In Angular, custom pipes are a powerful tool for transforming data in templates. While simple pipes perform basic transformations, there are cases where you may need to pass parameters to customize the behavior of a pipe. Fortunately, Angular provides mechanisms for passing parameters to custom pipes, allowing you to create more versatile and reusable transformations. In this article, we'll learn how to pass parameters to custom pipes in Angular.
What are Custom Pipes?
Custom pipes in Angular are classes decorated with @Pipe
that implement the PipeTransform
interface. They allow you to define custom transformation logic that can be applied to data in Angular templates. Custom pipes can accept input data and optional parameters to customize their behavior.
Below are the Approaches to create custom pipes:
Table of Content
Approach 1: Using the NgModule decorator
In this approach, you can pass the string value to the pipe's constructor using Angular's dependency injection system and the @Inject decorator by creating a value in the providers array of the NgModule.
Step 1: Generate a new Angular project
ng new string-pipe-demo
Step 2: Generate a new pipe
ng generate pipe reverse
Folder Structure:
Code Example:
//reverse.pipe.ts
import { Pipe, PipeTransform } from '@angular/core';
@Pipe({
name: 'reverse',
pure: true
})
export class ReversePipe implements PipeTransform {
constructor(private suffix: string) { }
transform(value: string): string {
return `${value.split('')
.reverse().join('')}${this.suffix}`;
}
}
// app.module.ts
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppComponent } from './app.component';
import { ReversePipe } from './reverse.pipe';
@NgModule({
declarations: [
AppComponent,
ReversePipe
],
imports: [
BrowserModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
In this example, we're creating a pipe called ReversePipe that takes a string as input, reverses it, and appends a suffix to the end.
Output:
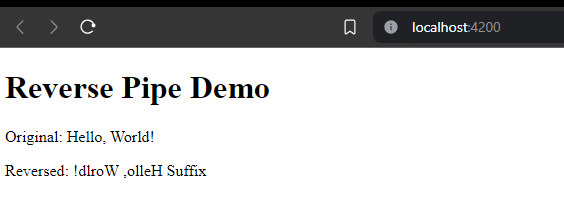
Approach 2: Using Dependency Injection
You can create a service that holds the string value, inject it into the pipe's constructor, and then use that value within the pipe's transformation logic.
Step 1: Create a new Service
ng generate service value-holding
Code Example:
<!-- app.component.html -->
<h1>Reverse Pipe Demo</h1>
<p>Original: {{ 'Hello, World!' }}</p>
<p>Reversed: {{ 'Hello, World!' | reverse}}</p>
//value-holding.service.ts
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root'
})
export class ValueHoldingService {
constructor() { }
suffix = ' Suffix';
}
//reverse.pipe.ts
import { Pipe, PipeTransform } from '@angular/core';
import { SuffixService } from './suffix.service';
@Pipe({
name: 'reverse',
pure: true,
standalone: true
})
export class ReversePipe implements PipeTransform {
constructor(private suffixService: SuffixService) { }
transform(value: string): string {
return `${value.split('')
.reverse().join('')}${this.suffixService.suffix}`;
}
}
In this approach, we create a SuffixService that holds the string value ' Suffix'. We then inject this service into the ReversePipe constructor and use the suffix property within the transform method.
Output: