In this article, we will read about how to add data from Queryset into Templates in Django Python. Data presentation logic is separated in Django MVT(Model View Templates) architecture. Django makes it easy to build web applications with dynamic content. One of the powerful features of Django is fetching data from a database and show on web pages.
Querysets serve as a powerful tool for retrieving data from databases and integrating data from the database. Integrating Querysets data into the templates is essential for displaying the dynamic data on the web pages.
Add Data from Queryset into Templates in Python Django
Below is the implementation to add data from the query set into templates in Django and Python:
Starting the Project Folder
To start the project use this command
django-admin startproject library
cd library
To start the app use this command
python manage.py startapp book
Now add this app to the ‘settings.py’
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'book'
]
File Structure
.png)
Setting Necessary Files
models.py : After the initial project setup, we create a Book Model inside the book app. Book models have price, name, and author fields.
# Create your models here.
# book/models.py
from django.db import models
class Book(models.Model):
name = models.CharField(max_length=100)
author = models.CharField(max_length=100)
price = models.IntegerField()
def __str__(self):
return self.name
views.py : Django code defines a view function named All_Book
that fetches all Book
objects from the database using Django's ORM (Book.objects.all()
) and passes them to a template named 'index.html' via the render
function, making them available as queryset
in the template.
# Create your views here.
# book/views.py
from django.shortcuts import render
from .models import Book
def All_Book(request):
queryset = Book.objects.all()
return render(request, 'index.html', {'queryset': queryset})
library/urls.py: Below, are the Project urls.py file.
from django.contrib import admin
from django.urls import path
from book.views import *
urlpatterns = [
path('admin/', admin.site.urls),
path('', All_Book),
]
admin.py : Here we are registering our models.
# admin.py
from django.contrib import admin
from .models import Book
admin.site.register(Book)
Creating GUI
index.html : HTML and CSS code creates a webpage layout for a book store, featuring a centered container with a heading "My Book Store" and a list of books with their names and authors, likely to be populated dynamically using a templating language like Django's template engine (indicated by {% for item in queryset %}
).
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Book store</title>
</head>
<body>
<h1>My Book</h1>
<ul>
{% for item in queryset %}
<li>{{ item.name }} - {{ item.author }}</li>
{% endfor %}
</ul>
</body>
</html>
Deployment of the Project
Run these commands to apply the migrations:
python3 manage.py makemigrations
python3 manage.py migrate
Run the server with the help of following command:
python3 manage.py runserver
Output:
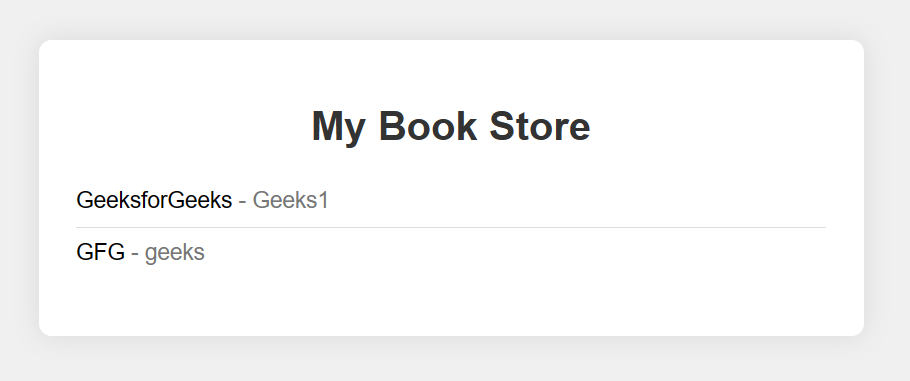
Add Data from Queryset into Templates in Django Python