A Cellular Automaton is a discrete model similar to any other automaton which has its own start state(s) and a set of rules.
A cellular automaton is a model of a system of "cell" objects with the following characteristics :
- The cells live on a grid which can be either 1D or even multi-dimensional
- Each cell has a state. The number of state possibilities is typically finite. The simplest example has the two possibilities of 1 and 0
- Each cell has a neighbourhood and it is typically a list of adjacent cells
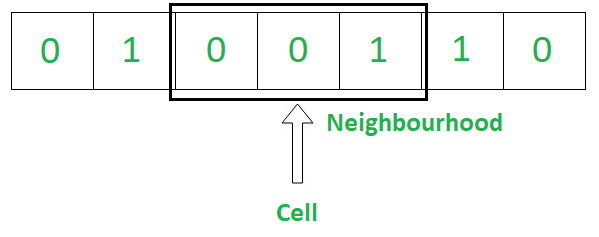
Working principle of Cellular Automata?
The working principle of the cellular automata is shown below:
- An initial state is selected by assigning a state for each cell.
- A new generation is created, according to some fixed rule that determines the new state of each cell in terms of:
- The current state of the cell.
- The states of the cells in its neighbourhood.
- Hence, a new state is calculated by looking at all previous neighbouring states.
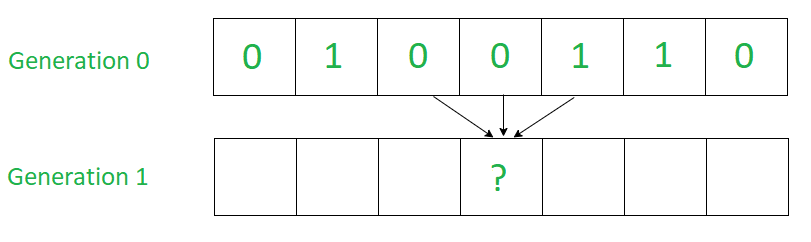
Examples of Cellular Automata:
2. Rule 90:
Rule 90 is an elementary cellular automaton that consists of a one-dimensional array of cells, each of which can hold either a 0 or a 1 value. Each cell is the exclusive or(XOR) of its two neighbours.
Current State | 111 | 011 | 101 | 100 | 011 | 010 | 001 | 000 |
Next State | 0 | 1 | 0 | 1 | 1 | 0 | 1 | 0 |
If we concatenate the Next State into a single binary number and convert it to decimal (01011010)2, it becomes 90, hence we get the name Rule 90.
3. When the initial state has a single nonzero cell, this diagram has the appearance of the Sierpiński triangle.
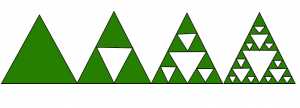
Implementation of Sierpiński triangle:
// C++ code to implement Sierpinski Triangle
#include <bits/stdc++.h>
using namespace std;
// Length of the string used as a "Seed"
#define LENGTH 34
// How many lines the program will generate
int n = 15;
// All the patterns possible
vector<vector<int> > rules = { { 0, 0, 0 }, { 0, 0, 1 },
{ 0, 1, 0 }, { 0, 1, 1 },
{ 1, 0, 0 }, { 1, 0, 1 },
{ 1, 1, 0 }, { 1, 1, 1 } };
// Utility Function to Print Rules
void printRules()
{
int i, j;
cout << "The rules of Rule 90 Cellular Automaton"
" are as follows: \n";
for (i = 0; i < 8; i++) {
cout << "\t\tRule " << i + 1 << ": ";
for (j = 0; j < 3; j++)
cout << rules[i][j] << " ";
cout << "-> sets cell to: "
<< (rules[i][0]
^ rules[i][2]) << "\n";
}
}
// Print the Current State
void outState(vector<int> s)
{
cout << "\t\t";
for (int i = 0; i < LENGTH; i++) {
if (s[i] == 1)
cout << "\u25A0";
else if (s[i] == 0)
cout << " ";
}
cout << "\n";
}
// Function to print the triangle
void utilize()
{
// Initialize starting state
// to sierpinski triangle
vector<int> sierpinski(LENGTH);
vector<int> updateState(LENGTH);
sierpinski[(LENGTH) / 2 - 1] = 1;
// Print Sierpinski Triangle
// initial String
outState(sierpinski);
// Loop to generate/update the state
// and update state arrays
for (int i = 0; i < n; i++)
{
// Erase the old state
updateState.assign(LENGTH, 0);
// Create the new state
for (int j = 1; j < LENGTH - 1; j++)
{
int f1 = sierpinski[j - 1];
int f2 = sierpinski[j];
int f3 = sierpinski[j + 1];
// Create an array with the
// current pattern
vector<int> current;
current.push_back(f1);
current.push_back(f2);
current.push_back(f3);
// XOR the current state's neighbours
// to set the cell's value
// in Update State
updateState[j] = current[0] ^ current[2];
}
// Update the current state
sierpinski.assign(updateState.begin(),
updateState.end());
// Print the new current state
outState(sierpinski);
}
}
// Driver code
int main()
{
// Print out the rules for
// rule 90 cellular Automaton
printRules();
cout << "\n\t\t\tSIERPINSKI TRIANGLE\n\n";
utilize();
return 0;
}
import java.util.ArrayList;
import java.util.List;
public class SierpinskiTriangle {
// Length of the string used as a "Seed"
final static int LENGTH = 34;
// How many lines the program will generate
static int n = 15;
// All the patterns possible
final static List<List<Integer>> rules = List.of(
List.of(0, 0, 0),
List.of(0, 0, 1),
List.of(0, 1, 0),
List.of(0, 1, 1),
List.of(1, 0, 0),
List.of(1, 0, 1),
List.of(1, 1, 0),
List.of(1, 1, 1)
);
// Utility Function to Print Rules
static void printRules() {
System.out.println("The rules of Rule 90 Cellular Automaton are as follows: ");
for (int i = 0; i < 8; i++) {
System.out.print("\t\tRule " + (i + 1) + ": ");
for (int j = 0; j < 3; j++) {
System.out.print(rules.get(i).get(j) + " ");
}
System.out.println("-> sets cell to: " + (rules.get(i).get(0) ^ rules.get(i).get(2)));
}
}
// Print the Current State
static void outState(List<Integer> s) {
System.out.print("\t\t");
for (int i = 0; i < LENGTH; i++) {
if (s.get(i) == 1)
System.out.print("*");
else if (s.get(i) == 0)
System.out.print(" ");
}
System.out.println();
}
// Function to print the triangle
static void utilize() {
// Initialize starting state to sierpinski triangle
List<Integer> sierpinski = new ArrayList<>();
List<Integer> updateState = new ArrayList<>();
for (int i = 0; i < LENGTH; i++) {
sierpinski.add(0);
updateState.add(0);
}
sierpinski.set((LENGTH) / 2 - 1, 1);
// Print Sierpinski Triangle initial String
outState(sierpinski);
// Loop to generate/update the state and update state arrays
for (int i = 0; i < n; i++) {
// Erase the old state
// Create the new state
for (int j = 1; j < LENGTH - 1; j++) {
int f1 = sierpinski.get(j - 1);
int f2 = sierpinski.get(j);
int f3 = sierpinski.get(j + 1);
// XOR the current state's neighbors to set the cell's value in Update State
updateState.set(j, f1 ^ f3);
}
// Update the current state
sierpinski = new ArrayList<>(updateState);
// Print the new current state
outState(sierpinski);
}
}
// Driver code
public static void main(String[] args) {
// Print out the rules for rule 90 cellular Automaton
printRules();
System.out.println("\n\t\t\tSIERPINSKI TRIANGLE\n");
utilize();
}
}
# Length of the string used as a "Seed"
LENGTH = 34
# How many lines the program will generate
n = 15
# All the patterns possible
rules = [
[0, 0, 0], [0, 0, 1],
[0, 1, 0], [0, 1, 1],
[1, 0, 0], [1, 0, 1],
[1, 1, 0], [1, 1, 1]
]
# Utility Function to Print Rules
def print_rules():
print("The rules of Rule 90 Cellular Automaton are as follows:")
for i in range(8):
print(f"\t\tRule {i + 1}: {rules[i]} -> sets cell to: {rules[i][0] ^ rules[i][2]}")
# Print the Current State
def out_state(s):
print("\t\t", end="")
for i in range(LENGTH):
if s[i] == 1:
print("\u25A0", end="")
elif s[i] == 0:
print(" ", end="")
print()
# Function to print the triangle
def utilize():
# Initialize starting state to Sierpinski triangle
sierpinski = [0] * LENGTH
update_state = [0] * LENGTH
sierpinski[(LENGTH) // 2 - 1] = 1
# Print Sierpinski Triangle initial String
out_state(sierpinski)
# Loop to generate/update the state and update state arrays
for _ in range(n):
# Erase the old state
update_state = [0] * LENGTH
# Create the new state
for j in range(1, LENGTH - 1):
f1, f2, f3 = sierpinski[j - 1], sierpinski[j], sierpinski[j + 1]
# Create an array with the current pattern
current = [f1, f2, f3]
# XOR the current state's neighbors to set the cell's value in Update State
update_state[j] = current[0] ^ current[2]
# Update the current state
sierpinski = update_state.copy()
# Print the new current state
out_state(sierpinski)
# Driver code
if __name__ == "__main__":
# Print out the rules for Rule 90 Cellular Automaton
print_rules()
print("\n\t\t\tSIERPINSKI TRIANGLE\n\n")
utilize()
// Length of the string used as a "Seed"
const LENGTH = 34;
// How many lines the program will generate
const n = 15;
// All the patterns possible
const rules = [
[0, 0, 0], [0, 0, 1],
[0, 1, 0], [0, 1, 1],
[1, 0, 0], [1, 0, 1],
[1, 1, 0], [1, 1, 1]
];
// Utility Function to Print Rules
function print_rules() {
console.log("The rules of Rule 90 Cellular Automaton are as follows:");
for (let i = 0; i < 8; i++) {
console.log(`\t\tRule ${i + 1}: ${rules[i]} -> sets cell to: ${rules[i][0] ^ rules[i][2]}`);
}
}
// Print the Current State
function out_state(s) {
let output = "\t\t";
for (let i = 0; i < LENGTH; i++) {
if (s[i] === 1) {
output += "\u25A0";
} else if (s[i] === 0) {
output += " ";
}
}
console.log(output);
}
// Function to print the triangle
function utilize() {
// Initialize starting state to Sierpinski triangle
let sierpinski = Array(LENGTH).fill(0);
let update_state = Array(LENGTH).fill(0);
sierpinski[Math.floor(LENGTH / 2) - 1] = 1;
// Print Sierpinski Triangle initial String
out_state(sierpinski);
// Loop to generate/update the state and update state arrays
for (let _ = 0; _ < n; _++) {
// Erase the old state
update_state = Array(LENGTH).fill(0);
// Create the new state
for (let j = 1; j < LENGTH - 1; j++) {
let f1 = sierpinski[j - 1], f2 = sierpinski[j], f3 = sierpinski[j + 1];
// Create an array with the current pattern
let current = [f1, f2, f3];
// XOR the current state's neighbors to set the cell's value in Update State
update_state[j] = current[0] ^ current[2];
}
// Update the current state
sierpinski = [...update_state];
// Print the new current state
out_state(sierpinski);
}
}
// Driver code
(function main() {
// Print out the rules for Rule 90 Cellular Automaton
print_rules();
console.log("\n\t\t\tSIERPINSKI TRIANGLE\n\n");
utilize();
})();
Output
The rules of Rule 90 Cellular Automata are as follows: Rule 1: 0 0 0 -> sets cell to: 0 Rule 2: 0 0 1 -> sets cell to: 1 Rule 3: 0 1 0 -> sets cell to: 0 Rule 4: 0 1 1 -> sets cell to: 1 Rule 5: 1 0 0 -> sets cell to: 1 Rule 6: 1 0 1 -> sets cell to: 0 Rule 7: 1 1 0 -> sets cell to: 1 Rule 8: 1 1 1 -> sets cell to: 0 SIERPINSKI TRIANGLE ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■ ■