Downloading large files in Python can sometimes be a tricky task, especially when efficiency and reliability are crucial. Python's requests
library provides a convenient way to handle HTTP requests, including downloading files. In this article, we will explore how to download large files in Python with Requests
and code examples to demonstrate different approaches.
How To Download Large Files in Python With Requests
Below, are the code examples of how to download large files in Python with Requests.
Example 1: Efficient Large File Download with Python Requests
main.py: In this example, the below code defines a function download_large_file
that takes a URL and a destination path as parameters, then attempts to download a large file from the given URL using the requests
library, downloading it in chunks to conserve memory. If successful, it prints "File downloaded successfully!" Otherwise, it catches and prints any exceptions that occur during the download process.
import requests
def download_large_file(url, destination):
try:
with requests.get(url, stream=True) as response:
response.raise_for_status()
with open(destination, 'wb') as f:
for chunk in response.iter_content(chunk_size=8192):
f.write(chunk)
print("File downloaded successfully!")
except requests.exceptions.RequestException as e:
print("Error downloading the file:", e)
# Example usage
url = 'https://releases.ubuntu.com/20.04.4/ubuntu-20.04.4-desktop-amd64.iso'
save_path = 'ubuntu-20.04.4-desktop-amd64.iso'
download_large_file(url, save_path)
Output
File donwloaded successfully
After downloading file
Example 2:Download Large File in Python Using shutil With Requests
main.py : In this example, below code efficiently downloads large files using the requests
library and saves them to the specified destination using shutil.copyfileobj()
. It streams the file content to avoid loading it entirely into memory, making it suitable for handling large files. Simply provide the URL of the file to download and the destination path.
import requests
import shutil
def download_large_file(url, destination):
try:
response = requests.get(url, stream=True)
with open(destination, 'wb') as out_file:
shutil.copyfileobj(response.raw, out_file)
print("File downloaded successfully!")
except requests.exceptions.RequestException as e:
print("Error downloading the file:", e)
# Example usage
url = 'https://releases.ubuntu.com/20.04.4/ubuntu-20.04.4-desktop-amd64.iso'
save_path = 'ubuntu-20.04.4-desktop-amd64.iso'
download_large_file(url, save_path)
Output
File donwloaded successfully
After downloading file
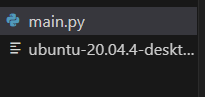
Conclusion
In conclusion, Downloading large files in Python using the requests
library is a straightforward task, thanks to its convenient API. In this article, we explored three different approaches to download large files efficiently: basic file download, resumable download, and progress monitoring. Depending on your specific requirements, you can choose the most suitable approach for your application. With these techniques at your disposal, you can handle large file downloads in Python with ease and reliability.