In Angular, when you're working on a component, you use TypeScript files (.ts) to write the code that controls how the component behaves. On the other hand, HTML files (.html) handle how the user interface looks and gets displayed.
If you want to show a variable from the TypeScript file in the HTML file, you have to use something called data binding. Data binding basically connects the properties of your component to what gets shown on the screen, making sure everything stays in sync.
Data Binding
Data binding is a fundamental concept in Angular that allows you to pass data between the component and the view. There are four different types of data binding in Angular:
- Interpolation: Allows you to display component properties in the HTML template using the double curly brace syntax {{ }}.
- Property Binding: Allows you to bind component properties to HTML element properties.
In this article, we'll focus on interpolation, which is the most common way to display variables from the .ts file in the .html file.
Steps to Create Angular Application
Follow these steps to create an Angular application and implement the approaches mentioned above:
Step 1: Create a new Angular project
Open your terminal or command prompt and run the following command to create a new Angular project:
ng new my-app
Folder Structure:
Dependencies
"dependencies": {
"@angular/animations": "^17.2.0",
"@angular/common": "^17.2.0",
"@angular/compiler": "^17.2.0",
"@angular/core": "^17.2.0",
"@angular/forms": "^17.2.0",
"@angular/platform-browser": "^17.2.0",
"@angular/platform-browser-dynamic": "^17.2.0",
"@angular/platform-server": "^17.2.0",
"@angular/router": "^17.2.0",
"@angular/ssr": "^17.2.3",
"express": "^4.18.2",
"rxjs": "~7.8.0",
"tslib": "^2.3.0",
"zone.js": "~0.14.3"
}
Approach 1: Interpolation
To display a variable from the .ts file in the .html file using interpolation, you need to wrap the variable name with double curly braces {{ }}.
Syntax:
<p>{{ variableName }}</p>
Code Example:
<!-- app.component.html -->
<h1>{{ title }}</h1>
<p>Hello, {{ name }}!</p>
//app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'Angular Data Binding';
name = 'John Doe';
}
To start the application run the following command.
ng serve
Output:
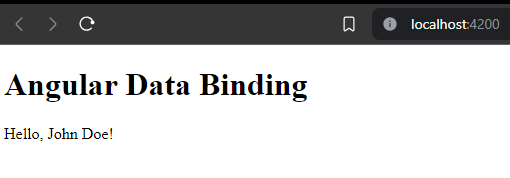
Ouptut
Approach 2: Property Binding
You can also use property binding to display variables in the HTML template. This approach is useful when you want to bind the variable to an element property, such as src for an image or value for an input field.
Syntax:
<img [src]="imageUrl" />
<input [value]="inputValue" />
Code Example:
<!-- app.component.html -->
<h1>{{ title }}</h1>
<p>Hello, {{ name }}!</p>
<img [src]="imageUrl" alt="Example Image">
// app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'Angular Data Binding';
name = 'John Doe';
imageUrl = 'https://example.com/image.jpg';
}
Output:
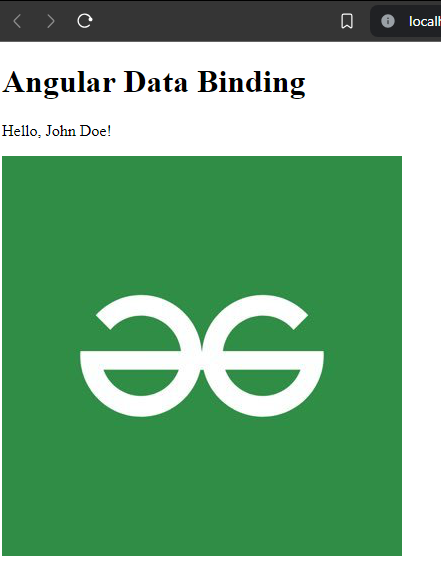
Output