Explore the componentDidCatch lifecycle method in React, designed to manage errors within the component tree effectively. Discover its features, including error containment, graceful handling, and access to error details, through practical demonstrations. Harness the power of error boundaries to fortify your React applications against unexpected errors and ensure a smoother user experience.
Table of Content
What is the componentDidCatch lifecycle method in the context of error boundaries?
In React, the componentDidCatch lifecycle method is pivotal for managing errors within the component tree. Specifically, when an error occurs during the rendering process of a component or its children, React invokes componentDidCatch. This method acts as a boundary, catching and encapsulating errors to prevent them from propagating and breaking the entire application. Developers can implement componentDidCatch to gracefully handle errors, providing fallback UIs or logging error details, thus ensuring a smoother user experience and enhancing application robustness.
Why is it used?
- Error Containment: componentDidCatch helps in containing errors within specific components, preventing them from crashing the entire application.
- Graceful Error Handling: It allows developers to implement fallback UIs or error boundaries to display user-friendly messages when errors occur, improving the overall user experience.
- Preventing Error Propagation: Errors caught by componentDidCatch are not propagated to the entire component tree, ensuring that other parts of the application remain functional.
- Logging and Reporting: Developers can log error details or report them to external services for analysis, debugging, and monitoring application health.
- Improved Application Stability: By handling errors at the component level, componentDidCatch contributes to the stability and robustness of React applications, minimizing disruptions caused by unexpected errors.
- Encapsulation of Error Handling Logic: It encapsulates error handling logic within individual components, promoting modular and maintainable codebases.
- Enhanced Debugging: Developers can use componentDidCatch to capture error information and debug issues more effectively, speeding up the troubleshooting process during development and testing..
Features of componentDidCatch Lifecycle Method
- Error Boundary Integration: Utilize
componentDidCatch
in React error boundary components. - Error Handling: Gracefully manage errors during rendering to prevent application crashes.
- Local Error Handling: Confined to the error boundary component for specific error management.
- Access to Error Information: Receives
error
anderrorInfo
providing context like stack traces. - State Management: Update component state to reflect error occurrence for handling.
- Fallback Rendering: Render alternate UI to inform users about errors.
- Error Reporting: Utilize componentDidCatch for error tracking and logging in production.
Setup React Environment:
Create a new directory for your React project.
npx create-react-app error-boundary-demo
Exaplanation:
- Display a fallback UI when an error occurs in the ChildComponentWithError component.
- This code showcases the implementation of an error boundary in React using the componentDidCatch lifecycle method.
- The ErrorBoundary class component tracks errors with state and logs them using getDerivedStateFromError and componentDidCatch methods.
- In case of an error, it renders a fallback UI. ChildComponentWithError simulates an error scenario. App component wraps ChildComponentWithError with ErrorBoundary for error handling.
Example: Below is an example of componentDidCatch lifecycle method in the context of error boundaries.
import React, { Component } from 'react';
// ErrorBoundary component
class ErrorBoundary extends Component {
constructor(props) {
super(props);
this.state = { hasError: false };
}
// Update state to indicate an error has occurred
static getDerivedStateFromError(error) {
return { hasError: true };
}
// Log the error to an error reporting service
componentDidCatch(error, errorInfo) {
console.error(
'Error caught by ErrorBoundary:', error, errorInfo);
}
render() {
if (this.state.hasError) {
// Fallback UI to display when an error occurs
return <h1>
Something went wrong. Please try again later.
</h1>;
}
// Render the children components normally
return this.props.children;
}
}
// ChildComponentWithError component
class ChildComponentWithError extends Component {
render() {
try {
// Simulating an error
throw new Error('An error occurred in ChildComponentWithError');
} catch (error) {
// Log the error
console.error(
'Error in ChildComponentWithError:', error);
// Fallback UI to display when an error occurs
return <h1>
Something went wrong. Please try again later.
</h1>;
}
}
}
// Usage of ErrorBoundary
class App extends Component {
render() {
return (
<ErrorBoundary>
<div>
<h1>Hello, World!</h1>
{/* Simulating an error */}
<ChildComponentWithError />
</div>
</ErrorBoundary>
);
}
}
export default App;
Output:
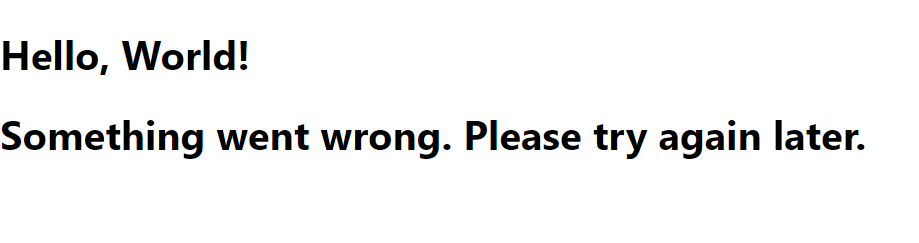
Error boundary in action: Displaying a fallback UI when an error occurs in the ChildComponentWithError component.