div *ngIf="age?.errors?.['max']">Age cannot exceed 65.</div>
</div>
</div>
<button type="submit" [disabled]="userForm.invalid">Submit</button>
</form>
//app.component.ts
import { NgIf } from '@angular/common';
import { Component, inject } from '@angular/core';
import {
AbstractControl,
FormBuilder,
FormControl,
FormGroup,
FormsModule,
ReactiveFormsModule,
ValidationErrors,
ValidatorFn,
Validators,
} from '@angular/forms';
@Component({
selector: 'app-root',
standalone: true,
imports: [NgIf, ReactiveFormsModule, FormsModule],
templateUrl: './app.component.html',
styleUrl: './app.component.css',
})
export class AppComponent {
userForm: FormGroup;
constructor(private fb: FormBuilder) {
this.userForm = this.fb.group({
name: [
'',
[
Validators.required,
Validators.minLength(3),
Validators.maxLength(20),
],
],
email: ['', [Validators.required, Validators.email]],
password: [
'',
[
Validators.required,
Validators.pattern(
'^(?=.*[a-z])(?=.*[A-Z])(?=.*\\d)
(?=.*[$@$!%*?&])[A-Za-z\\d$@$!%*?&]{8,}'
),
],
],
age: ['', [Validators.required, Validators.min(18), Validators.max(65)]],
});
}
get name() {
return this.userForm.get('name');
}
get email() {
return this.userForm.get('email');
}
get password() {
return this.userForm.get('password');
}
get age() {
return this.userForm.get('age');
}
onSubmit() {
if (this.userForm.valid) {
console.log('Form submitted:', this.userForm.value);
}
}
}
Explanation
In this example, we have created a user form with four form controls: name, email, password, and age. Each form control is validated using various validation functions from the Validators class, such as required, minLength, maxLength, email, pattern, min, and max.
The validation errors are displayed in the template using Angular's built-in *ngIf directive, which shows or hides error messages based on the validity of the form controls.
When the user submits the form, the onSubmit method is called, which checks if the form is valid. If the form is valid, the form data can be processed further, such as sending it to a server.