Using JSON in AJAX requests with jQuery is a fundamental aspect of web development. JSON or JavaScript Object Notation, offers a lightweight and structured format for data exchange between a server and a web application. jQuery simplifies this process further through its AJAX functionalities.
We will explore how to effectively use JSON in AJAX requests using jQuery.
Table of Content
Using the $.ajax() Method
In this approach, we are requesting the user information from the Node Server using the $.ajax() method. The server responds with user information that is in JSON format. The $.ajax() method offers versatility in making asynchronous HTTP requests. When the "Fetch User Data" button is clicked, an AJAX request is made to http://localhost:3000/users to fetch user data. If successful, the returned JSON data containing user names and ages is dynamically displayed on the webpage.
Steps to run the code:
npm i express
npm i cors
node server.js
Example: The below example shows how to use JSON in Ajax jQuery Using the $.ajax() Method.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="https://code.jquery.com/jquery-3.7.1.min.js"
integrity=
"sha256-/JqT3SQfawRcv/BIHPThkBvs0OEvtFFmqPF/lYI/Cxo="
crossorigin="anonymous"></script>
<style>
h2 {
color: green;
}
button {
padding: 0.5rem 2rem;
border: 0;
border-radius: 0.5rem;
background-color: green;
color: white;
cursor: pointer;
}
</style>
</head>
<body>
<div>
<h2>GeeksforGeeks</h2>
<button id="fetchUserData">
Fetch User Data
</button>
<div id="userData"></div>
</div>
<script>
$(document).ready(function () {
$('#fetchUserData').click(function () {
$.ajax({
url: 'http://localhost:3000/users',
dataType: 'json',
success: function (data) {
displayUserData(data);
},
error: function () {
$('#userData').text('Error: Unable to fetch user data');
}
});
});
function displayUserData(data) {
var userDataHtml = '<ul>';
$.each(data, function (index, user) {
userDataHtml += '<li>Name: '
+ user.name + ', Age: '
+ user.age + '</li>';
});
userDataHtml += '</ul>';
$('#userData').html(userDataHtml);
}
});
</script>
</body>
</html>
/*server.js*/
const express = require("express");
const app = express();
const port = 3000;
const cors = require("cors");
app.use(cors());
const users = [
{ id: 1, name: "Alice", age: 25 },
{ id: 2, name: "Bob", age: 30 },
{ id: 3, name: "Charlie", age: 35 },
];
app.get("/users", (req, res) => {
res.json(users);
});
app.listen(port, () => {
console.log(`Server running at http://localhost:${port}`);
});
Output:
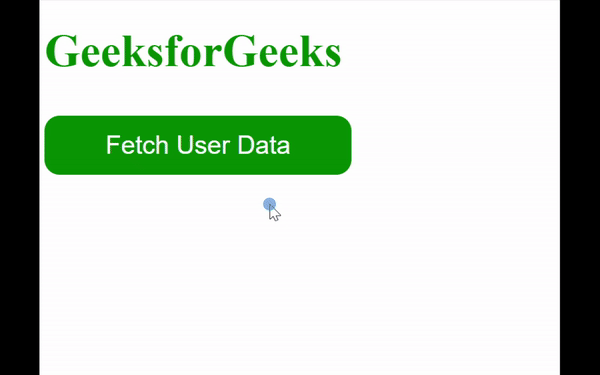
Fetchig user information user $.ajax() method
Using the $.getJSON() Method
Example: In this example, we are requesting the user information from the Node Sever using the $.getJSON() method.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="https://code.jquery.com/jquery-3.7.1.min.js"
integrity="sha256-/JqT3SQfawRcv/BIHPThkBvs0OEvtFFmqPF/lYI/Cxo="
crossorigin="anonymous"></script>
<style>
h2 {
color: green;
}
button {
padding: 0.5rem 2rem;
border: 0;
border-radius: 0.5rem;
background-color: green;
color: white;
cursor: pointer;
}
</style>
</head>
<body>
<div>
<h2>GeeksforGeeks</h2>
<button id="fetchUserData">
Fetch User Data
</button>
<div id="userData"></div>
</div>
<script>
$(document).ready(function () {
$('#fetchUserData').click(function () {
$.getJSON('http://localhost:3000/users',
function (data) {
displayUserDataJSON(data);
})
.fail(function () {
$('#userDataJSON')
.text('Error: Unable to fetch user data');
});
});
function displayUserDataJSON(data) {
var userDataJsonHtml = '<ul>';
$.each(data, function (index, user) {
userDataJsonHtml += '<li>Name: '
+ user.name + ', Age: '
+ user.age + '</li>';
});
userDataJsonHtml += '</ul>';
$('#userData').html(userDataJsonHtml);
}
});
</script>
</body>
</html>
/*server.js*/
const express = require("express");
const app = express();
const port = 3000;
const cors = require("cors");
app.use(cors());
const users = [
{ id: 1, name: "Alice", age: 25 },
{ id: 2, name: "Bob", age: 30 },
{ id: 3, name: "Charlie", age: 35 },
];
app.get("/users", (req, res) => {
res.json(users);
});
app.listen(port, () => {
console.log(`Server running at http://localhost:${port}`);
});
Output:
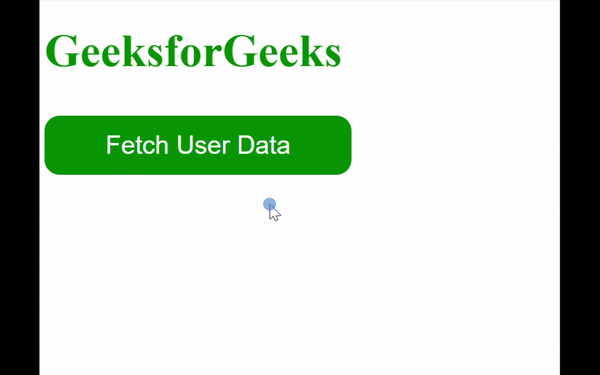
Fetching JSON using $.getJSON() method