Lazy loading in NextJS is a technique used to improve the performance and loading times of web applications built with the NextJS framework. With lazy loading, components or modules are loaded only when they are needed, rather than upfront when the page is initially rendered.
This means that resources are fetched asynchronously, allowing the initial page load to be faster and reducing the overall bandwidth usage. NextJS provides built-in support for lazy loading through dynamic imports, allowing developers to split their code into smaller chunks and load them on demand.
We will discuss the approach of Lazy Loading in NextJS:
Prerequisites
Steps to Create a NextJS App:
Step 1: Create a NextJS application.
npx create-next-app <-foldername->
Let's say, This will be the procedure, with no changes in dependencies.
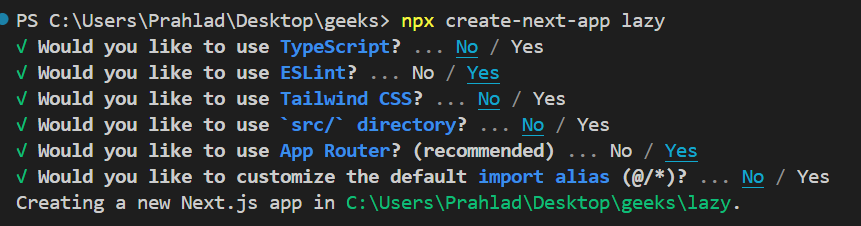
Procedure to make nextjs app with javascript
Step 2: Move to the Project directory
cd <-foldername->
Project Structure:
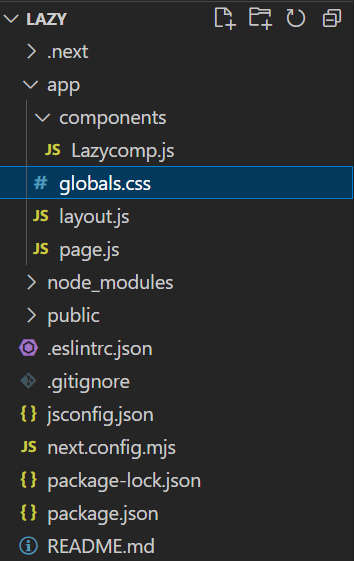
Project Structure
Using Dynamic Imports with next/dynamic
next/dynamic
combines the functionalities of both React.lazy() and Suspense. It operates similarly within both the app and pages directories, facilitating gradual migration.
Example: Below is an example of lazy loading using Dynamic Imports with next/dynamic.
// page.js this is the entry point of application
"use client";
import React, { useState } from "react";
import dynamic from "next/dynamic";
const LazyComp = dynamic(() => import("./components/Lazycomp"), {
loading: () => <h1>Loading...</h1>,
});
function Home() {
const [shown, setShown] = useState(false);
return (
<div style={{ margin: "30px" }}>
<p> GeeksforGeeks lazy loading article in nextjs app</p>
<button
style={{
background: "green", color: "white"
}}
onClick={() => setShown(!shown)}
>
Load Component
</button>
{shown && <LazyComp />}
</div>
);
}
export default Home;
//lazy Component that render dynamically
function Lazycomp() {
return (
<div style={{ margin: "30px" }}>
Lazy loading in Next.js is a technique used to improve the performance and
loading times of web applications built with the Next.js framework. With
lazy loading, components or modules are loaded only when they are needed,
rather than upfront when the page is initially rendered. This means that
resources are fetched asynchronously, allowing the initial page load to be
faster and reducing the overall bandwidth usage. Next.js provides built-in
support for lazy loading through dynamic imports, allowing developers to
split their code into smaller chunks and load them on demand. By
implementing lazy loading, Next.js applications can deliver a smoother and
more responsive user experience, particularly for larger applications with
complex component hierarchies.
</div>
);
}
export default Lazycomp;
Start your application using the following command.
npm run dev
Output:
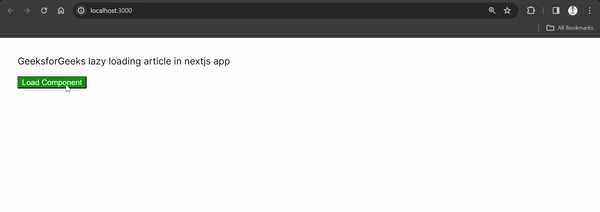
Loading before component rendering
Using React.lazy() with Suspense
Lazy loading with React.lazy() and Suspense in a Next.js app allows for dynamic import of components, improving performance by loading them only when needed. Suspense provides a seamless loading experience for users, making the application more responsive and efficient.
Here are the steps to implement lazy loading using React.lazy() with Suspense.
Example: Below is an example of lazy loading Using React.lazy() with Suspense
// page.js, this is the entry point of application
"use client";
import React, { useState, Suspense } from "react";
const LazyComp = React.lazy(() => import('./components/LazyComponent'));
function Home() {
const [shown, setShown] = useState(false);
return (
<div style={{ margin: "30px" }}>
<p> GeeksforGeeks lazy loading article in nextjs app</p>
<button
style={{ background: "green", color: "white" }}
onClick={() => setShown(!shown)}
>
Load Component
</button>
{shown && <Suspense fallback={<h1>Loading...</h1>}>
<LazyComponent />
</Suspense>}
</div>
);
}
export default Home;
//lazy Component that render dynamically
function Lazycomp() {
return (
<div style={{margin:"30px"}}>
Lazy loading in Next.js is a technique used to improve the performance and
loading times of web applications built with the Next.js framework. With
lazy loading, components or modules are loaded only when they are needed,
rather than upfront when the page is initially rendered. This means that
resources are fetched asynchronously, allowing the initial page load to be
faster and reducing the overall bandwidth usage. Next.js provides built-in
support for lazy loading through dynamic imports, allowing developers to
split their code into smaller chunks and load them on demand. By
implementing lazy loading, Next.js applications can deliver a smoother and
more responsive user experience, particularly for larger applications with
complex component hierarchies.
</div>
);
}
export default Lazycomp;
Start your application using the following command:
npm run dev
Output: