MongoDB is a popular, open-source, NoSQL (non-relational) database that provides high performance, high availability, and easy scalability. Unlike traditional relational databases, MongoDB stores a JSON-like format called BSON (Binary JSON).
In this article, we connect the MongoDB database to your backend application built by Node.js.
Setup for Database Connection
- Installation : For connecting MongoDB to the backend, you have to install MongoDB on your local system or you can also create an account on MongoDB atlas and get your MongoDB credentials like URL.
- Node.js Driver: The next step is to install the Node.js to your local system ( if it is not available)
- Module: Installing the module ( MongoDB, Mongoose ) to connect the database.
- Connect to MongoDB: This step establishes a connection to the MongoDB server.
Below mentioned are 2 approaches to connect database in the application.
Table of Content
Using MongoDB
Install MongoDB module. Establish a connection to the MongoDB database by creating an instance of the MongoClient class and providing the MongoDB connection URI.
Steps to Create Application
Step 1. Project Structure : Make a folder named 'mongodb-connection' and navigate to it using this command.
mkdir mongodb-connection
cd mongodb-connection
Step 2. Install Module : Install required module like mongodb , express.
npm i mongodb express dotenv
Updated dependencies in package.json file
"dependencies": {
"dotenv": "^16.4.5",
"express": "^4.19.2",
"mongodb": "^6.6.0"
}
Project Structure:
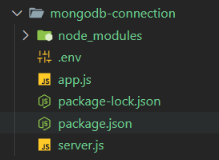
Project Structure
Example: This example demonstrates connection to database using the MongoDB.
// server.js
const { MongoClient } = require('mongodb');
// Connect to the MongoDB server
async function connectToMongoDB() {
// Create a new MongoClient
const client = new MongoClient(process.env.DB_URI);
try {
await client.connect();
console.log('Connected to MongoDB');
return client.db();
} catch (error) {
console.error('Error connecting to MongoDB:', error);
throw error;
}
}
module.exports = connectToMongoDB;
// app.js
const express = require('express')
const app = express();
const dotenv = require('dotenv')
const connectToMongoDB = require('./server')
dotenv.config()
const PORT = process.env.PORT || 5000
// Function to insert an item
async function insertItem(itemData) {
try {
const db = await connectToMongoDB();
const collection = db.collection('items');
const result = await collection.insertOne(itemData);
console.log('Item inserted : ', result);
return result;
} catch (error) {
console.error('Error inserting item:', error);
throw error;
}
}
// Example usage
const newItemData = { name: 'Example Item',
description: 'This is an example item',
quantity: 3 };
insertItem(newItemData);
app.listen(PORT, () => {
console.log(`Server is running on PORT ${PORT}`)
})
Output:
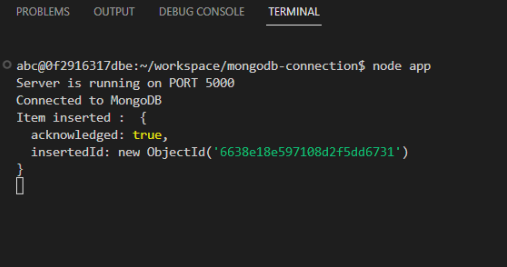
Mongo DB Output
Using Mongoose
Installing Mongoose in your Node.js project using npm. Connect your MongoDB database by providing the MongoDB connection URI. Mongoose.connect method.
Steps to Create Application and Install Required Modules
Step 1. Project Structure : Make a folder named 'mongoose-connection' and navigate to it using this command.
mkdir mongoose-connection
cd mongoose-connection
Step 2. Install Module : Install required module like mongoose , express.
npm i mongoose express dotenv
Updated dependencies in package.json file
"dependencies": {
"express": "^4.19.2",
"mongoose": "^8.3.3",
}
Example: This example demonstrates connection to database using Mongoose.
// server.js
const mongoose = require('mongoose')
const DB_URI = 'xxx-xxx'
const dbConnection = () => {
mongoose.connect(DB_URI);
console.log('Database is successfully connected!')
}
// Define a schema
const itemSchema = new mongoose.Schema({
name: String,
description: String,
quantity: Number
});
// Define a model
const Item = mongoose.model('Item', itemSchema)
module.exports = {
Item, dbConnection
}
// app.js
const express = require('express');
const app = express();
const { Item, dbConnection } = require('./server')
const PORT = process.env.PORT || 8000;
//
app.use(express.json())
dbConnection();
async function Inserting() {
// Example of creating a new document
const newItem = new Item({ name: 'Example Item',
description: 'This is an example item',
quantity: 3 });
await newItem.save()
console.log(newItem)
}
Inserting();
app.listen(PORT, () => {
console.log(`Server is running on ${PORT}`)
})
Output:
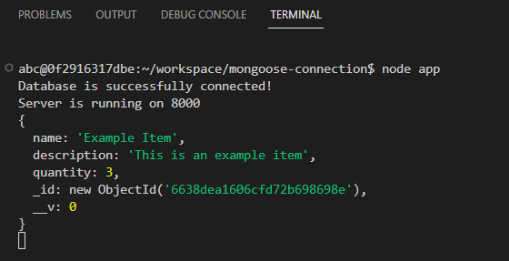
Mongoose Output
MongoDB Output:
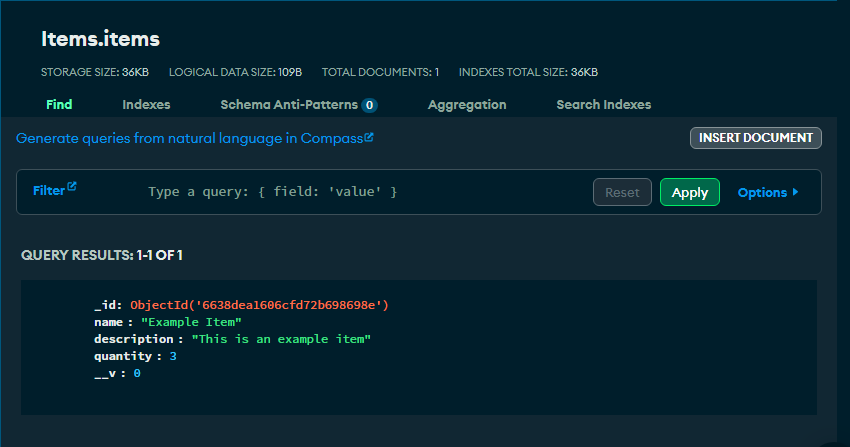
Output at Mongo Database