What is Ctrl + V ?
The ctrl+V is a keyboard shortcut used to paste anything from anywhere. It can be disabled for a particular task or page. Let's see how to disable cut, copy, paste, and right-click.
To disable the ctrl+V (paste) keyboard shortcut in JavaScript, you would typically capture the keydown event and prevent the default behavior if the ctrl key and V key are pressed simultaneously.
Below are the approaches to disable Ctrl+V in JavaScript:
Table of Content
Using JQuery
JQuery is a fast, small, and feature-rich JavaScript library. It simplifies tasks like HTML document traversal and manipulation, event handling, animation, and AJAX.
Syntax :
$(document).ready(function(){
$('#txtInput').on("paste",function(e) {
e.preventDefault();
});
});
Explanation:
- $(document).ready(function(){...}): This is a jQuery shorthand for the document ready event handler. It ensures that the code inside the function is executed when the DOM is fully loaded and ready to be manipulated.
- $('#txtInput'): This selects an element with the ID txtInput.
- .on("cut copy paste", function(e) {...}): This attaches event handlers to the specified events - paste.
- function(e) {...}: This is the callback function that gets executed when the paste event occured.
- e.preventDefault();: This line prevents the default behavior of the event. In this case, it prevents the default behavior of the paste.
Example: Below is an example of disabling ctrl+v in javascript using JQuery.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>Disable Paste</title>
<script src=
"https://code.jquery.com/jquery-3.6.0.min.js">
</script>
<script>
$(document).ready(function () {
$('#txtInput2').on("paste", function (e) {
e.preventDefault();
alert("Paste functionality disabled.");
});
});
</script>
</head>
<body>
<h1>Try to Paste This</h1>
<input type="text" id="txtInput1"
placeholder="Paste Is Enabled">
<br>
<br>
<input type="text" id="txtInput2"
placeholder="Paste Is Disabled">
</body>
</html>
Output: In this Example it will show a alert - Paste functionality disabled
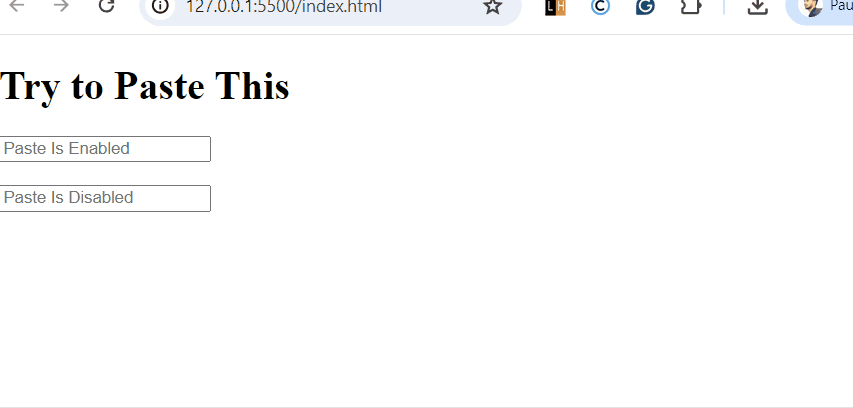
Disable ctrl+v in javascript using JQuery
Using EventListner
An EventListener in JavaScript waits for a specified event, like a click or key press, on a DOM element and triggers a function to execute in response to that event.
Syntax:
const inputField = document.getElementById('email'); // fetch Id of Inputfield
inputField.addEventListener('paste', e => e.preventDefault());
Example: Below is an example of disabling ctrl+v in javascript using EventListner.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<h2>Text To Copy</h2>
<label>CRTL V Disabled </label><br>
<input type="text" id="email" required> <br><br>
<label>CRTL V Enabled </label><br>
<input type="text" id="pass" required>
<script>
const inputField =
document.getElementById('email');
inputField.addEventListener('paste', e => e.preventDefault());
</script>
</body>
</html>
Output:
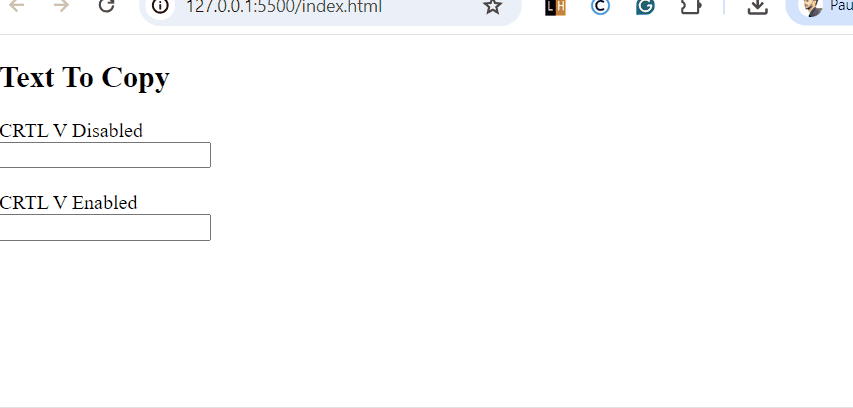
Disable ctrl+v in javascript using EventListner