Using Web Workers for background processing in React Hooks applications offers a powerful solution to handle computationally intensive tasks without blocking the main UI thread. With React Hooks, developers can easily integrate Web Workers into their applications to perform tasks such as data processing, image manipulation, or any other heavy computation without impacting user interaction.
Introduction to Web Workers
Web Workers offer a solution to the single-threaded nature of JavaScript by enabling concurrent execution in the browser environment. They allow developers to run scripts in the background, separate from the main UI thread, enabling tasks like complex computations, data processing, and I/O operations to be performed without blocking the user interface.
In React Hooks applications, leveraging Web Workers can enhance performance and responsiveness by offloading intensive tasks to separate threads, thereby improving the overall user experience.
Features
These are the features of utilizing Web Workers for Background Processing in React Hooks Applications
- Improved Performance: Offloading heavy computational tasks to Web Workers allows for improved performance by executing these tasks in the background, freeing up the main thread for other operations.
- Responsive User Interface: By executing intensive tasks in the background, the user interface remains responsive and doesn't freeze or become unresponsive during heavy processing.
- Concurrency: Web Workers enable concurrent execution of tasks, allowing multiple tasks to run simultaneously without blocking the main thread.
- Modular Architecture: Integrating Web Workers with React Hooks applications follows a modular architecture, making it easy to manage and maintain codebases.
- Scalability: With Web Workers, applications can scale more effectively to handle complex computations or large datasets without sacrificing performance.
- Error Handling: Proper error handling mechanisms can be implemented to manage errors and exceptions that may occur during background processing, ensuring robustness and reliability.
- Cross-Browser Compatibility: Web Workers are supported across all major browsers, ensuring cross-browser compatibility for applications leveraging this technology.
- Real-Time Updates: Applications can receive real-time updates and notifications from background tasks, allowing for dynamic and interactive user experiences.
- Optimization: Web Workers can optimize performance by distributing tasks across multiple CPU cores, leveraging the full processing power of the user's device.
- Flexibility: Web Workers offer flexibility in terms of where and how tasks are executed, providing developers with greater control over application performance and resource utilization.
Understanding Background Processing
Background processing refers to the execution of tasks that do not directly interact with the user interface or block the main thread of execution. In React applications, background processing is crucial for handling computationally intensive tasks, such as data fetching, image processing, or heavy calculations, without affecting the responsiveness of the user interface. By offloading these tasks to separate threads or processes, background processing ensures that the application remains smooth and responsive, providing a seamless user experience even when handling complex operations. Utilizing techniques like Web Workers enables developers to implement background processing effectively in React applications, enhancing performance and scalability.
Error Handling
Error handling in Web Workers is crucial to ensure robustness and reliability in background processing tasks. When working with Web Workers in React Hooks applications, consider the following error handling strategies:
- Try-Catch Blocks: Wrap the code inside the Web Worker with try-catch blocks to catch any synchronous errors that may occur during execution.
- Error Event Listener: Set up an error event listener (onerror) within the Web Worker to capture any uncaught errors or exceptions. This allows you to handle asynchronous errors gracefully and prevent them from crashing the application.
- Error Reporting: Implement error reporting mechanisms to log and track errors that occur within Web Workers. This can include logging errors to the console, sending error reports to a server, or displaying error messages to users.
- Graceful Degradation: Design your application to gracefully degrade when errors occur in Web Workers. Provide fallback mechanisms or alternative approaches for handling tasks in case of failure.
- Testing and Debugging: Test your Web Worker code thoroughly and use debugging tools to identify and resolve errors during development. Tools like Chrome DevTools offer debugging support for Web Workers, allowing you to inspect variables, set breakpoints, and debug code effectively.
- By implementing these error handling strategies, you can ensure that your React Hooks applications remain stable and responsive even when performing intensive tasks in the background using Web Workers.
Significance in React Applications
In React applications, heavy tasks such as complex calculations or data processing can sometimes lead to UI freezes or unresponsiveness. This is because JavaScript is single-threaded, and performing intensive operations on the main thread can impact user interaction. By leveraging Web Workers, we can execute these tasks concurrently in the background, allowing the main thread to remain responsive.
Performing Heavy Tasks in the Background
In this section, we'll delve into the practical example provided in the code snippet to demonstrate how heavy computational tasks can be offloaded to Web Workers for improved performance. The provided code example showcases the utilization of Web Workers to perform heavy computational tasks in the background. By moving these tasks to a separate thread, we prevent them from blocking the main UI thread, ensuring a smooth user experience.
Integrating Web Workers with React Hooks
In the following code example, we demonstrate how to integrate Web Workers into a React application using React Hooks. Let's break down the steps involved:
- Creating a Web Worker Inline: Instead of creating a separate file for the Web Worker logic, we generate it inline within the runWorker() function. This is achieved by creating a Blob containing the Web Worker code and creating a new Worker instance using the URL.createObjectURL() method.
- Sending Data to the Web Worker:Once the Worker instance is created, we send data to the Web Worker using the postMessage() method. In this example, we send the number 10 to the Web Worker, which will then perform background processing on it.
- Listening for Messages: We set up an event listener using the onmessage() property to listen for messages sent from the Web Worker. When the Web Worker finishes processing the data and sends back the result using the postMessage() method, we capture it in the onmessage event handler.
- Updating Component State: Inside the onmessage() event handler, we update the component state using the setResult() function provided by the useState hook. This causes the component to re-render with the updated result, which is then displayed in the UI.
By following this approach, we can leverage the power of Web Workers for background processing tasks within a React application, enhancing performance and responsiveness without blocking the main thread.
Example: In the given code, upon clicking the "Run Worker" button, a Web Worker is instantiated inline with a simple background processing logic. This logic doubles the value received from the main thread and sends it back as a result. This serves as a basic illustration of how heavy tasks can be executed in the background without affecting the main thread's performance. If you want you can have the required styling and even this is given in the following code example as "App.css".
First, create a new React project named "web-worker-example" using the following commands:
npx create-react-app web-worker-example
cd web-worker-example
Next, replace the contents of the "App.js" file located in the "src" directory with the code snippet below:
/* App.css */
.App {
text-align: center;
}
.App-header {
background-color: #282c34;
min-height: 100vh;
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
font-size: calc(10px + 2vmin);
color: white;
}
button {
margin-top: 20px;
padding: 10px 20px;
font-size: 16px;
cursor: pointer;
background-color: #61dafb;
border: none;
border-radius: 5px;
}
button:hover {
background-color: #45aaf2;
}
// Filename - App.js
import React, { useState } from 'react';
import './App.css';
const App = () => {
const [result, setResult] = useState(null);
const runWorker = () => {
const worker = new Worker(URL.createObjectURL(
new Blob([`
onmessage = function(e) {
const result = e.data * 2; // Simple background processing
postMessage(result);
};
`], { type: 'application/javascript' })
));
worker.postMessage(10); // Send data to the Web Worker
worker.onmessage = function (e) {
// Listen for message from Web Worker
setResult(e.data); // Update state with result
};
};
return (
<div className="App">
<header className="App-header">
<h1>Web Worker Example</h1>
<button onClick={runWorker}>Run Worker</button>
{result && <p>Result: {result}</p>} {/* Display result */}
</header>
</div>
);
};
export default App;
After saving the changes, start the development server using
npm start
Output:
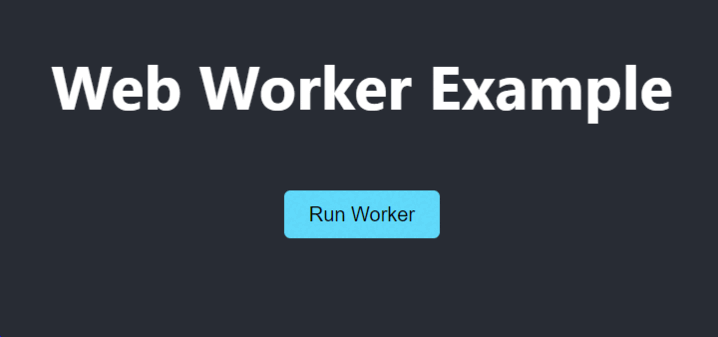
Web application interface showing the 'Run Worker' button and the result of the background processing task.
Real-World Use Cases
Web Workers offer a wide range of use cases in React Hooks applications, including:
- Image Processing: Web Workers can be used to perform image processing tasks such as resizing, cropping, or applying filters to images in the background, improving performance and responsiveness of image-heavy applications.
- Data Processing: Utilize Web Workers for processing large datasets, performing complex calculations, or running data-intensive algorithms without blocking the main UI thread. This is particularly useful for applications dealing with data visualization, analytics, or scientific computations.
- Network Requests: Offload network requests and API calls to Web Workers to prevent blocking the main thread during data fetching operations. This ensures that the UI remains responsive while waiting for network responses.
- Game Development: Implement game logic, physics simulations, or AI algorithms using Web Workers to distribute computation across multiple threads and improve game performance.
- Background Tasks: Use Web Workers for running background tasks such as data synchronization, caching, or periodic updates without interrupting user interaction.
These real-world use cases demonstrate the versatility and effectiveness of Web Workers in enhancing the performance and user experience of React Hooks applications.
Conclusion
Integrating Web Workers with React Hooks applications offers improved performance, responsive interfaces, and enhanced scalability. By offloading intensive tasks to separate threads, developers ensure the main UI thread remains unblocked, resulting in smoother applications. Throughout this article, we explored the significance of Web Workers in background processing tasks, covering error handling, real-world use cases, and best practices. By following these guidelines, developers can optimize performance and deliver seamless user experiences. In summary, consider leveraging Web Workers for background processing tasks in React Hooks applications to achieve better performance, responsiveness, and scalability.