The JDBC is the Application Programming Interface for Java programming language. In this article, we will learn how to handle duplicate key violations in JDBC by using try and catch block. If we insert new data with an already existing primary key, we get an SQL exception then we need to handle that exception properly. Otherwise, the new data is successfully inserted into the database. Below we have explained this concept with proper examples for both duplicate key violations and successful insertion.
Handle Duplicate key violations in JDBC
To handle duplicate key violations in JDBC. Here, we have used the primary key concept as well as the try-and-catch concept in Java to handle that duplicate key exception.
- In Java, the try-catch concept is used for handling exceptions, and the primary key concept is used for handling duplicate data in the table.
- The primary key is a column that uniquely identifies each row in the table.
- We have created one table with a name book in the database.
- This Table contains four columns namely id, author, name, and price.
- Here, we have used primary for the id column.
- This means If we insert data with an existing ID in the table, then we get an error message like duplicate data found.
Below we provide the table meta information for reference.
Table Structure:
In the below table, we have six columns and four fields:
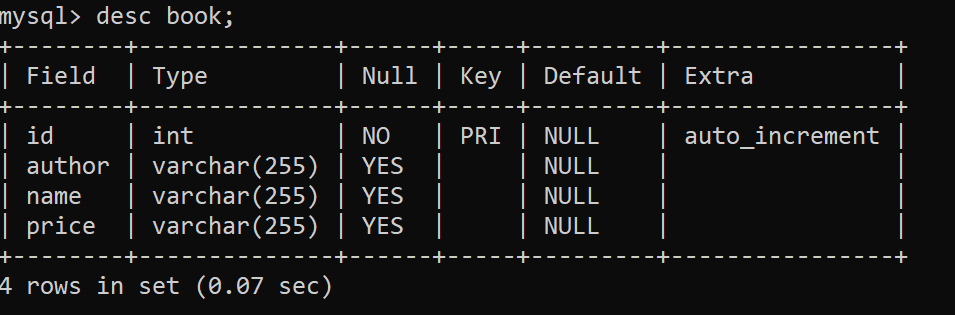
Table Data:
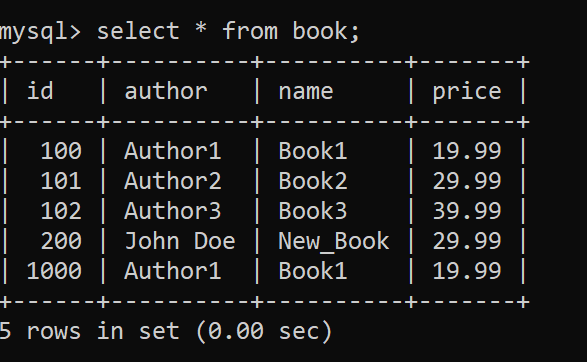
Java Program to handle Duplicate Key Violations in JDBC
Example 1: Duplicate key Violations
In this example, inside class, we define the error code to handle the duplicate key violations in JDBC. By using this error code, we will provide the handling message to the end users for understanding what the exact problem is.
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
import java.sql.Statement;
/**
* This class demonstrates how to insert data into a database table and handle duplicate key violations in JDBC.
*/
public class RetrieveDataExample {
/** The error code for duplicate key violations. */
static final int DUPLICATE_KEY_ERROR_CODE = 1062;
/**
* The main method to execute the JDBC code.
*
* @param args The command-line arguments.
*/
public static void main(String[] args) {
try {
// Load the JDBC driver class
Class.forName("com.mysql.cj.jdbc.Driver");
// Establish database Connection
Connection con = DriverManager.getConnection("jdbc:mysql://localhost:3306/books", "root", "password");
// Create a statement object
Statement stmt = con.createStatement();
// Define the SQL insert statement
String sql = "INSERT INTO book (id, author, name, price) VALUES (100,'John Doe', 'My Book', 19.99)";
// Execute the insert operation
stmt.executeUpdate(sql);
// Print success message
System.out.println("Record inserted successfully.");
// Close the database connection
con.close();
} catch (ClassNotFoundException | SQLException e)
{
// Handle exceptions
if (((SQLException) e).getErrorCode() == DUPLICATE_KEY_ERROR_CODE) {
// Handle duplicate key violation
System.out.println("Duplicate key violation detected. Please change the Id Value");
} else {
// Print exception message
System.out.println(e.getMessage());
}
}
}
}
Output:
Below we can refer to the console output.
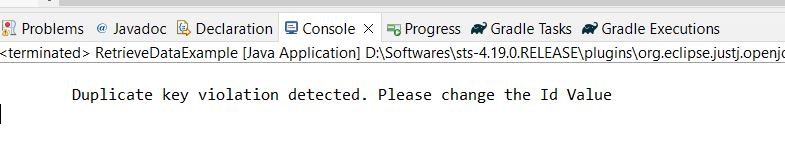
Explanation of the Program:
Here, the error code is 1062 which indicates the error while duplicate key violations is happening.
- In main method, we have started developing required logic. First, we started with database connection with required configuration.
- After successful database connection, we have inserted one new row data into the Table with already existing id in the table.
- Here, we inserted with duplicate key, so we got exception.
- Then, it handles that SQL exception by using error code in the catch block.
- Once SQL exceptions is raised, then we check the exception, then it prints the Error message.
- Otherwise, the new data successfully inserted into table.
Example 2: Successful Insertion
/**
* This class demonstrates how to insert data into a database table and handle duplicate key violations in JDBC.
*/
public class RetrieveDataExample {
/** The error code for duplicate key violations. */
static final int DUPLICATE_KEY_ERROR_CODE = 1062;
/**
* The main method to execute the JDBC code.
*
* @param args The command-line arguments.
*/
public static void main(String[] args) {
try {
// Load the JDBC driver class
Class.forName("com.mysql.cj.jdbc.Driver");
// Establish database Connection
Connection con = DriverManager.getConnection("jdbc:mysql://localhost:3306/books", "root", "password");
// Create a statement object
Statement stmt = con.createStatement();
// Define the SQL insert statement
String sql = "INSERT INTO book (id, author, name, price) VALUES (400,'Gupta', 'My Book', 29.99)";
// Execute the insert operation
stmt.executeUpdate(sql);
// Print success message
System.out.println("Record inserted successfully.");
// Close the database connection
con.close();
} catch (ClassNotFoundException | SQLException e)
{
// Handle exceptions
if (((SQLException) e).getErrorCode() == DUPLICATE_KEY_ERROR_CODE) {
// Handle duplicate key violation
System.out.println("Duplicate key violation detected. Please change the Id Value");
} else {
// Print exception message
System.out.println(e.getMessage());
}
}
}
}
Output:
Below we can see the output in console.

Database Table:
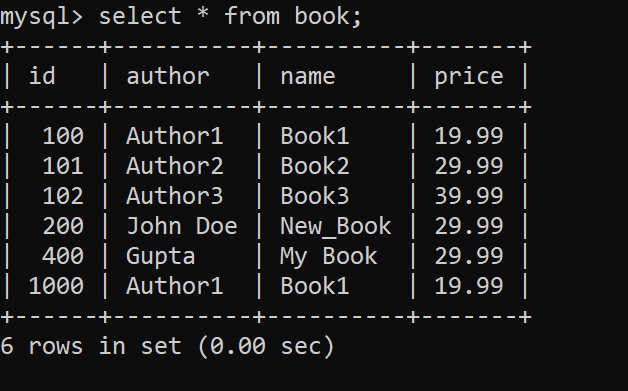
Explanation of the above Program:
- We provide a new row of data with non-existing id in the table.
- This means, we have inserted the data with a new id.
- Then the data is successfully inserted into the Table without any exceptions.
- If any exceptions are raised, then then the catch block logic will handle that exception.