'Partition' in Scala is a method used to split a collection a List or a tuple into separate collections, lists, or tuples based on a predicate function/method.
- In the Output, we get two collections, lists, or tuples. The first one contains the elements that satisfy or align with the predicate method and the other list or collection contains those elements which do not satisfy or align with the method.
- Here, the role of the predicate method is to provide the functionality of conditions or criteria to split the elements.
Usage:
- It is mainly used to split a collection into two groups based on a condition or criteria.
- It eliminates the need for iteration over the collection for splitting or filtering elements based on a given criteria.
- It also reduces boilerplate code to an extent.
- It required a predicate function that provides the condition for the splitting process.
Let's consider a simple example of the same.
Example 1:
Scala
object PartitionMethodExample1{
def main(args: Array[String]): Unit = {
val numberslist = List(1,2,3,4,5,6,7,8)
//define a predicate function
def isEvenNumber(n: Int): Boolean = n % 2 == 0
//Use partition to split the list into separate collections
val(evenNumbers, oddNumbers) = numberslist.partition(isEvenNumber)
//Print the output
println("Even Numbers: "+ evenNumbers)
println("Odd Numbers: "+ oddNumbers)
}
}
Explanation:
- The above block of code, splits a list of number into an even number and odd number list.
- There is a value named 'numberslist' which has a list with integers from 1-8 within it.
- Then we define a predicate function which provides the condition for splitting. The function takes an integer 'n' and returns true if it is an even number. The number is even or not is checked through the condition provided within it i.e. 'n % 2 == 0'.
- Then next line of code after the predicate function uses 'partition' on the list - 'numberslist'. The method splits the list into two separate list based on the result of applying the predicate method. The resulting 'evenNumbers' contains those values which were true means the even numbers and odd number contains the odd numbers.
Output:
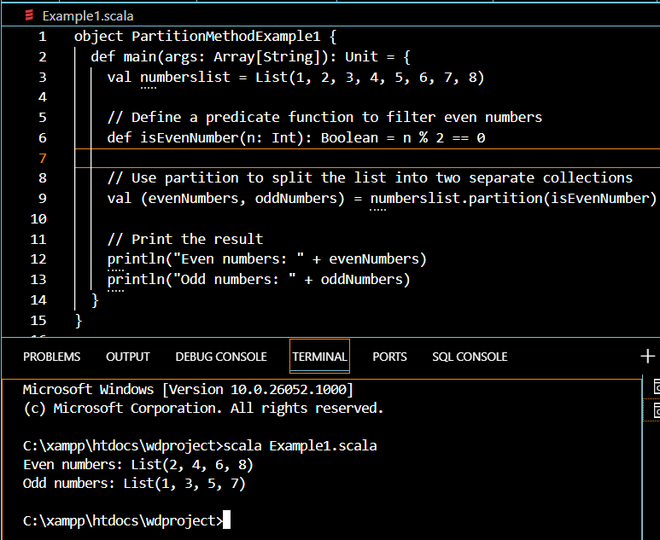
Example-1
Example 2:
Scala
object PartitionMethodExample2{
def main(args: Array[String]){
val words = Set("Channel", "Gucci", "Louis Vuitton", "Versace", "Adidas", "Allen Solly", "H&M", "Belanciaga", "Zara")
//Define a predicate function to filter short words
def isShortWord(word: String): Boolean = word.length <= 7
//Use Partition to split the set into two collections.
val(shortWords, longWords) = words.partition(isShortWord)
//print the output of partition method
println("Short Words: " + shortWords)
println("Long Words: " + longWords)
}
}
Explanation:
- In above Example 2, we used a set which contains brand names.
- Now we wanted to split the Set on the bases of the length of the brand name i.e. whichever brand name has a length less than or equal to 7, those will go in short words and others in log words.
- The 'isShortWord' method is a predicate method which contributed with the condition.
- Next, the partition method is applied with the Set of words by passing the predicate function in the partition method.
- If the condition is true i.e. the words gets stored in first Set i.e. 'shortWords' and the words which do not align with the condition gets stored in Set 'longWords'.
Output:
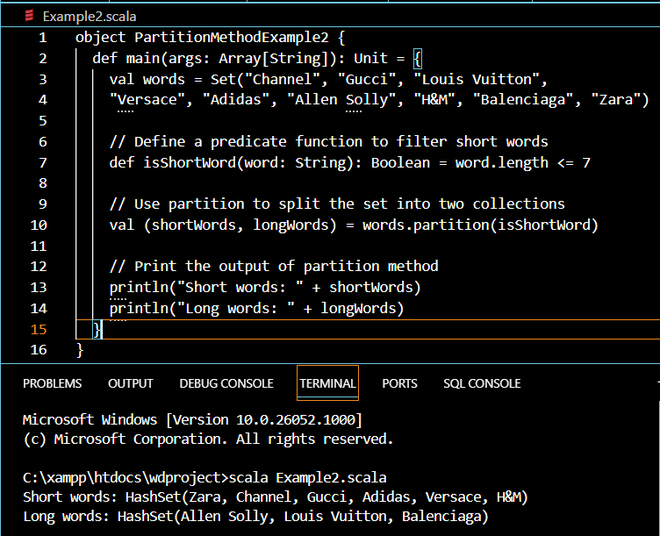
Example-2
Article Tags :
Recommended Articles
10. Scala Keywords