Next.js, a popular React framework, provides powerful features for building server-side rendered (SSR) and statically generated (SSG) applications. Handling query parameters becomes essential when working with dynamic routes and user interactions. This article will explore how to efficiently extract and manage query parameters from the URL in Next.js applications.
Prerequisites:
Understanding Query Parameters
Before diving into implementation, let's understand what query parameters are. Query parameters are key-value pairs appended to the end of a URL, separated by a question mark (?
) and ampersands (&
). For example, in the URL https://example.com/products?id=123&category=electronics
, id
and category
are query parameters.
Steps to Create a NextJS application
Step 1: First create a folder and navigate to that folder.
mkdir nextJS
cd nextJS
Step 2: Now, create a nextJS application using the following command.
npx create-next-app demo
Project Structure:
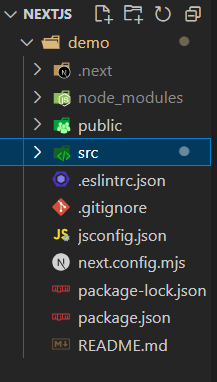
nextJS Structure
The updated dependencies in package.json file will look like this.
"dependencies": {
"react": "^18",
"react-dom": "^18",
"next": "14.2.1"
},
Querying Parameters from URL
Querying parameters from the URL involves extracting key-value pairs from the URL string to access specific data or parameters associated with a web page or application, aiding in dynamic content generation and customization.
Using useRouter
In Next.js, you can use the useRouter hook from the next/router package to access query parameters from the URL. Simply import the useRouter hook in your component and call it to get the router object. Then, you can access the query object from the router object to retrieve query parameters passed in the URL.
Example: Implementation to show how to get query parameters from the URL using useRouter.
//index.js
import { useRouter } from "next/router";
function Dashboard() {
const router = useRouter();
const { query } = router;
const parameter1 = query.postName;
const parameter2 = query.postId;
return (
<main style={{ height: "100vh" }}>
<div>
<p>parameter1 is postName value is: {parameter1}</p>
<p>parameter1 is postId: {parameter2}</p>
</div>
</main>
);
}
export default Dashboard;
Output:

getting params
Using useSearchParams
In Next.js, you can use the useSearchParams hook from the next/navigation package to access query parameters from the URL within functional components. By utilizing the .get method provided by useSearchParams, you can retrieve the value of specific query parameters. Ensure that the parameter names used with .get match those in the URL query string for accurate retrieval.
Example: Implementation to show how to get query parameters from the URL using useSearchParams.
//index.js
import { useSearchParams } from "next/navigation";
function Dashboard() {
const searchParams = useSearchParams();
let property1 = searchParams.get("name");
let property2 = searchParams.get("postId");
return (
<main style={{ height: "100vh" }}>
<div style={{ marginLeft: "10vw" }}>
<h1>Query Parameters:</h1>
<p>parameter 1 value is {property1}</p>
<p>parameter 2 value is {property2}</p>
</div>
</main>
);
}
export default Dashboard;
Output:
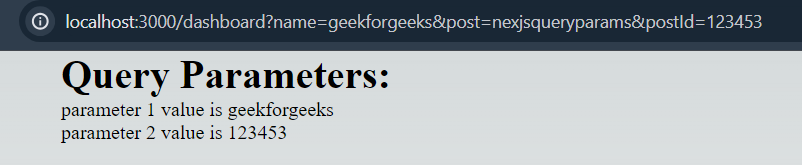
query params using useSearchParams
Example 2: Implementation to show how to get query parameters for multiple unknown params.
//index.js
import { useEffect, useState } from "react";
import { useSearchParams } from "next/navigation";
function Dashboard() {
const searchParams = useSearchParams();
//for multiple queries.
const [queryParams, setQueryParams] = useState([]);
useEffect(() => {
const params = Object.fromEntries(searchParams.entries());
setQueryParams(params);
}, [searchParams]);
return (
<main style={{ height: "100vh" }}>
<div style={{ marginLeft: "10vw" }}>
<h1>Query Parameters:</h1>
<ul>
{Object.entries(queryParams).map(
([param, value]) => (
<li key={param}>
<strong>{param}:</strong> {value}
</li>
))}
</ul>
</div>
</main>
);
}
export default Dashboard;
Output:
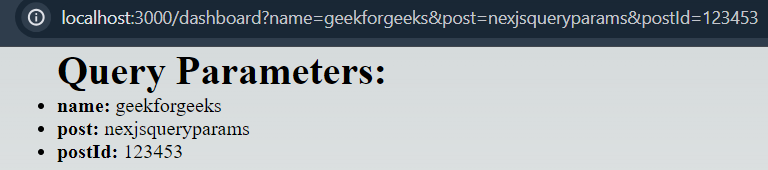
multiple parmeters using useSearchParams
Using getInitialProps
In Next.js, the getInitialProps method is utilized within page components to fetch data and pass it as props before rendering, essential for server-side rendering and pre-rendering pages. It enables data fetching from APIs, server-side rendering, SEO enhancement, and dynamic content generation by handling query parameters efficiently.
Example: Implementation to show how to get query parameters from the URL using getInitialProps.
//index.js
import Head from "next/head";
function Home({ param1, param2 }) {
// Access param1 and param2 here
// For example:
const paramsdata =
`Param1: ${param1}, Param2: ${param2}`;
return (
<>
<Head>
<title>Create Next App</title>
</Head>
<main style={{ height: "100vh" }}>
<p>{paramsdata}</p>
</main>
</>
);
}
Home.getInitialProps = async ({ query }) => {
// Access query parameters from query object
const { param1, param2 } = query;
// Fetch data based on query parameters
// Return data as props
return { param1, param2 };
};
export default Home;
Output:
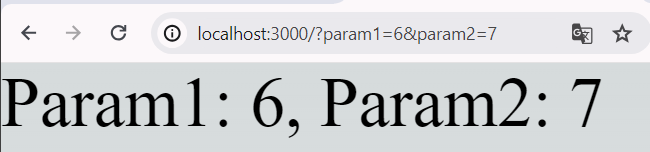
getting queries from getinitialProps
Using getServerSideProps
getServerSideProps in Next.js serves a similar purpose to getInitialProps, allowing data fetching before page rendering. It dynamically fetches data on each request, enhancing server-side rendering, SEO, and performance. With its ability to handle query parameters effectively, it enables dynamic content generation and personalized user experiences.
Example: Implementation to show how to get query parameters from the URL using getServerSideProps.
//index.js
import Head from "next/head";
function Home({ p1, p2 }) {
// Access param1 and param2 here
const paramsData = `Param1: ${p1}, Param2: ${p2}`;
return (
<>
<Head>
<title>Create Next App</title>
</Head>
<main style={{ height: "100vh" }}>
<p>{paramsData}</p>
</main>
</>
);
}
export async function getServerSideProps({ query }) {
// Access query parameters from query object
const { p1, p2 } = query;
// Fetch data based on query parameters
// Returns data as props
return { props: { p1, p2 } };
}
export default Home;
Output:
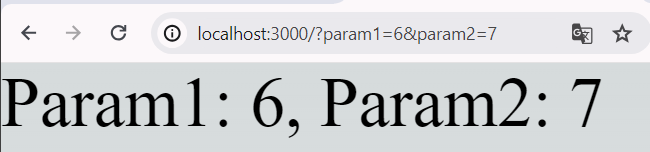
getting queries from getinitialProps
Conclusion
In a Next.js application, the combination of the useRouter hook from the 'next/router' package and the useSearchParams hook from the 'next/navigation' package provides a powerful solution for accessing and processing query parameters from the URL within functional components. These hooks allow us to seamlessly integrate URL parameters in our application logic, enabling dynamic workflows. By utilizing the useRouter hook, developers gain access to the router object, which contains information about the current URL and its query parameters. On the other hand, the useSearchParams hook simplifies the process of extracting and managing query parameters directly from the URL's search string.