Spring Boot is one of the best frameworks for back-end purposes, and it is mostly used for Web Application development. In this article, we will explain about properties file in Spring Boot using environment variable. The properties file is available in the Resource folder in the Spring Project folder. The Spring Boot properties file is identified by Its logo. The logo is leaf, Mostly the application properties file plays an important role in Spring Boot Application development. The file extension is properties. In this file, we can define different kinds of properties.
Advantages of Properties File:
- For creating a database connection, the Properties File is used and we can define the required configuration details in the Properties File.
- We can able to create different profiles also like dev, QA, production, and other profiles.
- Integration with Spring Environment means we can integrate the required configuration through application.properties.
- Our deployment has become very easy due to the application.properties file.
- The application.properties file provides dynamic behavior to the application while accessing configuration details from this file.
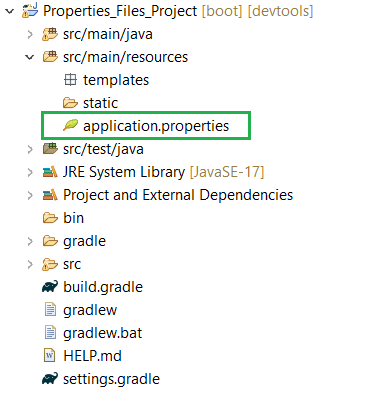
Environment Variable
In Spring Boot, The Environment Variables are variables which are set in Operating System environment and those variables are available to the application in runtime. Mostly the variables are used for configure the various aspects of the application. Spring Boot framework provides lot of annotations to simplify the solution. To access the Environment Variables from the application.properties file, we have one annotation that is @Autowired.
By using Environment object, we can access the Environment variables by using getProperty() method. This method can able to access the environment variables from the application.properties File.
@Autowired
private Environment environment;
In application.properties file, we have defined two properties to explain how environment variables are work in Spring Boot's Properties Files.
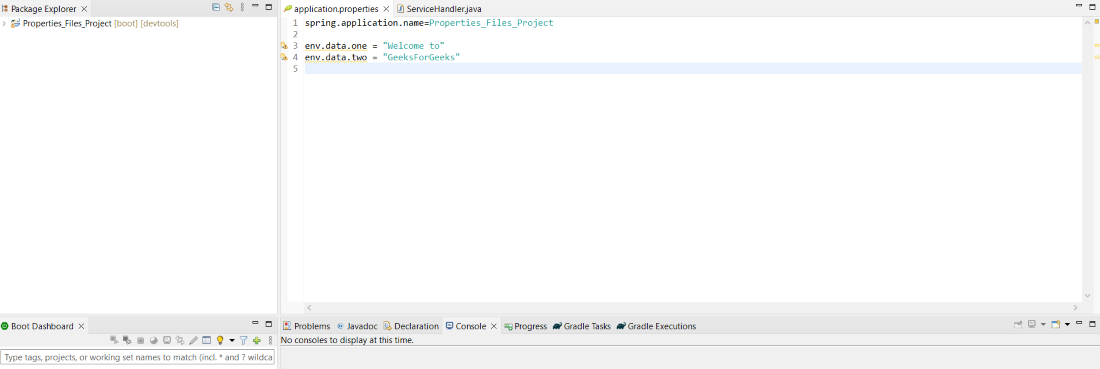
Implementation of Spring Boot's Properties File Using Environment Variables
- In this example, we have created one Spring Boot Starter project.
- After that, created one class for RestController.
- Here, we have used @RestController to created API end points.
- Then we have created one API end point. After this, we have created one displayMessage method to access the Environment Variables in Spring Boot's Properties Files.
- In this method, we access the Environment Variables from Spring Boot's Properties Files by using Environment interface.
- Then, it prints the result.
Main method class:
package com.app;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.core.env.Environment;
// Define the main application class annotated with @SpringBootApplication
@SpringBootApplication
public class PropertiesFilesProjectApplication {
// Autowire the Environment object for accessing environment variables
@Autowired
private Environment env;
// Main method to start the Spring Boot application
public static void main(String[] args) {
// Run the Spring Boot application
SpringApplication.run(PropertiesFilesProjectApplication.class, args);
}
}
- This is Spring Boot application's main class, annotated with
@SpringBootApplication
, serves as the entry point. - It autowires the
Environment
object to access environment variables, ensuring seamless integration of external configurations. - The
main
method initializes and starts the application usingSpringApplication.run()
.
service handler:
package com.app;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.core.env.Environment;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
// Define a RestController class named ServiceHandler
@RestController
public class ServiceHandler {
// Autowire the Environment object for accessing environment variables
@Autowired
private Environment environment;
// Define a GET mapping for the "/msg" endpoint
@GetMapping("/msg")
// Method to display messages retrieved from environment variables
public void displayMessage() {
// Retrieve message1 from environment variable "env.data.one"
String message1 = environment.getProperty("env.data.one");
// Retrieve message2 from environment variable "env.data.two"
String message2 = environment.getProperty("env.data.two");
// Print message1 to the console
System.out.println("Message One: " + message1);
// Print message2 to the console
System.out.println("Message Two: " + message2);
}
}
- In this Spring Boot application, a
RestController
namedServiceHandler
is defined. - It autowires the
Environment
object to access environment variables. - The
displayMessage()
method is mapped to the "/msg" endpoint using@GetMapping
, retrieving and printing messages retrieved from environment variables "env.data.one" and "env.data.two".
Output:
After running this spring project, we will get the following result in the java console. Below is the console output for reference.
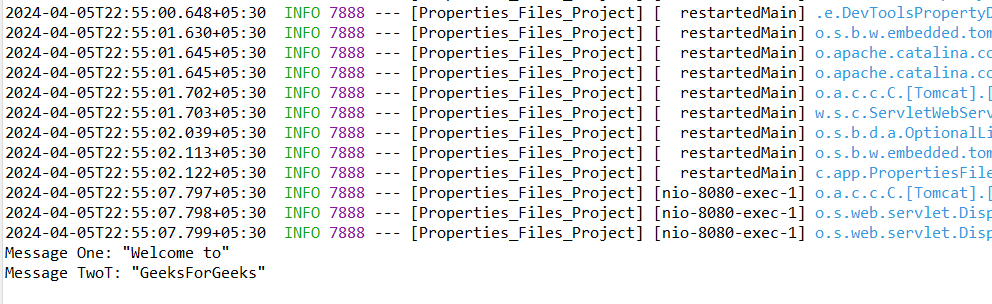