Node.js is an open-source and cross-platform JavaScript runtime environment. It’s a powerful tool suitable for a wide range of projects. Node.js stands out as a game-changer. Imagine using the power of JavaScript not only in your browser but also on the server side.
Table of Content
What is MERN stack?
The MERN Stack is a JavaScript Stack that makes the deployment of full-stack web applications easier and faster. It includes four technologies: MongoDB, Express, React, and Node.js. MERN Stack is designed to simplify the development process and make it smoother.
- MongoDB: Non-Relational Database
- Express: Node.js web server
- React: JavaScript Frontend Framework
- Node: JavaScript Web Server
What is Node?
Node.js is an open-source, cross-platform JavaScript runtime built on Chrome’s V8 JavaScript engine. It allows the creation of scalable Web servers without threading and networking tools using JavaScript and a collection of “modules” that handle various core functionalities. It can make console-based and web-based node.js applications.
Why Node?
Node.js is used to build back-end services like APIs like Web App, Mobile App or Web Server. A Web Server will open a file on the server and return the content to the client. It’s used in production by large companies such as Paypal, Uber, Netflix, Walmart, and so on.Here are some reasons to choose Node.js :-
- Easy to Get Started: Node.js is beginner-friendly and ideal for prototyping and agile development.
- Scalability: It scales both horizontally and vertically.
- Real-Time Web Apps: Node.js excels in real-time synchronization.
- Fast Suite: It handles operations quickly (e.g., database access, network connections).
- Unified Language: JavaScript everywhere—frontend and backend.
- Rich Ecosystem: Node.js boasts a large open-source library and supports asynchronous, non-blocking programming.
Key features of Node
- Non-blocking I/O: Node.js is asynchronous, enabling efficient handling of concurrent requests.
- Event-driven architecture: Developers can create event-driven applications using callbacks and event emitters.
- Extensive module ecosystem: npm (Node Package Manager) provides access to thousands of reusable packages.
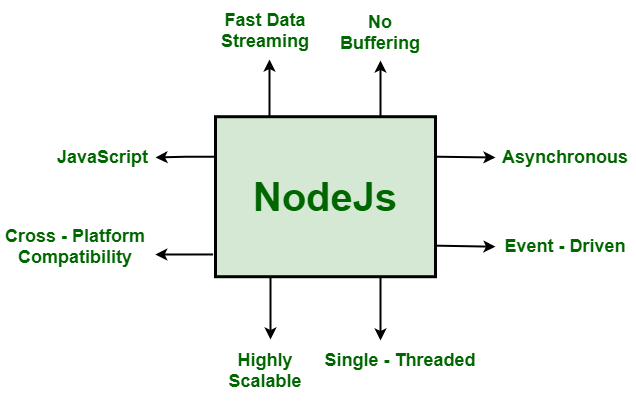
Node Advantages
- Easy Scalability: Node JS is built upon Chrome V8’s engine powered by Google. It allows Node to provide a server-side runtime environment that compiles and executes JavaScript at lightning speeds.
- Real-time web apps: Today the web has become much more about interaction. Users want to interact with each other in real-time. Chat, gaming, constant social media updates, collaboration tools, eCommerce websites, real-time tracking apps, marketplace, each of these features requires real-time communication between users, clients, and servers across the web.
- Fast Suite: As we have discussed that Node is highly scalable and lightweight that’s why it’s a heavy favorite for microservice architectures. In a nutshell, microservice architectures mean breaking down the application into isolated and independent services.
- Easy to learn and code: No matter what language you are using for the backend application you’re gonna need JavaScript for front-end anyway so instead of spending your time learning a server-side language such as Php, Java or Ruby on Rails, you can spend all your efforts in learning JS and mastering in it.
- Data Streaming: Node comes to the rescue since it’s good at handling such an I/O process which allows users to transcode media files simultaneously while they are being uploaded. It takes less time compared to other data processing methods for processing data.
- Non Blocking Event-Drive Archtecture: Unlikly other traditional web servers that wait for one request to finish before handling another, Node.js uses an event-driven architecture and this things makes effective for handling many concurrent requests.
- Corporate Support: It’s an independent community aimed at facilitating the development of Node core tools. The foundation of Node was formed to speed up the development of Node, and it was intended to allow broad adoption of it.
How NodeJS works?
Node accepts the request from the clients and sends the response, while working with the request node.js handles them with a single thread. To operate I/O operations or requests node.js use the concept of threads. Thread is a sequence of instructions that the server needs to perform. It runs parallel on the server to provide the information to multiple clients. Node.js is an event loop single-threaded language. It can handle concurrent requests with a single thread without blocking it for one request.
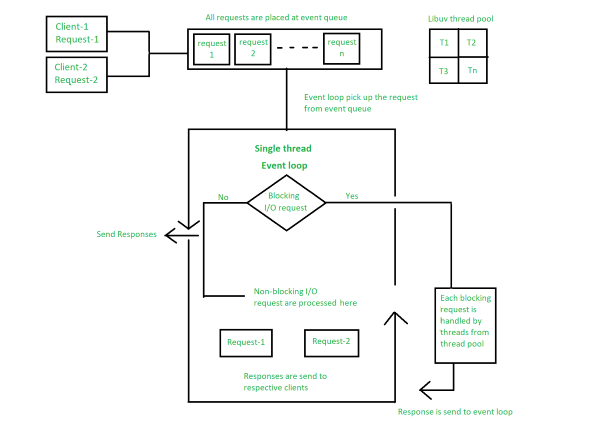
Application of NodeJS
- Web Development: Node.js powers server-side logic for web applications, handling HTTP requests and data processing efficiently.
- Real-time Applications: It's ideal for building chat apps, online gaming platforms, and live streaming services due to its event-driven architecture and WebSocket support.
- API Development: Node.js simplifies creating RESTful APIs and microservices, making it easy to handle requests, data validation, and response generation.
- Single-page Applications: Used as the back-end for SPAs built with front-end frameworks like React and Angular, managing server-side logic and API integrations.
- IoT (Internet of Things): Node.js is lightweight and suitable for managing IoT devices, handling sensor data, and facilitating communication between devices and cloud services.
- Streaming Services: It's efficient for building video/audio streaming platforms, real-time analytics dashboards, and live broadcasting services due to its non-blocking I/O capabilities.
NodeJS Ecosystem
Node.js has a vibrant ecosystem with a plethora of libraries, frameworks, and tools. Here are some key components:
- npm (Node Package Manager): npm is the default package manager for Node.js. It allows developers to install, manage, and share reusable code packages (called modules). You can find thousands of open-source packages on the npm registry.
- Express.js: Express is a popular web application framework for Node.js. It simplifies routing, middleware handling, and request/response management. Many developers choose Express for building APIs, web servers, and single-page applications.
- Socket.io: For real-time communication, Socket.io is a go-to library. It enables bidirectional communication between the server and clients using WebSockets or fallback mechanisms.
- Mongoose: If you’re working with MongoDB (a NoSQL database), Mongoose provides an elegant way to model your data and interact with the database. It offers schema validation, middleware, and query building.
Creating a Simple Node Application
Step 1: Create a folder for the project using the following command.
mkdir node-basics
cd node-basics
Step 2: Initialize the Node application using the following command.
npm init -y
Step 3: Create a file server.js to create a simple hello program.
touch server.js
Project Structure:
Folder Structure
Example: Illustration to showcase the basic structure for Node.js
//server.js
const http = require('http');
const server = http.createServer((req, res) => {
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.write('Hello, World!');
res.end();
});
server.listen(3000, () => {
console.log('Server is running on port 3000');
});
Step 4: To start the server run the following command.
node server.js
Output:
Creating a Simple API
Use Express.js to create API endpoints that handle HTTP requests and responses.
//index.js
const express = require('express');
const app = express();
app.get('/api/hello', (req, res) => {
res.send('Hello from Node.js!');
});
const PORT = process.env.PORT || 5000;
app.listen(PORT, () => {
console.log(`Server running on port ${PORT}`);
});
Output:
Connecting to MongoDB
Use Mongoose to connect your Node.js application to MongoDB and define data models.
const mongoose = require('mongoose');
async function connectToDatabase() {
try {
// Connect to MongoDB using the provided connection string
await mongoose.connect('mongodb://127.0.0.1:27017/myapp', {
useNewUrlParser: true,
useUnifiedTopology: true
});
console.log("Database Connected");
} catch (error) {
console.error("Error connecting to database:", error);
}
}
connectToDatabase();
// Define the user schema and model
// after establishing the connection
const Schema = mongoose.Schema;
const userSchema = new Schema({
name: String,
email: String,
age: Number
});
// Create a User model based on the userSchema
const User = mongoose.model('User', userSchema);
// Export the User model to use it elsewhere if needed
module.exports = User;
Output:
Conclusion
Node.js is a powerful platform for building scalable and real-time applications, especially in the context of the MERN stack. By understanding Node.js basics, its event-driven architecture, and integration with other technologies like Express.js and MongoDB, developers can leverage its capabilities to create robust back-end systems for modern web applications. Start exploring Node.js today and unlock the full potential of the MERN stack for your projects.